Inside this article we will see the concept i.e Password Generator with Strength Checker in JavaScript Example Tutorial. Article contains the classified information about Building a Simple Password Strength Checker in Javascript.
Having a secured and strong password is always important to web applications to avoid the chances of Brute force attack or any other security breach.
Read More: How To Encrypt and Verify Password in PHP Tutorial
Let’s get started.
Application Programming
Create a folder say password-generator in your localhost directory. Create two files into it:
- index.html
- validate.js
Design Sign Up Form
Open index.html and write this code into it. It will create the frontend form where user can generate password.
<!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Password Generator with Strength Checker in JavaScript</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/js/bootstrap.min.js"></script> <script src="validate.js"></script> <style> #strengthWrapper { width: 200px; height: 35px; border-radius: 3px; } </style> </head> <body> <div class="container"> <h4>Password Generator with Strength Checker in JavaScript</h4> <br><br> <div class="row"> <div class="col-md-6"> <div class="col-md-12"> <form name="form" class="form" action="#" method="post"> <div class="row"> <div class="form-group col-md-6" style="padding: 0px 2px 0px 15px"> <input type="text" name="password" id="password" class="form-control" placeholder="Password.."> </div> <div class="form-group col-md-4" style="padding: 1px 2px 3px 1px"> <input type="button" id="generatePassword" class="btn btn-primary btn-md" value="Generate Password"> </div> </div> <div class="row hidden" id="strengthSection"> <div class="form-group col-md-6"> <div id="strengthWrapper"> <div class="form-control" id="strength"></div> </div> </div> </div> </form> </div> </div> </div> </div> </body> </html>
Read More: Useful JavaScript console.log() Tricks Which You Need To Know
Strong Password Generator Settings
Open validate.js file and write this complete code into it.
$(document).ready(function() { $("#password").keyup(function() { validatePassword(); }); $("#generatePassword").click(function() { var password = Array(20).fill('0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz~!@-#$') .map(x => x[Math.floor(crypto.getRandomValues(new Uint32Array(1))[0] / (0xffffffff + 1) * x.length)]).join(''); $("#password").val(password); validatePassword(); }); }); function validatePassword() { var pass = $("#password").val(); if (pass != "") { $("#strengthSection").removeClass('hidden'); if (pass.length <= 8) { $("#strength").css("background-color", "#FF0000").text("Very Weak").animate({ width: '200px' }, 300); } if (pass.length > 8 && (pass.match(/[a-z]/) || pass.match(/\d+/) || pass.match(/.[!,@,#,$,%,^,&,*,?,_,~,-,(,)]/))) { $("#strength").css("background-color", "#F5BCA9").text("Weak").animate({ width: '200px' }, 300); } if (pass.length > 8 && ((pass.match(/[a-z]/) && pass.match(/\d+/)) || (pass.match(/\d+/) && pass.match(/.[!,@,#,$,%,^,&,*,?,_,~,-,(,)]/)) || (pass.match(/[a-z]/) && pass.match(/.[!,@,#,$,%,^,&,*,?,_,~,-,(,)]/)))) { $("#strength").css("background-color", "#FF8000").text("Good").animate({ width: '200px' }, 300); } if (pass.length > 8 && pass.match(/^(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[^a-zA-Z0-9])(?!.*\s).{8,24}$/)) { $("#strength").css("background-color", "#00FF40").text("Strong").animate({ width: '200px' }, 300); } } else { $("#strength").animate({ width: '200px' }, 300); $("#strengthSection").addClass('hidden'); } }
Application Testing
Open browser and type your application URL:
URL: http://localhost/password-generator/index.html
Whenever you type any password value into input, it will check it’s strength.
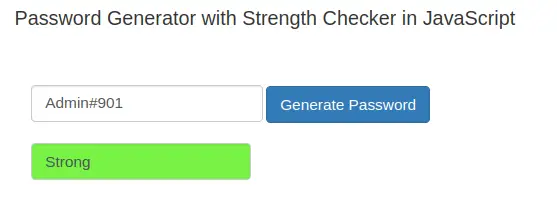
Read More: Laravel Check For File Existence Inside Storage Location
Next, if you want to generate a strong password instead of typing. Click on Generate Password button.
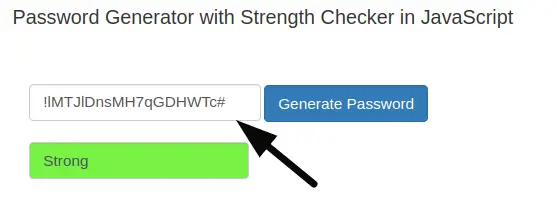
We hope this article helped you to learn Password Generator with Strength Checker in JavaScript Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.