Inside this article we will see the concept of Add Image into PDF laravel 8. This will be very interesting topic to see. We will convert a HTML view template into PDF document and attach a image file into it.
By the help of this article, we can easily add a logo into PDF files.
DomPDF is a package we install via composer which helps to work with PDF. How to generate PDF in laravel 8 Using DOMPdf, Click here.
This tutorial is not laravel 8 specific, we can use the same concept into other versions of laravel as well.
Let’s get started.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Install dompdf Package
Here, we will install a pdf package to laravel application. Open project in terminal and type this composer command to install.
$ composer require barryvdh/laravel-dompdf
When we install we should see the given image in terminal
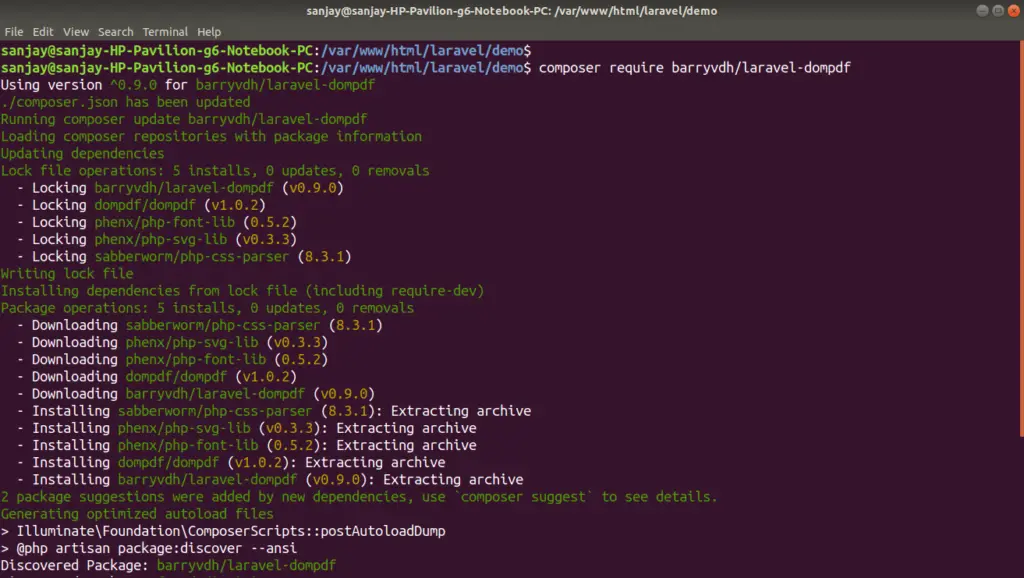
Package Configuration in Application
Successfully, we have installed above. Next, we need to set some application configuration.
Open app.php from /config/app.php
Search for the array of Providers and aliases. Add the given code into providers and aliases array.
//.. Other Codes 'providers' => [ .... Barryvdh\DomPDF\ServiceProvider::class, ], 'aliases' => [ .... 'PDF' => Barryvdh\DomPDF\Facade::class, ]
Add Route
To add application route, open up the file web.php from /routes.
Open web.php and add this following code.
# Import Controller Class use App\Http\Controllers\PDFController; # Add Route Route::get('generate-pdf', [PDFController::class, 'generatePDF']);
Create Controller
Open laravel application into terminal and run this following command. This command will create a controller class file at location /app/Http/Controllers
$ php artisan make:controlle
r PDFController
Open controller file and write the given code into that.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use PDF; class PDFController extends Controller { public function generatePDF() { $data = [ 'title' => 'Welcome to OnlineWebTutorBlog.com', 'author' => "Sanjay" ]; $pdf = PDF::loadView('my-pdf-file', $data); return $pdf->download('onlinewebtutorblog.pdf'); } }
Create PDF Template File
Create a file named as my-pdf-file.blade.php at /resources/views folder.
This template file will be the layout for pdf file.
First let’s place logo file into /public folder which is at application root. This logo file we will add into pdf.
Placing a image file with name “image.png” inside /public folder.
Open file my-pdf-file.blade.php
<!DOCTYPE html> <html> <head> <title>Title From OnlineWebTutorBlog</title> </head> <body> <div style="text-align: center;"> <img src="{{ public_path('image.png') }}" style="width: 100px; height: 100px"> </div> <h1>Title: {{ $title }}</h1> <h3>Author: {{ $author }}</h3> <p>ut aliquip ex ea commodoconsequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidata.</p> </body> </html>
- public_path(‘image.png’) Returns the path of image.png from /public folder.
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Open up the URL – http://localhost:8000/generate-pdf
When we type this URL, it will download a pdf file into system.
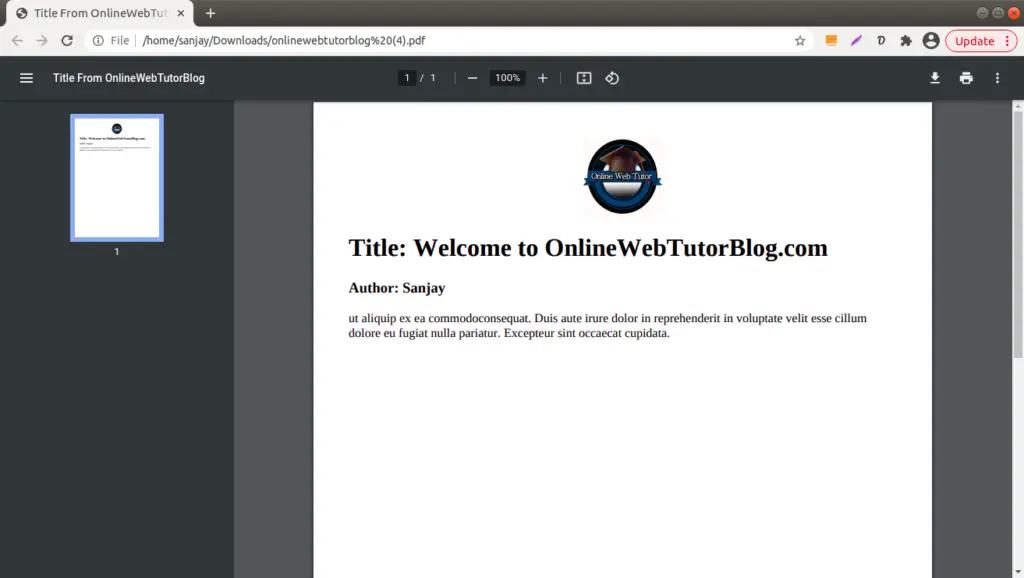
We hope this article helped you to learn about How To Add Image Into PDF Laravel 8 Tutorial Example in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.