Inside this article we will see the complete concept to get current location details in laravel 8. It will be super easy to understand and very few simple steps to integrate into laravel application.
We will use a composer package which helps us to get the all the details country, zip code, latitude, longitude etc.
This article is not laravel 8 specific even you are free to use in any of your version.
Let’s get started.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Install Composer Package
Open project into terminal and run this composer command to install this Location package.
$ composer require stevebauman/Location
It will install all needed package files into /vendor folder.
Configure Package in Application
Open app.php file from /config folder.
Search for providers, add this line to into array
//... Stevebauman\Location\LocationServiceProvider::class,
Search for aliases, add this line to into array
//... 'Location' => 'Stevebauman\Location\Facades\Location',
Publish Configuration Files
Open project in terminal and run this command to publish the files.
$ php artisan vendor:publish --provider="Stevebauman\Location\LocationServiceProvider"
Create Controller
Go to terminal and run this artisan command to create controller file.
$ php artisan make:controller LocationController
It will create LocationController.php at /app/Http/Controllers folder.
Open file LocationController.php and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Location; class LocationController extends Controller { public function ip_details() { // Put your IP address $ip = '203.115.91.17'; $data = Location::get($ip); return view('details', compact('data')); } }
Create Blade Layout File
Go to /resources/views folder and create a file details.blade.php.
Open details.blade.php and write this complete into it,
<html> <head> <title>Current Location Details in Laravel 8</title> <style> .box { margin: auto; width: 50%; border: 3px dashed green; padding: 10px; text-align: center; } </style> </head> <body style="text-align: center;"> <h1>Current Location Details in Laravel 8</h1> <div class="box"> <h3>IP: {{ $data->ip }}</h3> <h3>Country Name: {{ $data->countryName }}</h3> <h3>Country Code: {{ $data->countryCode }}</h3> <h3>Region Code: {{ $data->regionCode }}</h3> <h3>Region Name: {{ $data->regionName }}</h3> <h3>City Name: {{ $data->cityName }}</h3> <h3>Zipcode: {{ $data->zipCode }}</h3> <h3>Latitude: {{ $data->latitude }}</h3> <h3>Longitude: {{ $data->longitude }}</h3> </div> </body> </html>
Add Route
Open web.php file from /routes folder. We need to add a route into it.
# Add this to header use App\Http\Controllers\LocationController; //... Route::get('ip-details', [LocationController::class, 'ip_details'])->name('ip_details');
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/ip-details
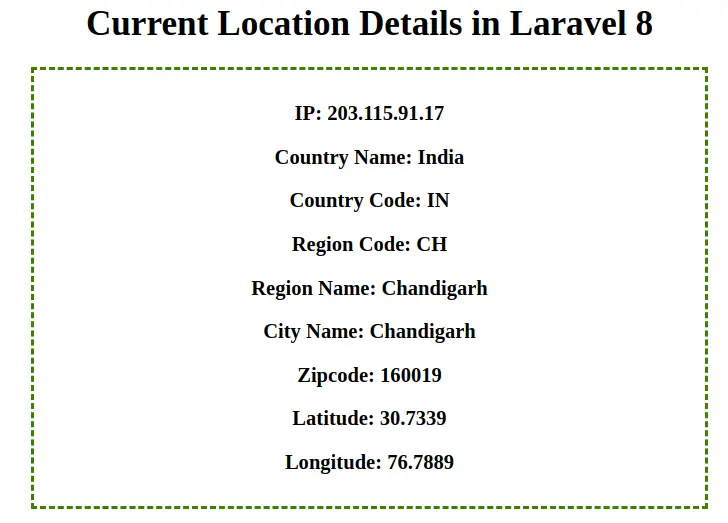
We hope this article helped you to learn about How To Get Current Location Details in Laravel 8 Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.