Inside this article we will see how Form data submit using XMLHttpRequest in javascript. Article contains a classified information about sending form data to server using Post request type.
What is FormData in Javascript?
The FormData interface provides a way to easily construct a set of key/value pairs representing form fields and their values, which can then be easily sent using the XMLHttpRequest.
In javascript we use XMLHttpRequest for ajax requests. Additionally if you don’t have any idea about how to use XMLHttpRequest then also you will get some idea from it.
We will consider a HTML form with few input elements. Submit this form using XMLHttpRequest in javascript using formdata concept.
The keystone of AJAX is the XMLHttpRequest object.
- Create an XMLHttpRequest object
- Define a callback function
- Open the XMLHttpRequest object
- Send a Request to a server
Learn More –
- Encrypt Form Data in JavaScript Before Sending to Server
- Get Days Difference Between Two Dates In Javascript
- Get Element X and Y Position on Screen Using jQuery
- Get Latitude and Longitude of Location Using jQuery
Let’s get started.
Create an Application
Create a folder with name js-ajax in your localhost directory. Create files index.html and server.php into it.
index.html will contain HTML form and also the code which submits form data to server. server.php file process form data and returns a response to client.
Open index.html and write this complete code into it.
<!DOCTYPE html> <html lang="en"> <head> <title>Form Data Submit Using XMLHttpRequest in Javascript</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> </head> <body> <div class="container"> <h4>Form Data Submit Using XMLHttpRequest in Javascript</h4> <div class="panel panel-primary"> <div class="panel-heading">Form Data Submit Using XMLHttpRequest in Javascript</div> <div class="panel-body"> <form class="form-horizontal" action="javascript:void(0);" id="frmdata" onsubmit="submitFormData(this)"> <div class="form-group"> <label class="control-label col-sm-2" for="name">Name:</label> <div class="col-sm-10"> <input type="text" class="form-control" id="name" name="name" placeholder="Enter name"> </div> </div> <div class="form-group"> <label class="control-label col-sm-2" for="email">Email:</label> <div class="col-sm-10"> <input type="email" class="form-control" id="email" name="email" placeholder="Enter email"> </div> </div> <div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <button type="submit" class="btn btn-success">Submit</button> </div> </div> </form> </div> </div> </div> <script> // handle form submit function submitFormData(event) { // form values var name = event.name.value; var email = event.email.value; var data = new FormData(); data.append('name', name); data.append('email', email); var http = new XMLHttpRequest(); var url = 'server.php'; http.open('POST', url, true); http.onreadystatechange = function() { if (http.readyState == 4 && http.status == 200) { alert("Form submitted successfully"); document.getElementById("frmdata").reset(); console.log(http.responseText); } } http.send(data); } </script> </body> </html>
Here,
When we click on submit button, it runs submitFormData() function.
Submit handler function receiving form values by this code –
var name = event.name.value;
var email = event.email.value;
var data = new FormData();
data.append('name', name);
data.append('email', email);
Create an instance of XMLHttpRequest –
var http = new XMLHttpRequest();
Prepare form data which will be send to server –
http.send(data);
Open connection to send data via POST method –
http.open('POST', url, true);
On state change of instance, we will get server response –
http.onreadystatechange = function() {
if (http.readyState == 4 && http.status == 200) {
alert("Form submitted successfully");
document.getElementById("frmdata").reset();
console.log(http.responseText);
}
}
Open server.php and write this code into it.
<?php if ($_SERVER['REQUEST_METHOD'] === 'POST') { echo json_encode( array( "status" => 1, "message" => "Form submitted", "data" => $_POST ) ); } exit;
This is checking for submission via POST request type and simply returning the submitted form data. You can add any other code here for data like save in database, etc.
Application Testing
Open browser and type this –
URL – http://localhost/js-ajax/index.html
It will show a simple form with Name & Email as two input fields. When you submit, after getting server response it will show you a alert.
Here , is the image of HTML form and request processing via server.php
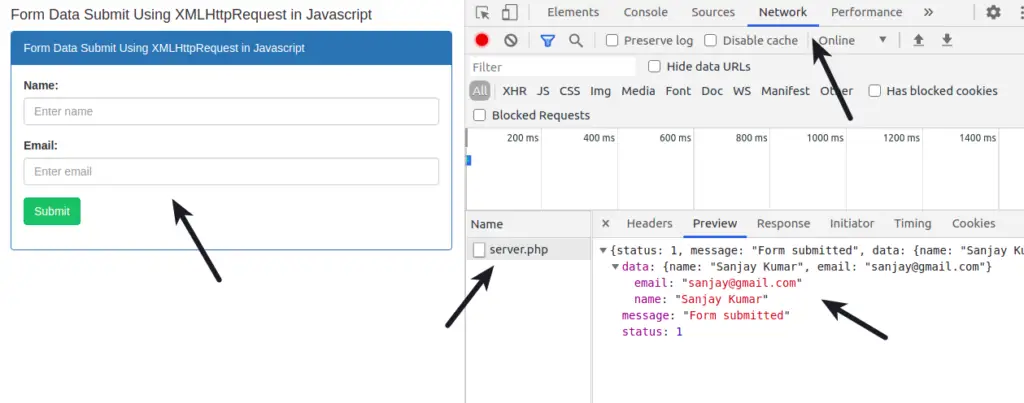
We hope this article helped you to learn Form Data Submit Using XMLHttpRequest in Javascript Tutorial Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.