Inside this article we will see the concept i.e CodeIgniter 4 How To Work with MySQL Joins Tutorial. Article contains the classified information about CodeIgniter 4 Query Builder join() Methods.
When we work with MySQL Queries, then definitely for some relational data we need to work with Joins. Inside this article we will see the concept of Joins in CodeIgniter 4.
Joins in CodeIgniter 4 is the connection between one and more tables to get data. In MySQL we have Inner join, Left join, Right join, Outer join.
Learn More –
- Concept of Seeders in CodeIgniter 4 Tutorial
- Concept of Trait in CodeIgniter 4 Tutorial with Example
- Create Custom 404 Page in CodeIgniter 4 | Page Not Found
- Create Custom Command in CodeIgniter 4
Let’s get started.
Types of Joins available in CodeIgniter 4
In CodeIgniter 4 application, as per the documentation we have 3 types of joins available. Here are the followings –
- Inner Join
- Left Join
- Right Join
Inner Join – This join brings the data on the basis of a common value condition between two or more than two tables. According to matched condition it will bring all data what we expected for. It remove those rows from result set which has no matched condition.
Left Join – This also works same the matched condition between two or more than two tables. But in this case we also get the rows of left table which doesn’t match with the condition with the right hand sided table. Means we get all rows of left table including values of matched with right table.
Right Join – Same matched condition rows including right sided all rows with no condition matched.
Have a look into the image about inner join, left join, right join.
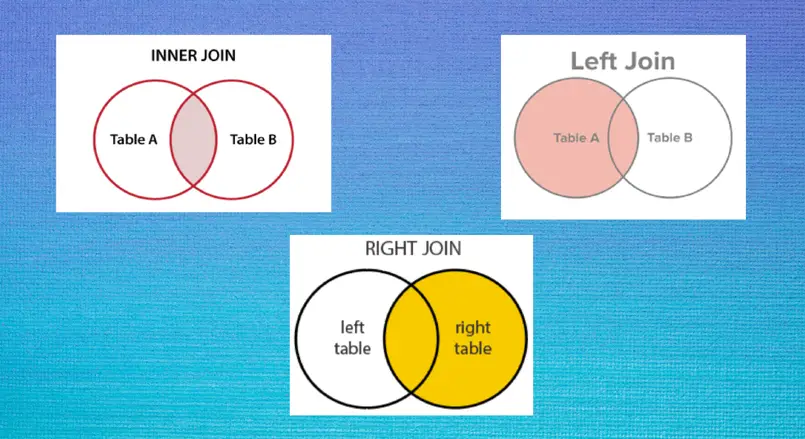
How can we use inside an application and get relational data we will see by making an application. Let’s create an application in which we use followings.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Also if you want to remove public & index.php from URL – Remove Public & Index.php from CodeIgniter 4 URLs.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Create Database
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
We will use MySQL command to create database. Run this command into Sql tab of PhpMyAdmin.
CREATE DATABASE codeigniter4_app;
Successfully, we have created a database.
Create Database Table
Next, we need tables. Those table will be responsible to store data.
Let’s create two tables with some columns.
CREATE TABLE `tbl_users` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(120) DEFAULT NULL,
`email` varchar(120) DEFAULT NULL,
`phone_no` varchar(120) DEFAULT NULL,
`created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
CREATE TABLE `tbl_courses` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(120) NOT NULL,
`user_id` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Table courses contains user_id column on the basis of which we are going to make use of join. Successfully, we have created two tables.
Let’s insert some dummy data into these tables.
Database Connection
Open .env file from project root.
Search for DATABASE. You should see the connection environment variables into it. Put your updated details of database connection string values.
#-------------------------------------------------------------------- # DATABASE #-------------------------------------------------------------------- database.default.hostname = localhost database.default.database = codeigniter4_app database.default.username = admin database.default.password = admin database.default.DBDriver = MySQLi database.default.DBPrefix = database.default.port = 3306
Now, database successfully connected with the application.
Creating Test data for Application
Here we have some sql queries, you need to copy and run into database. It will inserts data into tables.
Test data for tbl_users –
-- -- Dumping data for table `tbl_users` -- INSERT INTO `tbl_users` (`id`, `name`, `email`, `phone_no`, `created_at`) VALUES (1, 'Sanjay', 'sanjay@gmail.com', '8527419630', '2020-09-10 13:19:38'), (2, 'Vijay', 'vijay@gmail.com', '9875643125', '2020-09-25 11:33:00'), (3, 'Ashish Kumar', 'ashish@gmail.com', '7896541230', '2020-09-25 11:33:17');
Test data for tbl_courses –
-- -- Dumping data for table `tbl_courses` -- INSERT INTO `tbl_courses` (`id`, `name`, `user_id`) VALUES (1, 'Learn CodeIgniter 4', 1), (2, 'Learn PHP', 2), (3, 'Learn CakePHP 4', 30), (4, 'Learn Laravel', 35);
To Learn about Seeders in CodeIgniter 4, Click here.
Create Routes
Open Routes.php from /app/Config. Add these routes into this file.
//.. $routes->get('inner-join', 'Site::innerJoinMethod'); $routes->get('left-join', 'Site::leftJoinMethod'); $routes->get('right-join', 'Site::rightJoinMethod'); //...
Let’s create Controller.
Create Controller
Open project into terminal and run this spark command to create controller.
$ php spark make:controller Site
It will create Site.php at /app/Controllers folder.
Open Site.php and write this complete code into it.
<?php namespace App\Controllers; class Site extends BaseController { private $db; public function __construct() { $this->db = db_connect(); // Loading database // OR $this->db = \Config\Database::connect(); } public function innerJoinMethod() { //.... } public function leftJoinMethod() { //.... } public function rightJoinMethod() { //.... } }
Step by step we are going to use Joins in CodeIgniter 4 application.
Inner Join
//... # Method for Inner Join public function innerJoinMethod() { $builder = $this->db->table("tbl_users as user"); $builder->select('user.*, course.name as course_name'); $builder->join('tbl_courses as course', 'user.id = course.user_id'); $data = $builder->get()->getResult(); echo "<pre>"; print_r($data); } //...
Behind the scene executed query is –
SELECT `user`.*, `course`.`name` as `course_name` FROM `tbl_users` as `user` JOIN `tbl_courses` as `course` ON `user`.`id` = `course`.`user_id`
Start development server –
$ php spark serve
Open up the browser and run the route http://localhost:8080/inner-join. Have a look output –
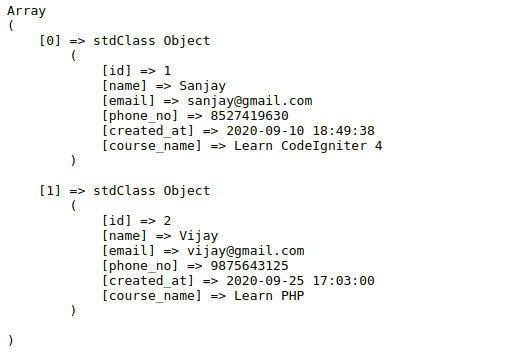
We are getting only matched rows according to the condition user.id = course.user_id
Left Join
//... # Method for Left Join public function leftJoinMethod() { $builder = $this->db->table("tbl_users as user"); $builder->select('user.*, course.name as course_name'); $builder->join('tbl_courses as course', 'user.id = course.user_id', "left"); // added left here $data = $builder->get()->getResult(); echo "<pre>"; print_r($data); } //...
Behind the scene executed query is –
SELECT `user`.*, `course`.`name` as `course_name` FROM `tbl_users` as `user` LEFT JOIN `tbl_courses` as `course` ON `user`.`id` = `course`.`user_id`
Open up the browser and run the route http://localhost:8080/left-join. Have a look output –
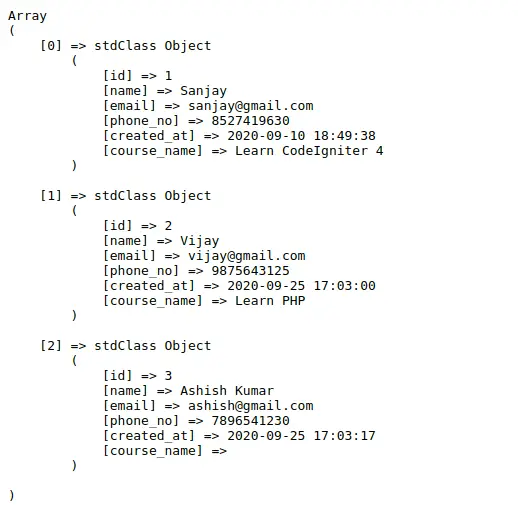
We are getting matched rows including left hand side all rows.
Right Join
//... # Method for Right Join public function rightJoinMethod() { $builder = $this->db->table("tbl_users as user"); $builder->select('user.*, course.name as course_name'); $builder->join('tbl_courses as course', 'user.id = course.user_id', "right"); $data = $builder->get()->getResult(); echo "<pre>"; print_r($data); } //...
Behind the scene executed query is –
SELECT `user`.*, `course`.`name` as `course_name` FROM `tbl_users` as `user` RIGHT JOIN `tbl_courses` as `course` ON `user`.`id` = `course`.`user_id`
Open up the browser and run the route http://localhost:8080/right-join. Have a look output –
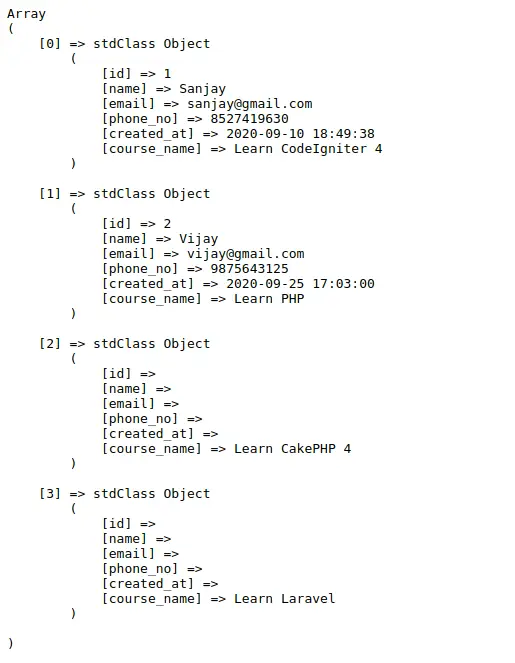
We are getting matched rows including right hand side all rows.
We hope this article helped you to learn about CodeIgniter 4 How To Work with MySQL Joins Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.