Welcome to our complete tutorial on how to use two powerful methods in Laravel 10 collections, count() and countBy(). You understand the significance of efficient data handling and analysis as a Laravel developer.
In this tutorial, we will go into the world of Laravel collections and look at how the count() and countBy() functions work. You will learn Step-by-step guide to Use Laravel Collection count() and countBy() Methods.
The Illuminate\Support\Collection
class provides a fluent, convenient wrapper for working with arrays of data. For example, check out the following code. We’ll use the collect
helper to create a new collection instance from the array.
Read More: Learn Laravel 10 Collection filter() Tutorial for Beginners
As mentioned above, the collect
helper returns a new Illuminate\Support\Collection
instance for the given array.
So, creating a collection is as simple as:
$collection = collect([1, 2, 3]);
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Example #1 Use count() Method
In this example we will consider an array. We will see how to use count() into collection.
Read More: How To Get Unique values in Laravel 10 Collection Tutorial
Suppose we have SiteController.php a controller file inside /app/Http/Controllers folder.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class SiteController extends Controller { public function index() { $data = collect([1, 2, 3, 4, 2, 3, 1, 5, 4, 7, 6, 8, 9, 3, 10, 4, 5]); $total = $data->count(); echo "Total Collection Items: " . $total; } }
Concept
$total = $data->count();
Output
Total Collection Items: 17
Example #2 Use countBy() Method
We will see how to use countBy() into collection.
Suppose we have SiteController.php a controller file inside /app/Http/Controllers folder.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class SiteController extends Controller { public function index() { $data = collect([1, 2, 3, 4, 2, 3, 1, 5, 4, 7, 6, 8, 9, 3, 10, 4, 5]); $elements = $data->countBy(); dd($elements); } }
It will count total occurrence in collection item wise.
Read More: Step-by-Step Guide To Merge Eloquents in Laravel 10 Collection
Concept
$elements = $data->countBy();
Output
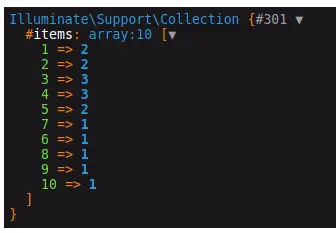
We hope this article helped you to learn How To Use Laravel 10 Collection count() and countBy() Methods Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.