Inside this article we will see How to generate QR code using Javascript or jQuery. This article is super easy to understand as well as to implement in your code.
We will generate QR code without any kind of package or plugin instead we will create QR code using an API. By using this concept you can create dynamic QR code using QR code API. Additionally using this API, you can customize as per your requirements of QR Code like size, color, bgcolor, margin, format etc.
QR Code API is very easy to use for generate QR code using javascript.
Learn More –
- How To Integrate CKEditor 4 in HTML And jQuery
- How to Prevent Styles and JavaScript Files From Cached
- Javascript Auto Logout in CodeIgniter 4 Tutorial
- jQuery Datatables Header Misaligned With Vertical Scrolling
Let’s get started.
About QR Code API
QR Code api helps to customize all types of settings like size, color, bgcolor, margin, format etc.
API URL
http(s)://api.qrserver.com/v1/create-qr-code/?data=[URL-encoded-text]&size=[pixels]x[pixels]
We hit this API URL using GET request type with few parameters.
create-qr-code is command API URL,
Query string parameters – data = pass text as per requirement, size = 100*100
Create an Application
Create a folder with name js-qrcode. Inside this folder create a file index.php.
Open index.php and write this complete code into it.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>How To Generate QR Code Using Javascript</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <style type="text/css" media="screen"> { margin: 0; padding: 0; box-sizing: border-box; font-family: Quicksand; } body { width: 100%; height: 100vh; display: flex; justify-content: center; align-items: center; } .main { width: 50%; padding: 0 15px; display: flex; justify-content: center; align-items: center; flex-direction: column; background: #fff; box-shadow: 0 10px 25px -10px rgba(0, 0, 0, 0.5); border-radius: 5px; } .main p { font-size: 25px; } .main .input { width: 90%; padding: 10px 25px; border: 3px solid #9e9e9e; outline: none; margin: 15px 0 40px; } .main .input:focus { border: 3px solid #439cfb; } .btn, .input { font-size: 1.1rem; border-radius: 5px; } .main .btn { width: 90%; padding: 12px 0; outline: none; border: none; border-radius: 5px; background: #439cfb; color: #fff; transition: 0.3s; margin: 0 0 25px 0; } .main .code { margin: 25px 0; } .main .btn:hover { box-shadow: 0 10px 25px -10px #439cfb; } .main #note { font-size: 1.2rem; font-family: 'Courier New', Courier, monospace; } #toast { position: absolute; bottom: 0; border-radius: 5px; padding: 15px 50px; background: #07f49e; opacity: 0; visibility: hidden; box-shadow: 0 10px 25px -10px #07f49e; transition: 0.3s; } #toast.show { visibility: visible; opacity: 1; bottom: 50px; } </style> <body> <div class="main"> <p>How To Generate QR Code Using Javascript</p> <input type="text" class="input" value="" id="textvalue"> <button class="btn">Generate</button> <img src="images/default.png" alt="Your QR Code will be show here..." class="code"> </div> <div id="toast">Successfully Generated, please wait</div> <script> var code = document.querySelector('.code'); var toast = document.querySelector('#toast'); $(function(){ $(document).on("click", ".btn", function(){ generate(); }); }); function generate() { var data = $('#textvalue').val(); var url = `https://api.qrserver.com/v1/create-qr-code/?size=150x150&data=${data}`; code.src = url; toastDiv(); } function toastDiv() { toast.className = "show"; setTimeout(function() { toast.className = toast.className.replace("show", ""); }, 2000); } </script> </body> </html>
- Currently, images/default.png not available. You can put your own image as a default placeholder.
- var data = $(‘#textvalue’).val(); To read input value when we click on Generate button
- API URL https://api.qrserver.com/v1/create-qr-code/?size=150×150&data=${data} with dynamic data.
Application Testing
Open browser and type this –
URL – http://localhost/js-qrcode/index.php
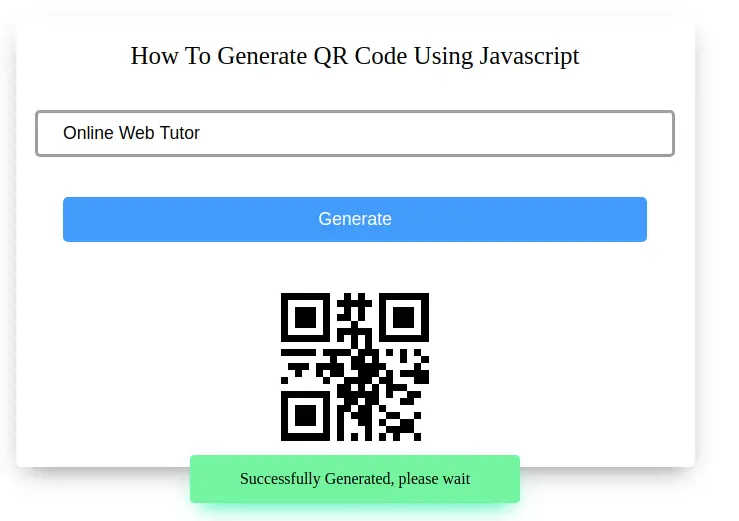
We hope this article helped you to How To Generate QR Code Using Javascript or jQuery Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.