Passing parameters to shortcodes is super easy to do. In the last tutorial we have seen about the concept of self-closed shortcode in wordpress. We will continue the same example but we pass some parameters into it.
Syntax to pass parameters to shortcode
[shortcode_name key1="values1" key2="value2" ...]
Examples
[social_links fb_username="onlinewebtutor" tw_username="skillshike"]
Here, fb_username and tw_username are keys of shortcode whereas onlinewebtutor and skillshike are values of those. We read these values with callback function of shortcode. You can define your own keys and values.
In case if you want to create shortcodes with optional parameters then you can also do it. We will use wordpress function shortcode_atts().
shortcode_atts( $defalt_array, $attr )
This is a wordpress function used to define attributes and their default values for shortcode. It takes 2 parameters and both are required.
- $defalt_array – an array with attributes and their values.
- $attr – an array of attributes that we pass through shortcode.
$args = shortcode_atts(array(
// default values
'fb_username' => 'onlinewebtutor', // facebook username
'tw_username' => 'skillshike' // twitter username
), $attributes);
$attributes will be an array of key value pairs. It may contain fb_username and tw_username.
If key doesn’t exists inside $attributes then default values will be used as defined above.
Here, is the complete code of plugin.
<?php
/*
Plugin Name: Simple Basic Shortcode
Description: It shows social links of facebook and twitter into website
Author: Online Web Tutor
Author URI: https://onlinewebtutorblog.com/
License: GPLv2 or later
License URI: https://www.gnu.org/licenses/gpl-2.0.html
Text Domain: basic-shortcode
Version: 1.0
*/
// Creating shortcode with multiple attributes
function owt_basic_shortcode_social_links($attributes)
{
$args = shortcode_atts(array(
// default values
'fb_username' => 'owthub', // facebook username
'tw_username' => 'owthub' // twitter username
), $attributes);
$facebook = "<a href='https://www.facebook.com/" . $args['fb_username'] . "'>Follow Us On facebook</a>";
$twitter = "<a href='https://www.twitter.com/" . $args['tw_username'] . "'>Follow Us On Twitter</a>";
$output = $facebook . '</br>' . $twitter;
return $output;
}
add_shortcode('social_links', 'owt_basic_shortcode_social_links');
You will see inside Plugins >> Installed Plugins

Activate this plugin to use.
Case #1
In your post/page you can use this shortcode.
[social_links fb_username="onlinewebtutor" tw_username="onlinetutor"]
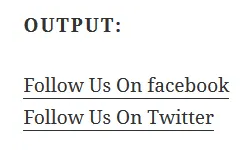
The links for facebook and twitter will be like this –
https://www.facebook.com/onlinewebtutor
https://www.twitter.com/onlinetutor
Case #2
If we don’t pass value of a key like twitter username –
[social_links fb_username="onlinewebtutor"]
The links for facebook and twitter will be like this –
https://www.facebook.com/onlinewebtutor
https://www.twitter.com/owthub
Default value for twitter username used.
Successfully, we have now created self-closed shortcode with parameters in wordpress.