Inside this article we will see the concept i.e Laravel 9 livewire multi step form wizard with an example form. Article contains classified information about livewire multi step form wizard.
Multi step form means a form is built in different different steps. Here, we will see a form wizard built using the concept of laravel livewire. Article is not laravel version specific. You can use it with any version which supports livewire package.
If you are looking for an article which gives you the concept of livewire multi step form wizard then this article will help you a lot to understand the things.
Learn More –
- Laravel 9 Cookies – Get, Set, Delete Cookie Example Tutorial
- Laravel 9 How To Save Array Data in Database Table
- Laravel 9 Livewire DataTable Pagination Using Package Tutorial
- Laravel 9 How To Work With Livewire Pagination Example
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Migration
Open project into terminal and run this command to create migration file.
$ php artisan make:migration create_products_table
It will create a file –
- Migration file – 2022_07_15_144958_create_products_table.php at /database/migrations folder.
Open Migration file and write this complete code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->id(); $table->string('name')->nullable(); $table->longText('description')->nullable(); $table->float('amount')->nullable(); $table->boolean('status')->default(0); $table->integer('stock')->default(0); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } };
Run Migration
Next,
We need to create table inside database.
$ php artisan migrate
This command will create table – products inside database.
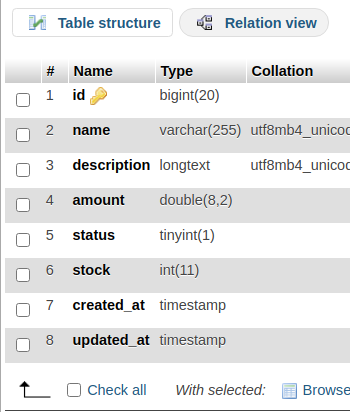
Create Model
Open project terminal and run this command to create model.
$ php artisan make:model Product
It will create Product.php file inside /app/Models folder. Open file and write this code into it.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'amount', 'description', 'status', 'stock' ]; }
Install Livewire – A Composer Package
Back to project terminal and run this command to install livewire inside this laravel application.
$ composer require livewire/livewire
It will install livewire composer package into application.
Generate Livewire Scaffolding
Again,
Back to terminal and run this command to create livewire folder & files.
$ php artisan make:livewire wizard
Above command will create these files –
- Wizard.php file inside /app/Http/Livewire folder.
- wizard.blade.php file inside /resources/views/livewire folder.
Next,
We will setup codes inside these files.
Livewire Form Wizard Settings
Open Wizard.php file from /app/Http/Livewire folder and write this following code into it.
<?php namespace App\Http\Livewire; use Livewire\Component; use App\Models\Product; class Wizard extends Component { public $currentStep = 1; public $name, $amount, $description, $status = 1, $stock; public $successMessage = ''; /** * Write code on Method * * @return response() */ public function render() { return view('livewire.wizard'); } /** * Write code on Method * * @return response() */ public function firstStepSubmit() { $validatedData = $this->validate([ 'name' => 'required|unique:products', 'amount' => 'required|numeric', 'description' => 'required', ]); $this->currentStep = 2; } /** * Write code on Method * * @return response() */ public function secondStepSubmit() { $validatedData = $this->validate([ 'stock' => 'required', 'status' => 'required', ]); $this->currentStep = 3; } /** * Write code on Method * * @return response() */ public function submitForm() { Product::create([ 'name' => $this->name, 'amount' => $this->amount, 'description' => $this->description, 'stock' => $this->stock, 'status' => $this->status, ]); $this->successMessage = 'Product created successfully.'; $this->clearForm(); $this->currentStep = 1; } /** * Write code on Method * * @return response() */ public function back($step) { $this->currentStep = $step; } /** * Write code on Method * * @return response() */ public function clearForm() { $this->name = ''; $this->amount = ''; $this->description = ''; $this->stock = ''; $this->status = 1; } }
Open wizard.blade.php blade template file from /resources/views/livewire folder and write this code into it.
<div> @if(!empty($successMessage)) <div class="alert alert-success"> {{ $successMessage }} </div> @endif <div class="stepwizard"> <div class="stepwizard-row setup-panel"> <div class="stepwizard-step"> <a href="#step-1" type="button" class="btn btn-circle {{ $currentStep != 1 ? 'btn-default' : 'btn-primary' }}">1</a> <p>Step 1</p> </div> <div class="stepwizard-step"> <a href="#step-2" type="button" class="btn btn-circle {{ $currentStep != 2 ? 'btn-default' : 'btn-primary' }}">2</a> <p>Step 2</p> </div> <div class="stepwizard-step"> <a href="#step-3" type="button" class="btn btn-circle {{ $currentStep != 3 ? 'btn-default' : 'btn-primary' }}" disabled="disabled">3</a> <p>Step 3</p> </div> </div> </div> <div class="row setup-content {{ $currentStep != 1 ? 'displayNone' : '' }}" id="step-1"> <div class="col-xs-12"> <div class="col-md-12"> <h3> Step 1</h3> <div class="form-group"> <label for="title">Product Name:</label> <input type="text" wire:model="name" class="form-control" id="taskTitle"> @error('name') <span class="error">{{ $message }}</span> @enderror </div> <div class="form-group"> <label for="description">Product Amount:</label> <input type="text" wire:model="amount" class="form-control" id="productAmount"/> @error('amount') <span class="error">{{ $message }}</span> @enderror </div> <div class="form-group"> <label for="description">Product Description:</label> <textarea type="text" wire:model="description" class="form-control" id="taskDescription">{{{ $description ?? '' }}}</textarea> @error('description') <span class="error">{{ $message }}</span> @enderror </div> <button class="btn btn-primary nextBtn btn-lg pull-right" wire:click="firstStepSubmit" type="button" >Next</button> </div> </div> </div> <div class="row setup-content {{ $currentStep != 2 ? 'displayNone' : '' }}" id="step-2"> <div class="col-xs-12"> <div class="col-md-12"> <h3> Step 2</h3> <div class="form-group"> <label for="description">Product Status</label><br/> <label class="radio-inline"><input type="radio" wire:model="status" value="1" {{{ $status == '1' ? "checked" : "" }}}> Active</label> <label class="radio-inline"><input type="radio" wire:model="status" value="0" {{{ $status == '0' ? "checked" : "" }}}> DeActive</label> @error('status') <span class="error">{{ $message }}</span> @enderror </div> <div class="form-group"> <label for="description">Product Stock</label> <input type="text" wire:model="stock" class="form-control" id="productAmount"/> @error('stock') <span class="error">{{ $message }}</span> @enderror </div> <button class="btn btn-primary nextBtn btn-lg pull-right" type="button" wire:click="secondStepSubmit">Next</button> <button class="btn btn-danger nextBtn btn-lg pull-right" type="button" wire:click="back(1)">Back</button> </div> </div> </div> <div class="row setup-content {{ $currentStep != 3 ? 'displayNone' : '' }}" id="step-3"> <div class="col-xs-12"> <div class="col-md-12"> <h3> Step 3</h3> <table class="table"> <tr> <td>Product Name:</td> <td><strong>{{$name}}</strong></td> </tr> <tr> <td>Product Amount:</td> <td><strong>{{$amount}}</strong></td> </tr> <tr> <td>Product status:</td> <td><strong>{{$status ? 'Active' : 'DeActive'}}</strong></td> </tr> <tr> <td>Product Description:</td> <td><strong>{{$description}}</strong></td> </tr> <tr> <td>Product Stock:</td> <td><strong>{{$stock}}</strong></td> </tr> </table> <button class="btn btn-success btn-lg pull-right" wire:click="submitForm" type="button">Finish!</button> <button class="btn btn-danger nextBtn btn-lg pull-right" type="button" wire:click="back(2)">Back</button> </div> </div> </div> </div>
Create Blade Template File
Create a blade template file default.blade.php inside /resources/views folder.
Open default.blade.php and write this following code into it.
<!DOCTYPE html> <html> <head> <title>Laravel 9 Livewire Multistep Form Wizard Tutorial</title> @livewireStyles <script src="//cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <link href="//maxcdn.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="//maxcdn.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js"></script> <link href="{{ asset('wizard.css') }}" rel="stylesheet" id="bootstrap-css"> </head> <body> <div class="container"> <div class="card"> <div class="card-header bg-primary text-white text-center"> Laravel 9 Livewire Multistep Form Wizard Tutorial </div> <div class="card-body"> <livewire:wizard /> </div> </div> </div> </body> @livewireScripts </html>
Add Wizard Custom CSS
Create a file wizard.css inside /public folder. Open that file and write this code into it.
body { margin-top: 40px; } .stepwizard-step p { margin-top: 10px; } .stepwizard-row { display: table-row; } .stepwizard { display: table; width: 100%; position: relative; } .stepwizard-step button[disabled] { opacity: 1 !important; filter: alpha(opacity=100) !important; } .stepwizard-row:before { top: 14px; bottom: 0; position: absolute; content: " "; width: 100%; height: 1px; background-color: #ccc; } .stepwizard-step { display: table-cell; text-align: center; position: relative; } .btn-circle { width: 30px; height: 30px; text-align: center; padding: 6px 0; font-size: 12px; line-height: 1.428571429; border-radius: 15px; } .displayNone { display: none; } span.error { color: red; }
Add Route
Open web.php file from /routes folder. Add this route into it.
//... Route::get('wizard', function () { return view('default'); });
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/wizard
We have successfully created a multi step form wizard in laravel livewire.
Step #1
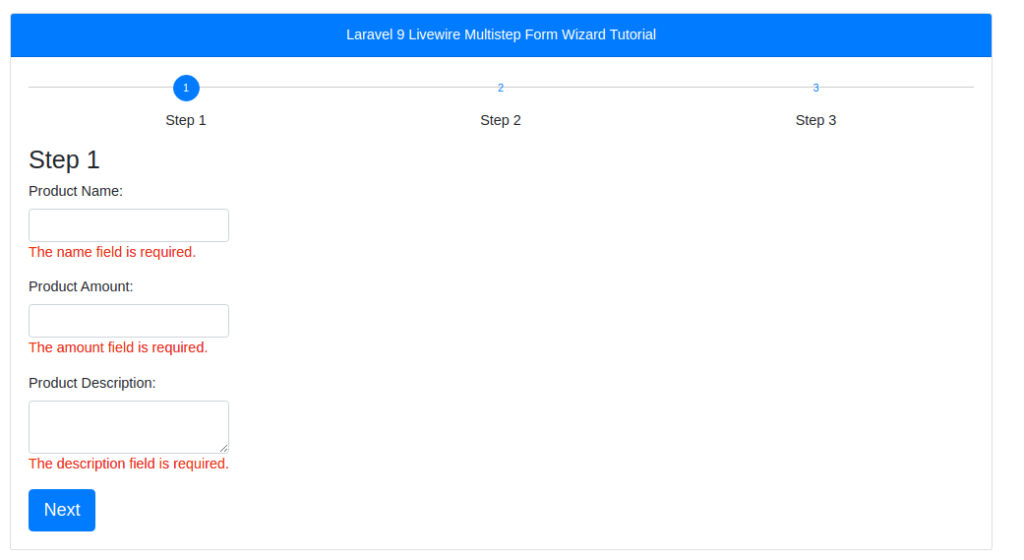
Step #2
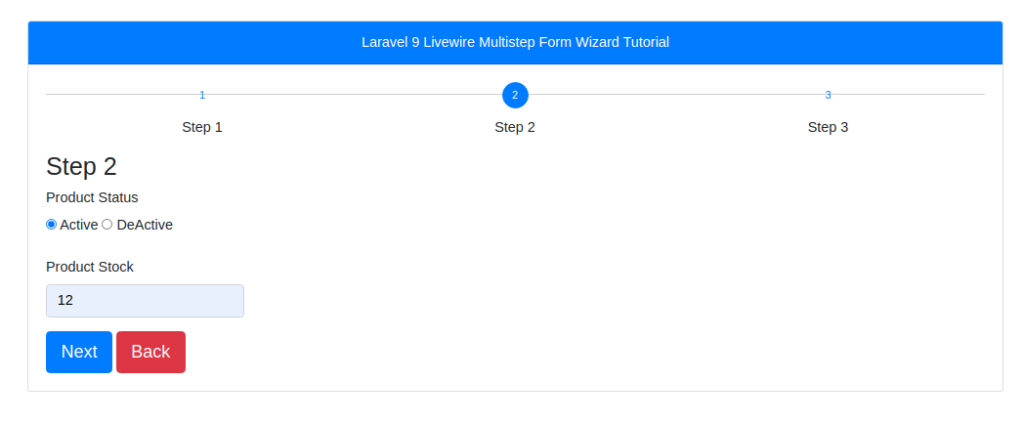
Step #3
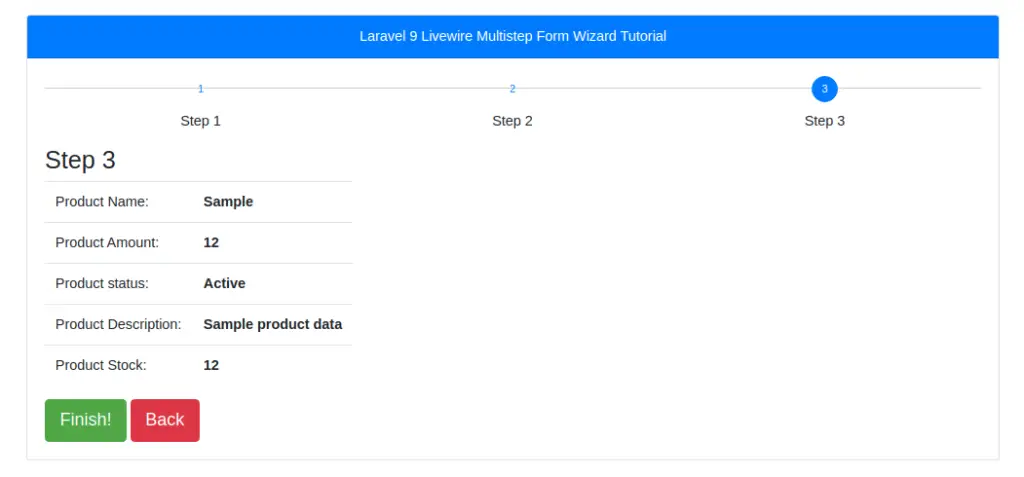
We hope this article helped you to Laravel 9 Livewire Multi step Form Wizard Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.