Laravel 8 is an open source PHP Framework. Inside this article we will see about implementation of Laravel 4 CRUD Application Tutorial. Nowadays, every application have a common operation CRUD. CRUD stands for Create, Read, Update & Delete.
We will see, about each operation of CRUD in Laravel 8 in a very clear way. We will interact with application database, model etc.
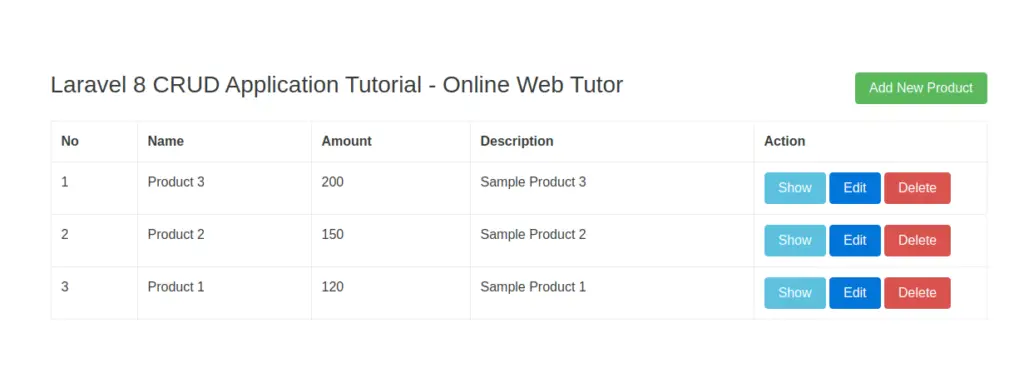
Learn More –
- Concept of Route Model Binding in Laravel 8 with Example
- Concept of Trait in Laravel 8 Tutorial with Example
- Create Custom 404 Page in Laravel 8 | Page Not Found
- Create Custom Facade Class in Laravel 8 Tutorial
Let’s get started CRUD Example in Laravel 8.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Migration
As we are going to create CRUD (Create, Read, Update, Delete) application in laravel. So let’s take an example as product.
Here we have to create a migration which creates a products table.
$ php artisan make:migration create_products_table --create=products
After running this command at your terminal, you will see one file will be created at this following path /database/migrations.
Open {timestamp}_create_products_table.php file and write this complete code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateProductsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->id(); $table->string('name'); $table->integer('amount'); $table->text('description'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } }
Now, you have to run this given command to migrate the above file.
$ php artisan migrate
Above command will creates a products table in database.
Add Application Controller and Model
Next, we should create a new controller as ProductController. We are going to create a resource controller by running the given command.
$ php artisan make:controller ProductController --resource --model=Product
This command will creates a ProductController i.e a resource controller and a model. You can find the created files at this path app/Http/Controllers/ProductController.php & model at app/Models/Product.php
In this resource controller we will have these following methods:
- index()
- create()
- store()
- show()
- edit()
- update()
- delete()
Open up the model from this path app/Models/Product.php and write this updated code.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; protected $fillable = [ 'name', 'description', 'amount' ]; }
Open up app/Http/Controllers/ProductController.php and paste this updated code.
<?php namespace App\Http\Controllers; use App\Models\Product; use Illuminate\Http\Request; class ProductController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $products = Product::latest()->paginate(5); return view('products.index',compact('products')) ->with('i', (request()->input('page', 1) - 1) * 5); } /** * Show the form for creating a new resource. * * @return \Illuminate\Http\Response */ public function create() { return view('products.create'); } /** * Store a newly created resource in storage. * * @param \Illuminate\Http\Request $request * @return \Illuminate\Http\Response */ public function store(Request $request) { $request->validate([ 'name' => 'required', 'description' => 'required', 'amount' => 'required', ]); Product::create($request->all()); return redirect()->route('products.index') ->with('success','Product created successfully.'); } /** * Display the specified resource. * * @param \App\Product $product * @return \Illuminate\Http\Response */ public function show(Product $product) { return view('products.show',compact('product')); } /** * Show the form for editing the specified resource. * * @param \App\Product $product * @return \Illuminate\Http\Response */ public function edit(Product $product) { return view('products.edit',compact('product')); } /** * Update the specified resource in storage. * * @param \Illuminate\Http\Request $request * @param \App\Product $product * @return \Illuminate\Http\Response */ public function update(Request $request, Product $product) { $request->validate([ 'name' => 'required', 'description' => 'required', 'amount' => 'required', ]); $product->update($request->all()); return redirect()->route('products.index') ->with('success','Product updated successfully'); } /** * Remove the specified resource from storage. * * @param \App\Product $product * @return \Illuminate\Http\Response */ public function destroy(Product $product) { $product->delete(); return redirect()->route('products.index') ->with('success','Product deleted successfully'); } }
Application Resource Route Settings
Open up route configuration file web.php from path routes/web.php.
Add the following code into it.
use App\Http\Controllers\ProductController; Route::resource('products', ProductController::class);
If we are interested to see the routes associated with Resource Controller, back to terminal and type command $ php artisan route:list
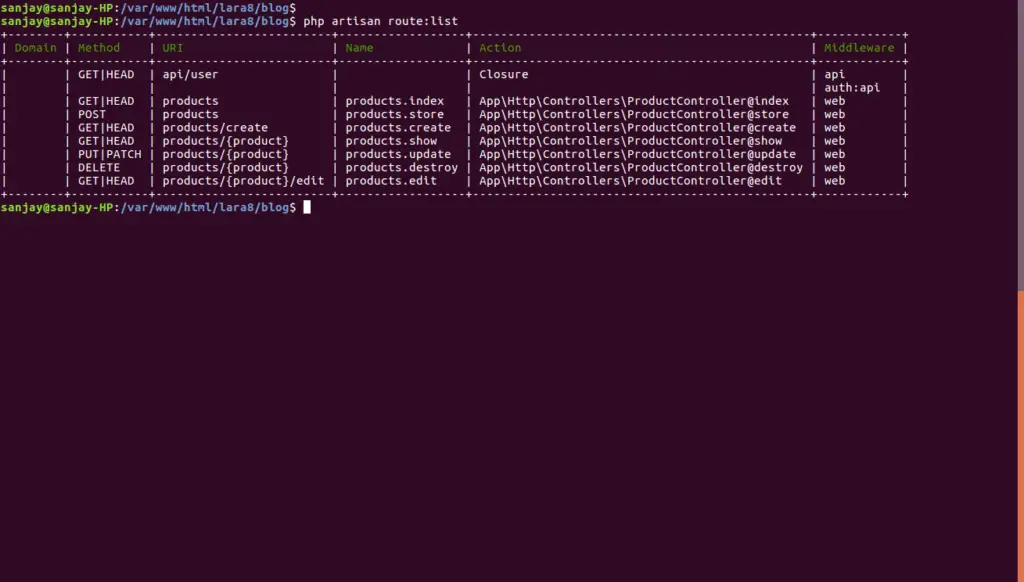
Adding Application Layout Files
Inside this we need to create layout files of product. Let’s create a new folder products at path resources/views/ then create blade files of CRUD application into it.
So we have to create the following bellow blade files:
- layout.blade.php
- index.blade.php
- create.blade.php
- edit.blade.php
- show.blade.php
Open up the file resources/views/products/layout.blade.php and paste the following given code into it.
<!DOCTYPE html> <html> <head> <title>Laravel 8 CRUD Application Tutorial - Online Web Tutor</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha/css/bootstrap.css" rel="stylesheet"> </head> <body> <div class="container" style="margin-top:100px;"> @yield('content') </div> </body> </html>
Open file resources/views/products/index.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h3>Laravel 8 CRUD Application Tutorial - Online Web Tutor</h3> </div> <div class="pull-right"> <a class="btn btn-success" href="{{ route('products.create') }}"> Add New Product</a> </div> </div> </div> @if ($message = Session::get('success')) <div class="alert alert-success"> <p>{{ $message }}</p> </div> @endif <table class="table table-bordered" style="margin-top:20px;"> <tr> <th>No</th> <th>Name</th> <th>Amount</th> <th>Description</th> <th width="280px">Action</th> </tr> @foreach ($products as $product) <tr> <td>{{ ++$i }}</td> <td>{{ $product->name }}</td> <td>{{ $product->amount }}</td> <td>{{ $product->description }}</td> <td> <form action="{{ route('products.destroy',$product->id) }}" method="POST"> <a class="btn btn-info" href="{{ route('products.show',$product->id) }}">Show</a> <a class="btn btn-primary" href="{{ route('products.edit',$product->id) }}">Edit</a> @csrf @method('DELETE') <button type="submit" class="btn btn-danger">Delete</button> </form> </td> </tr> @endforeach </table> {!! $products->links() !!} @endsection
Open up the file resources/views/products/create.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2>Add New Product</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a> </div> </div> </div> @if ($errors->any()) <div class="alert alert-danger"> <strong>Whoops!</strong> There are some issues.<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form action="{{ route('products.store') }}" method="POST"> @csrf <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Name:</strong> <input type="text" name="name" class="form-control" placeholder="Name"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Amount:</strong> <input type="number" name="amount" class="form-control" placeholder="Amount"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Description:</strong> <textarea class="form-control" style="height:150px" name="description" placeholder="Description"></textarea> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12 text-center"> <button type="submit" class="btn btn-primary">Submit</button> </div> </div> </form> @endsection
Open up the file resources/views/products/edit.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2>Edit Product</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a> </div> </div> </div> @if ($errors->any()) <div class="alert alert-danger"> <strong>Whoops!</strong> There are some issues.<br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form action="{{ route('products.update',$product->id) }}" method="POST"> @csrf @method('PUT') <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Name:</strong> <input type="text" name="name" value="{{ $product->name }}" class="form-control" placeholder="Name"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Amount:</strong> <input type="number" name="amount" value="{{ $product->amount }}" class="form-control" placeholder="Amount"> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Detail:</strong> <textarea class="form-control" style="height:150px" name="description" placeholder="Detail">{{ $product->description }}</textarea> </div> </div> <div class="col-xs-12 col-sm-12 col-md-12 text-center"> <button type="submit" class="btn btn-primary">Submit</button> </div> </div> </form> @endsection
Open up the file resources/views/products/show.blade.php
@extends('products.layout') @section('content') <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2> Show Product</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('products.index') }}"> Back</a> </div> </div> </div> <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Name:</strong> {{ $product->name }} </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Amount:</strong> {{ $product->amount }} </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Description:</strong> {{ $product->description }} </div> </div> </div> @endsection
Finally, we are ready to run Laravel 8 CRUD application.
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Open up the URL: http://127.0.0.1:8000/products
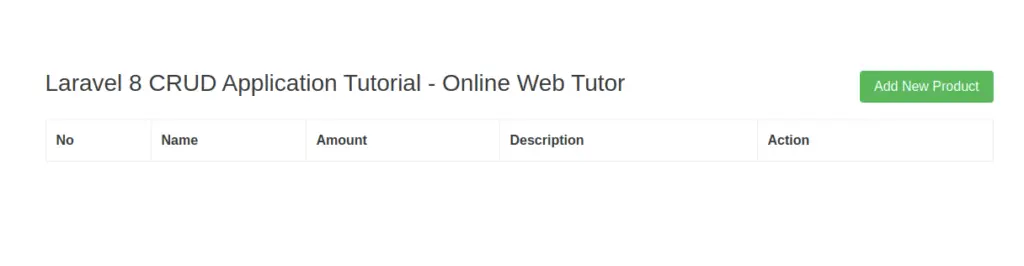
When we click on Add New Product button it will open http://127.0.0.1:8000/products/create
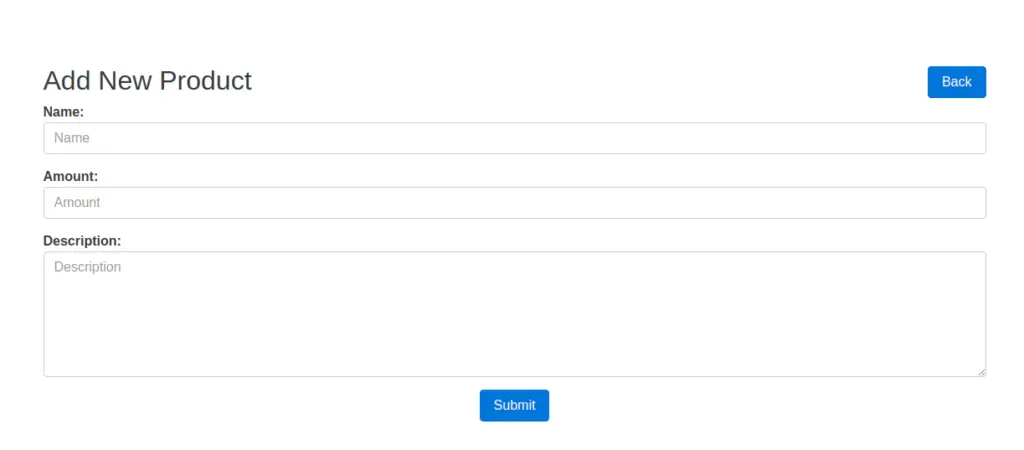
When we add products from Create Form, we will see products list at /products URL. In the list we will see the Edit & Delete button.
We hope this article helped you to learn about Laravel 8 CRUD Application Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.
Hello, I would like to subscribe for this blog to take hottest updates, thus where can i do it please help out.
Hi, you will see a bell icon at left bottom corner. Click on that to get every updates.
Fantastic goods from you, man. I have take into account your stuff
prior to and you are just extremely great.
I really like what you’ve obtained right here, certainly like what you are stating and the way through which you are saying it.
You’re making it entertaining and you continue to care for to stay it smart.
I cant wait to learn far more from you. This is really a great site.
Howdy! Would you mind if I share your blog with my twitter group?
There’s a lot of folks that I think would really appreciate your content.
Please let me know. Thank you
Sure, you can share. Thanks
Great article, totally what I wanted to find.
Your method of explaining the whole thing in this post is really fastidious, all be capable of easily understand it, Thanks a lot.
Thanks for finally talking about >Complete Laravel 8 CRUD
Application Tutorial <Loved it!
I visit everyday some sites and sites to read articles, except
this blog gives feature based content.
Excellent article. I’m experiencing many of these issues as well..
This blog was… how do you say it? Relevant!!
Finally I have found something that helped me. Cheers!
Greetings! Very helpful advice within this article!
It’s the little changes which will make the greatest changes.
Thanks a lot for sharing!
Hi there friends, nice post and good arguments commented at this place,
I am truly enjoying by these.
InvalidArgumentException
View [products.index] not found.
http://127.0.0.1:8000/products
Hide solutions
products.index was not found.
Are you sure the view exists and is a .blade.php file?
Hi, yes you can see we have a view file for index i.e resources/views/products/index.blade.php