Inside this article we will see the concept i.e How To Create a Custom Modal Popup Using jQuery. Article contains the classified information i.e How To Make a Modal Box With CSS and jQuery.
In a web application, it is common to display a popup window that shows information, feedback, contact forms, or confirmation messages. Fortunately, with the help of the jQuery library, implementing a custom popup is simple. Such a popup window opens independently of the current web page elements and does not require user interaction.
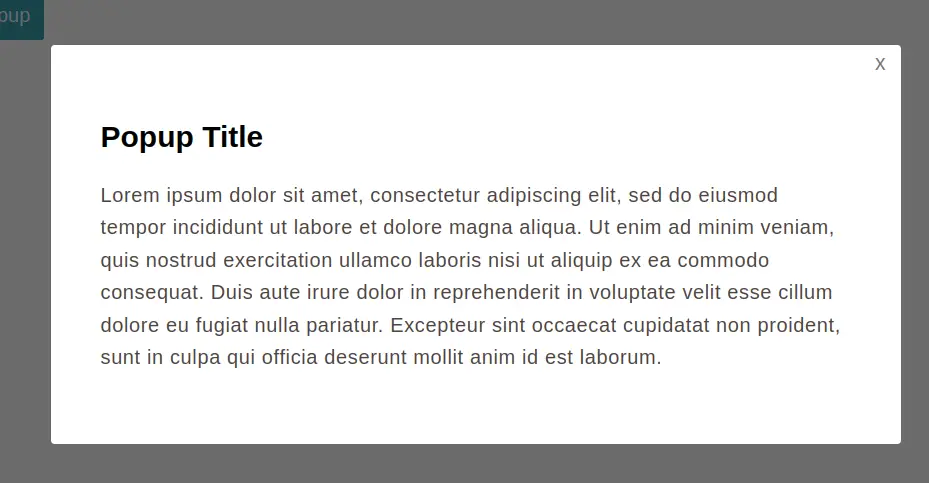
Read More: How to Use Chat GPT: Step by Step Guide to Start ChatGPT
Let’s get started.
Steps To Create Popup Modal Using jQuery
Here, you will have a very simple basic points by following you can create modal popup:
- Create an HTML
- Add CSS
- Write a jQuery script
- Output
Create an HTML webpage
First, we will create an index.html file and paste the following HTML code that creates modal popup.
<!DOCTYPE html> <html> <head> <title>How To Create a Modal Popup Using jQuery - Online Web Tutor</title> </head> <body> <h2>Create a Modal Popup Using jQuery - <a href="https://onlinewebtutorblog.com/" target="_blank" rel="noopener noreferrer">Online Web Tutor</a></h2> <a class="openBtn" href="javascript:void(0)"> Click To Open Popup </a> <div class="popup"> <div class="popup-content"> <h2>Popup Title</h2> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p> <a class="closeBtn" href="javascript:void(0)">x</a> </div> </div> </body> </html>
Let’s add CSS into it.
Read More: The Ultimate Guide to WordPress Custom Post Types
Add CSS
Now, we need to style the popup so that we will add the following CSS in the index.html
file before the closing head (</head>
) tag.
<style type="text/css"> body { font-family: Helvetica, Arial, sans-serif; } p { font-size: 16px; line-height: 26px; letter-spacing: 0.5px; color: #4f4343; } /* Popup open button */ .openBtn { color: #FFF; background: #269faf; padding: 10px; text-decoration: none; border: 1px solid #269faf; border-radius: 3px; } .openBtn:hover { background: #35c7db; } .popup { position: fixed; top: 0px; left: 0px; background: rgba(0, 0, 0, 0.58); width: 100%; height: 100%; display: none; } /* Popup inner div */ .popup-content { width: 600px; margin: 0 auto; padding: 40px; margin-top: 100px; border-radius: 3px; background: #fff; position: relative; } /* Popup close button */ .closeBtn { position: absolute; top: 5px; right: 12px; font-size: 17px; color: #7c7575; text-decoration: none; } </style>
Write a jQuery Script
To use jquery functions in webpage, you need to first include this jquery library link.
So, you have to add the following jQuery library in the head section of the page.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
Finally, we will write a jQuery script to trigger the action whenever users click to open/close a popup window.
Read More: How to Speed Up Your WordPress Website: A Beginner’s Guide
Add the following script in the index.html
file before the closing body (</body>
) tag.
<script type="text/javascript"> $(document).ready(function () { // Open Popup $('.openBtn').on('click', function () { $('.popup').fadeIn(300); }); // Close Popup $('.closeBtn').on('click', function () { $('.popup').fadeOut(300); }); // Close Popup when Click outside $('.popup').on('click', function () { $('.popup').fadeOut(300); }).children().click(function () { return false; }); }); </script>
Complete Output
Let’s combine all code into index.html web page.
<!DOCTYPE html> <html> <head> <title>How To Create a Modal Popup Using jQuery - Online Web Tutor</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <style type="text/css"> body { font-family: Helvetica, Arial, sans-serif; } p { font-size: 16px; line-height: 26px; letter-spacing: 0.5px; color: #4f4343; } /* Popup open button */ .openBtn { color: #FFF; background: #269faf; padding: 10px; text-decoration: none; border: 1px solid #269faf; border-radius: 3px; } .openBtn:hover { background: #35c7db; } .popup { position: fixed; top: 0px; left: 0px; background: rgba(0, 0, 0, 0.58); width: 100%; height: 100%; display: none; } /* Popup inner div */ .popup-content { width: 600px; margin: 0 auto; padding: 40px; margin-top: 100px; border-radius: 3px; background: #fff; position: relative; } /* Popup close button */ .closeBtn { position: absolute; top: 5px; right: 12px; font-size: 17px; color: #7c7575; text-decoration: none; } </style> </head> <body> <h2>Create a Modal Popup Using jQuery - <a href="https://onlinewebtutorblog.com/" target="_blank" rel="noopener noreferrer">Online Web Tutor</a></h2> <a class="openBtn" href="javascript:void(0)"> Click To Open Popup </a> <div class="popup"> <div class="popup-content"> <h2>Popup Title</h2> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.</p> <a class="closeBtn" href="javascript:void(0)">x</a> </div> </div> <script type="text/javascript"> $(document).ready(function () { // Open Popup $('.openBtn').on('click', function () { $('.popup').fadeIn(300); }); // Close Popup $('.closeBtn').on('click', function () { $('.popup').fadeOut(300); }); // Close Popup when Click outside $('.popup').on('click', function () { $('.popup').fadeOut(300); }).children().click(function () { return false; }); }); </script> </body> </html>
Run the project and check the output in the browser.
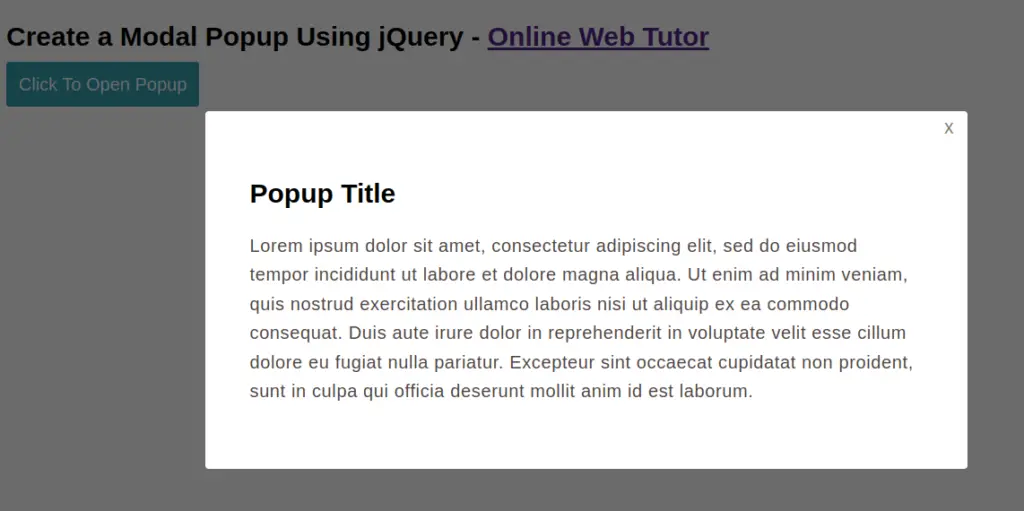
We hope this article helped you to learn about How To Create a Custom Modal Popup Using jQuery Tutorial in a very detailed way.
Read More: Top Best Free WordPress Plugins for Image Optimization Tutorial
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.