Inside this article we will see the concept of Laravel 9 Cookies – Get, Set, Delete Cookie Example. Article contains the classified information about laravel cookies. We will see about each concept like How to set cookie in laravel, how to get cookie in laravel, how to remove cookie in laravel, etc in a very clear way.
In laravel to work with cookies we have two options – Cookie Facade class and by Request Object.
HTTP cookies are small blocks of data created by a web server while a user is browsing a website and placed on the user’s computer or other device by the user’s web browser.
Learn More –
- Laravel 9 Work With Livewire Form Submit Tutorial
- Laravel 9 How To Save Array Data in Database Table
- Laravel 9 Livewire DataTable Pagination Using Package Tutorial
- Laravel 9 How To Work With Livewire Pagination Example
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Laravel Set Cookie
Here, we will see the ways to set cookie in laravel by two ways:
- Using Cookie Facade
- Using Request Object
Example 1: Cookie Set Using Cookie Facade
Here, we will use the concept of Cookie facade.
Syntax
Cookie::queue('name', 'value', $minutes);
Example Code
Suppose we have this controller, we can use the concept of cookie here as –
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Cookie; class CookieController extends Controller { /** * Write code on Method * * @return response() */ public function setCookie() { Cookie::queue('owt-cookie', 'Setting Cookie from Online Web Tutor', 120); return response()->json(['Cookie set successfully.']); } }
Example 2: Cookie Set with Request Response
Here, we will use the concept of Request object.
Syntax
cookie('name', 'value', $minutes);
Example Code
Suppose we have this controller, we can use the concept of cookie here as –
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class CookieController extends Controller { /** * Write code on Method * * @return response() */ public function setCookie() { return response()->json(['Cookie set successfully.'])->cookie( 'owt-cookie-2', 'This is a sample text inside cookie', 120 ); } }
When we execute above code we will cookie will be set inside browser cookies list.
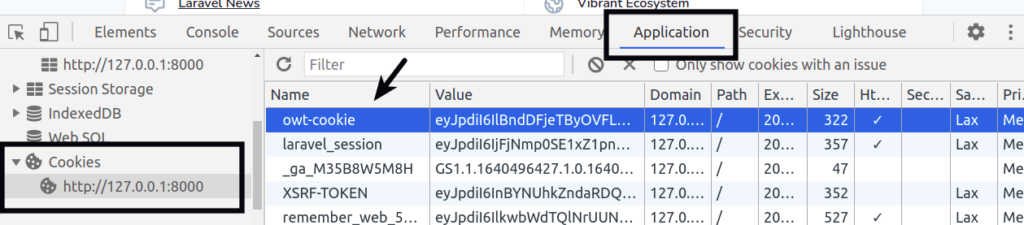
Laravel Get Cookies
Next,
We will see the available methods to work with laravel cookies to get values –
- Using Cookie Facade
- Using Request Object
Example 1: Cookie Get Using Cookie Facade
Syntax
Cookie::get('name')
Example Code
Suppose we have this controller, we can use the concept of cookie here as –
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Cookie; class CookieController extends Controller { /** * Write code on Method * * @return response() */ public function getCookie(Request $request) { $value = Cookie::get('owt-cookie'); dd($value); } }
Example 2: Cookie Get with Request Response
Here, we will use the concept of Request object.
Syntax
$request->cookie('name')
Example Code
Suppose we have this controller, we can use the concept of cookie here as –
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class CookieController extends Controller { /** * Write code on Method * * @return response() */ public function getCookie(Request $request) { $value2 = $request->cookie('owt-cookie-2'); dd($value2); } }
Laravel Delete Cookies
To delete a cookie from laravel, we have a very simple method of Cookie Facade.
Syntax
Cookie::forget('name')
Example Code
Suppose we have this controller, we can use the concept of cookie here as –
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Cookie; class CookieController extends Controller { /** * Write code on Method * * @return response() */ public function deleteCookie() { Cookie::forget('owt-cookie'); Cookie::forget('owt-cookie-2'); dd('Cookie removed successfully.'); } }
We hope this article helped you to learn Laravel 9 Cookies – Get, Set, Delete Cookie Example Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.