Inside this article we will understand CakePHP 4 How To Seed Data in Database Table. Article contains classified information about generating seeders for database table.
Seeders are those files which generates “test data” for database table. Database Seeding means Inserting Sample data inside database tables. This is usually we do to Test application.
In this article we will seed data for table products. Product table will have the columns and their attributes as –
- id [INTEGER]
- name [STRING]
- cost [INTEGER]
- product_image [STRING]
- description [TEXT]
- status [ENUM]
- created_at [TIMESTAMP]
CakePHP CLI gives a smart option to create seeder files. We will use bin/cake CLI to generate seeder files.
Learn More –
- CakePHP 4 How To Add Column in Table Using Migration
- CakePHP 4 How To Check All Routes of Application
- CakePHP 4 How To Check Migrations Status Tutorial
- CakePHP 4 How To Create Migrations For Database
Let’s get started.
CakePHP 4 Installation
To create a CakePHP project, run this command into your shell or terminal. Make sure composer should be installed in your system.
$ composer create-project --prefer-dist cakephp/app:~4.0 mycakephp
Above command will creates a project with the name called mycakephp.
Database Connection
Open app_local.php file from /config folder. Search for Datasources. Go to default array of it.
You can add your connection details here to connect with your database. It will be like this –
//... 'Datasources' => [ 'default' => [ 'host' => 'localhost', /* * CakePHP will use the default DB port based on the driver selected * MySQL on MAMP uses port 8889, MAMP users will want to uncomment * the following line and set the port accordingly */ //'port' => 'non_standard_port_number', 'username' => 'root', 'password' => 'sample@123', 'database' => 'mydatabase', /* * If not using the default 'public' schema with the PostgreSQL driver * set it here. */ //'schema' => 'myapp', /* * You can use a DSN string to set the entire configuration */ 'url' => env('DATABASE_URL', null), ], //... //...
You can pass host, username, password and database.
Successfully, you are now connected with the database.
Create Migration
Let’s create a migration file for “products” table.
Open project into terminal and run this command
$ bin/cake bake migration CreateProducts
This command will create a migration file inside /config/Migrations folder. File name will be prefixed with the timestamp value and look like 20220217021429_CreateProducts.php
Open migration file and write this code into it to create “products” table schema.
<?php declare(strict_types=1); use Migrations\AbstractMigration; class CreateProducts extends AbstractMigration { /** * Change Method. * * More information on this method is available here: * https://book.cakephp.org/phinx/0/en/migrations.html#the-change-method * @return void */ public function change() { $table = $this->table('products'); $table->addColumn('name', 'string', [ 'limit' => 120, 'null' => false, ]); $table->addColumn('cost', 'integer', [ 'limit' => 5, 'null' => false, ]); $table->addColumn('product_image', 'string', [ 'limit' => 220, 'null' => true, ]); $table->addColumn('description', 'text', [ 'null' => true, ]); $table->addColumn('status', 'enum', [ 'values' => [1, 0], 'default' => 1 ]); $table->addColumn('created_at', 'timestamp', [ 'default' => 'CURRENT_TIMESTAMP', 'null' => false, ]); $table->create(); } }
Run Migration
Open terminal and run this command to create table schema.
$ bin/cake migrations migrate
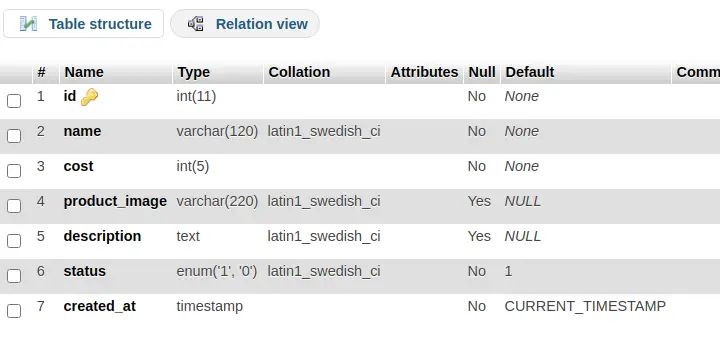
In this table we will seed data.
Create Data Seeder
To create data seeder, run this command to terminal.
$ bin/cake bake seed Products
It will create a file ProductsSeed.php inside /config/Seeds folder.
Open ProductsSeed.php and write this code into it.
<?php declare(strict_types=1); use Migrations\AbstractSeed; /** * Products seed. */ class ProductsSeed extends AbstractSeed { /** * Run Method. * * Write your database seeder using this method. * * More information on writing seeds is available here: * https://book.cakephp.org/phinx/0/en/seeding.html * * @return void */ public function run() { $data = [ [ "name" => "Sample Product 1", "cost" => 140, "product_image" => "https://i.picsum.photos/id/704/200/300.jpg", "description" => "This is sample content for this Sample Product 1", "status" => 1 ], [ "name" => "Sample Product 2", "cost" => 180, "product_image" => "https://i.picsum.photos/id/973/200/200.jpg", "description" => "This is sample content for this Sample Product 2", "status" => 1 ], [ "name" => "Sample Product 3", "cost" => 130, "product_image" => "https://i.picsum.photos/id/973/250/250.jpg", "description" => "This is sample content for this Sample Product 3", "status" => 0 ] ]; $table = $this->table('products'); $table->insert($data)->save(); } }
Inside above code we are inserting 3 Test rows of products into table.
Run Seeder
Back to terminal and run this command to seed data into table.
$ bin/cake migrations seed
This command will run the seeder from Seeds folder.
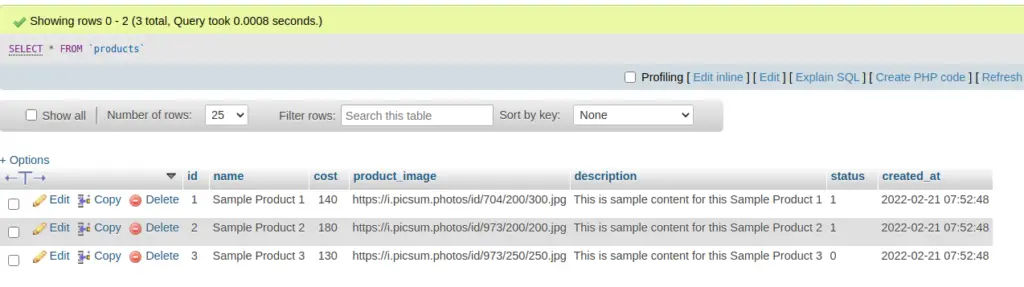
We hope this article helped you to learn about CakePHP 4 How To Seed Data in Database Table Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.