If we work in any type of web applications in any language then at somewhere we need the help of google location api to get latitude and longitude. As google provides huge amount of services, so we are going to use one of it’s services for this.
Inside this article we will see concept to get latitude and longitude of location using jquery an google map library. But while working with this, we need a google map api key.
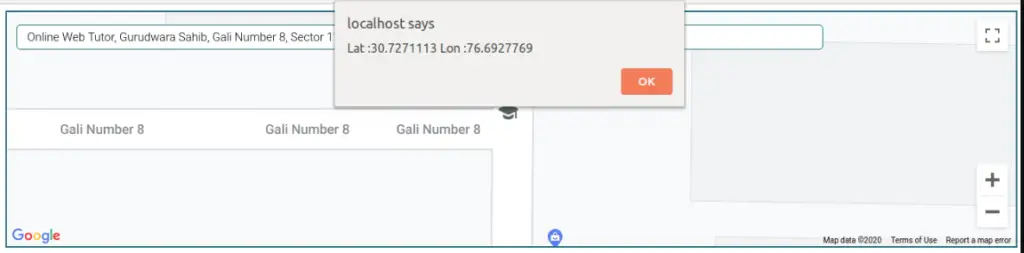
Let’s get started.
Project Setup – index.html
Create a project at your system. Inside that project folder create a file with any name let’s say index.html.
Copy and paste the below code into it.
<!DOCTYPE html> <html> <head> <title>Get Latitude and Longitude of Location Using jQuery - Online Web Tutor</title> <link href="map.css" rel="stylesheet"/> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.2.6/jquery.js"></script> <script src="https://maps.googleapis.com/maps/api/js?key=API_KEY&libraries=places"></script> <script src="map.js"></script> </head> <body> <div id="map" class=""> <input id="pac-input" class="controls" placeholder="Find your location" ame="location" type="text"> <div id="map-canvas"> <</div> <input name="lat" class="lat" type="hidden"> <input name="lon" class="lon" type="hidden"> </div> </body> </html>
Inside above code you need to replace API KEY with your google map api key only. Next inside this code, we have linked two files more named as map.css and map.js
Let’s set those files.
Project CSS & Js File
Create two more files in the above folder along with index.html called map.css and map.js file.
Let’s open up map.css and paste this given code.
#map { height: 300px; width: 100%; margin: auto auto; border: solid 2px #0d6b7a; -webkit-transform: translateZ(0); z-index: 10; } #map-canvas { height: 100%; width: 100%; margin: 0px; padding: 0px; z-index: 10; } .controls { margin-top: 16px; border: 1px solid #0d6b7a; box-sizing: border-box; -moz-box-sizing: border-box; height: 32px; outline: none; background-color: floralwhite; border-radius: 5px; } #pac-input { background-color: #fff; font-family: Roboto; font-size: 15px; font-weight: 300; margin-left: 12px; padding: 0 11px 0 13px; text-overflow: ellipsis; width: 80%; z-index: 1081; } #pac-input:focus { border-color: #0088FF; } .pac-container { font-family: Roboto; } #type-selector { color: #fff; background-color: #4d90fe; padding: 5px 11px 0px 11px; } #type-selector label { font-family: Roboto; font-size: 13px; font-weight: 300; } .pac-container { background-color: #FFF; z-index: 20; position: fixed; display: inline-block; float: left; }
Open up map.js file, copy and paste the given code.
var geocoder; var map; var infowindow = new google.maps.InfoWindow(); var marker; var g_err = 0; function initialize() { var markers = []; var mapOptions = { zoom: 7, center: new google.maps.LatLng(42.72, 12.00), mapTypeId: google.maps.MapTypeId.ROADMAP, mapTypeControl: false, streetViewControl: false }; map = new google.maps.Map(document.getElementById('map-canvas'), mapOptions); // Create the search box and link it to the UI element. var input = document.getElementById('pac-input'); map.controls[google.maps.ControlPosition.TOP_LEFT].push(input); var searchBox = new google.maps.places.SearchBox(input); // [START region_getplaces] // Listen for the event fired when the user selects an item from the // pick list. Retrieve the matching places for that item. google.maps.event.addListener(searchBox, 'places_changed', function() { var places = searchBox.getPlaces(); if (places.length == 0) { return; } for (var i = 0, marker; marker = markers[i]; i++) { marker.setMap(null); } // For each place, get the icon, place name, and location. markers = []; var bounds = new google.maps.LatLngBounds(); for (var i = 0, place; place = places[i]; i++) { var image = { url: place.icon, size: new google.maps.Size(75, 75), origin: new google.maps.Point(0, 0), anchor: new google.maps.Point(17, 34), scaledSize: new google.maps.Size(25, 25) }; // Create a marker for each place. var marker = new google.maps.Marker({ map: map, icon: image, title: place.name, position: place.geometry.location }); $('.lat').val(marker.position.lat()); $('.lon').val(marker.position.lng()); alert('Latitude :' + marker.position.lat() + ' Longitude :' + marker.position.lng()); markers.push(marker); bounds.extend(place.geometry.location); } map.fitBounds(bounds); }); // [END region_getplaces] // Bias the SearchBox results towards places that are within the bounds of the // current map's viewport. google.maps.event.addListener(map, 'bounds_changed', function() { var bounds = map.getBounds(); searchBox.setBounds(bounds); }); } google.maps.event.addDomListener(window, 'load', initialize);
That’s it.
We hope this article helped you to learn about to Get Latitude and Longitude of Location Using jQuery in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.