Image upload is a common feature in web applications, and it is critical for data integrity and security that the submitted images match specified criteria. Image upload validation in Laravel 10 is an efficient approach to validate and regulate the kind, size, and quality of submitted images.
We will walk you through the process of verifying image uploads in Laravel 10 in this article, providing a detailed guide with real examples.
We’ll go over image validation basics including file type, size, and dimension checks, as well as real-world examples of how to apply image upload validation in your web applications.
Read More: Laravel 10 Convert Word Docx File to PDF File Example
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Laravel 10 Rules to Validate an Image
Laravel 10 provides a list of default validation rules when image uploads only we need to use that,
- image
- mimes
- dimensions
- max
Using above validation rules you can set to upload function like file type as “jpg,png,jpeg,gif,svg”, Using max you can set limit of file size and Using dimensions you can set file height and width limit.
Setup Image Upload Controller
Back to project terminal and run this command into it,
php artisan make:controller ImageController
It will create a ImageController.php file inside /app/Http/Controllers folder.
Read More: Laravel 10 Model Create Events Example Tutorial
Open file and write this code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\View\View; use Illuminate\Http\RedirectResponse; class ImageController extends Controller { public function index(): View { return view('image-upload'); } public function store(Request $request): RedirectResponse { $this->validate($request, [ 'image' => ['required', 'image', 'mimes:jpg,png,jpeg,gif,svg', 'dimensions:min_width=100,min_height=100,max_width=1000,max_height=1000', 'max:2048'], ]); $imageName = time().'.'.$request->image->extension(); $request->image->move(public_path('images'), $imageName); /* You model queries Save $imageName name in DB from here. */ return back() ->with("success", "You have successfully uploaded an image.") ->with("image", $imageName); } }
Create Blade Template
Create a file named as image-upload.blade.php inside /resources/views folder.
Open file and write this code into it,
<!DOCTYPE html> <html lang="en"> <head> <title>How To Validate Image Upload in Laravel 10 Example</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <h3>How To Validate Image Upload in Laravel 10 Example</h3> <div class="panel panel-primary"> <div class="panel-heading">Image Upload Validation</div> <div class="panel-body"> @if ($message = Session::get('success')) <div class="alert alert-success"> <strong>{{ $message }}</strong> </div> <img src="images/{{ Session::get('image') }}"> @endif <form action="{{ route('image.store') }}" method="post" enctype="multipart/form-data"> @csrf <div class="form-group"> <label for="file">Image:</label> <input type="file" name="image" id="inputImage" class="form-control @error('image') is-invalid @enderror"> @error('image') <span class="text-danger">{{ $message }}</span> @enderror </div> <button type="submit" class="btn btn-success">Upload</button> </form> </div> </div> </div> </body> </html>
Add Route
Open web.php from /routes folder. Add these routes into it,
//... use App\Http\Controllers\ImageController; Route::controller(ImageController::class)->group(function(){ Route::get('image-upload', 'index'); Route::post('image-upload', 'store')->name('image.store'); }); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Read More: Laravel 10 How to Add Qr Code in PDF Using DomPDF
URL: http://127.0.0.1:8000/image-upload
When you upload an image with other extensions like .pdf, .mp4, etc.
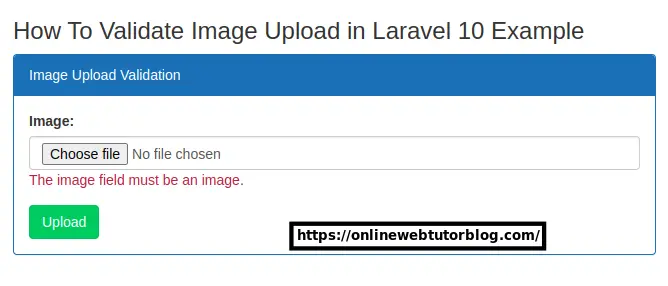
When you upload file of invalid dimensions,
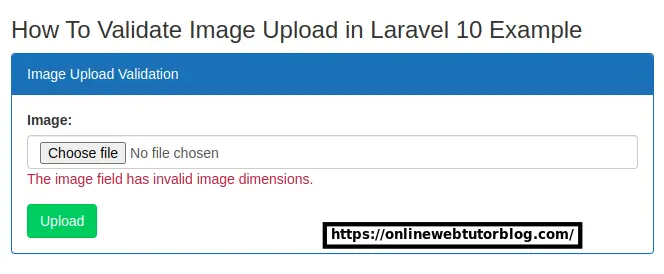
That’s it.
We hope this article helped you to learn about How To Validate Image Upload in Laravel 10 Example in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.