Inside this article we will see the concept i.e Laravel 9 Ajax DELETE request example tutorial. Article contains the classified information about working with Ajax DELETE request in laravel 9.
While working with forms we have these request methods – POST, GET, DELETE etc. Article contains the information about DELETE request in Ajax request. We will consider a list of users. To delete a specific user we will fire an ajax request using DELETE request type.
Learn More –
- Laravel 9 Cookies – Get, Set, Delete Cookie Example Tutorial
- Laravel 9 Livewire Multi Step Form Wizard Tutorial
- Laravel 9 How to Get Current Route Name Tutorial
- Laravel 9 How to Get Request Parameter Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Generate Fake Data Using Factory
As you install laravel 9 setup, you should see these files –
- User.php a model file inside /app/Models folder.
- UserFactory.php a factory file inside /database/factories folder.
We will use concept of factory to seed dummy data to this application. But first you need to migrate your application and create users table into your database.
$ php artisan tinker
It will open tinker shell to execute commands. Copy and paste this command and execute to create test data.
>>> User::factory()->count(20)->create()
It will generate 20 fake rows of users into table.
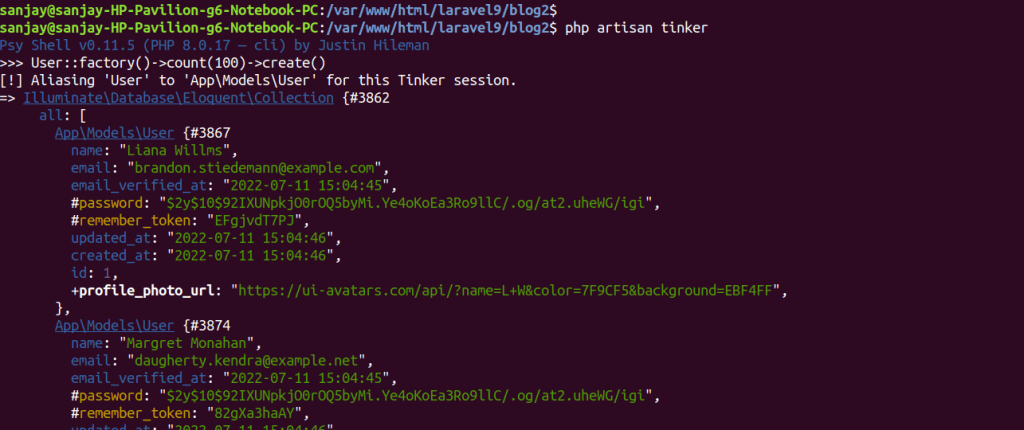
Create Controller
Next,
We need to create a controller file.
$ php artisan make:controller UserController
It will create a file UserController.php inside /app/Http/Controllers folder.
Open UserController.php and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $users = User::paginate(10); return view('users', compact('users')); } /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function delete($id) { User::find($id)->delete(); return response()->json(['success' => 'User Deleted Successfully!']); } }
Create Blade Layout File
Go to /resources/views folder and create a file with name users.blade.php
Open users.blade.php and write this complete code into it.
<!DOCTYPE html> <html> <head> <title>Laravel 9 Ajax DELETE Request Example Tutorial</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.0.1/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"></script> <meta name="csrf-token" content="{{ csrf_token() }}"> </head> <body> <div class="container" style="margin-top: 10px;"> <h3 style="text-align: center;">Laravel 9 Ajax DELETE Request Example Tutorial</h3> <table class="table table-bordered data-table" style="margin-top: 30px;"> <thead> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Action</th> </tr> </thead> <tbody> @foreach ($users as $user) <tr> <td>{{ $user->id }}</td> <td>{{ $user->name }}</td> <td>{{ $user->email }}</td> <td> <a href="javascript:void(0)" data-url="{{ route('users.delete', $user->id) }}" class="btn btn-danger delete-user">Delete</a> </td> </tr> @endforeach </tbody> </table> </div> </body> <script type="text/javascript"> $(document).ready(function() { $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); /*------------------------------------------ -------------------------------------------- When click user on Show Button -------------------------------------------- --------------------------------------------*/ $(document).on('click', '.delete-user', function() { var userURL = $(this).data('url'); var trObj = $(this); if (confirm("Are you sure you want to delete this user?") == true) { $.ajax({ url: userURL, type: 'DELETE', dataType: 'json', success: function(data) { //alert(data.success); trObj.parents("tr").remove(); } }); } }); }); </script> </html>
Concept
To delete a single user row, fired an ajax request using DELETE request type.
$(document).on('click', '.delete-user', function() {
var userURL = $(this).data('url');
var trObj = $(this);
if (confirm("Are you sure you want to delete this user?") == true) {
$.ajax({
url: userURL,
type: 'DELETE',
dataType: 'json',
success: function(data) {
//alert(data.success);
trObj.parents("tr").remove();
}
});
}
});
Add Route
Open web.php from /routes folder and add these route into it.
//... use App\Http\Controllers\UserController; //... Route::get('users', [UserController::class, 'index']); Route::delete('users/{id}', [UserController::class, 'delete'])->name('users.delete');
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/users
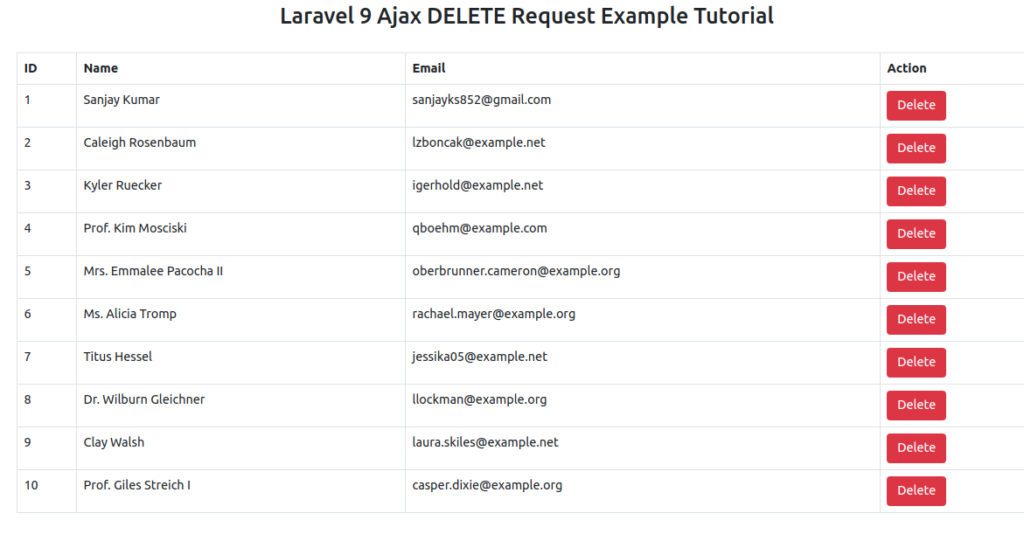
When you click on any row – Delete button. It will ask for confirmation, if press Ok and then delete from table.
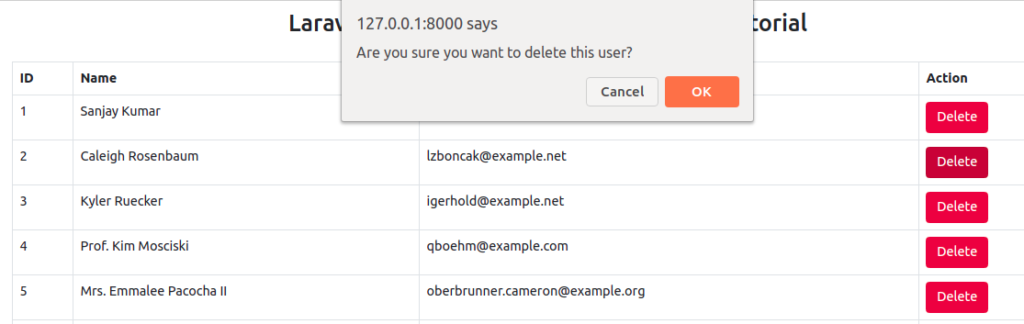
We hope this article helped you to Laravel 9 Ajax DELETE Request Example Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.