Inside this article we will see available techniques by the help of which we can remove array element from an array in javascript. By using splice method, using delete operator etc we can unset an array element from array in javascript.
Using this tutorial, we will see how to remove array element from array in javascript. This article will be super easy to understand and also you can implement this code very easily.
Learn More –
- Complete jQuery DataTable Tutorial
- Copy To Clipboard Functionality Using JQuery / Javascript
- Create Profile Image of First Letter of First & Last Name
- Download File with Timer Count – Javascript
Let’s get started.
Method #1 Using splice() Method
The splice()
method adds and/or removes array elements.
array.splice(index, howmany, item1, ....., itemX)
index: Required. The position to add/remove items. Negative values a the position from the end of the array.
howmany: Optional. Number of items to be removed.
item1, item2, …: Optional. New elements(s) to be added

Example
var names = ["Sanjay", "Vijay", "Ashish", "Dhananjay"]; console.log(names); //Output (4) ["Sanjay", "Vijay", "Ashish", "Dhananjay"] #Remove elements names.splice(1,2); //Output (2) ["Vijay", "Ashish"]
Method #2 Using delete Operator
The JavaScript delete
operator removes a property from an object.
delete expression;
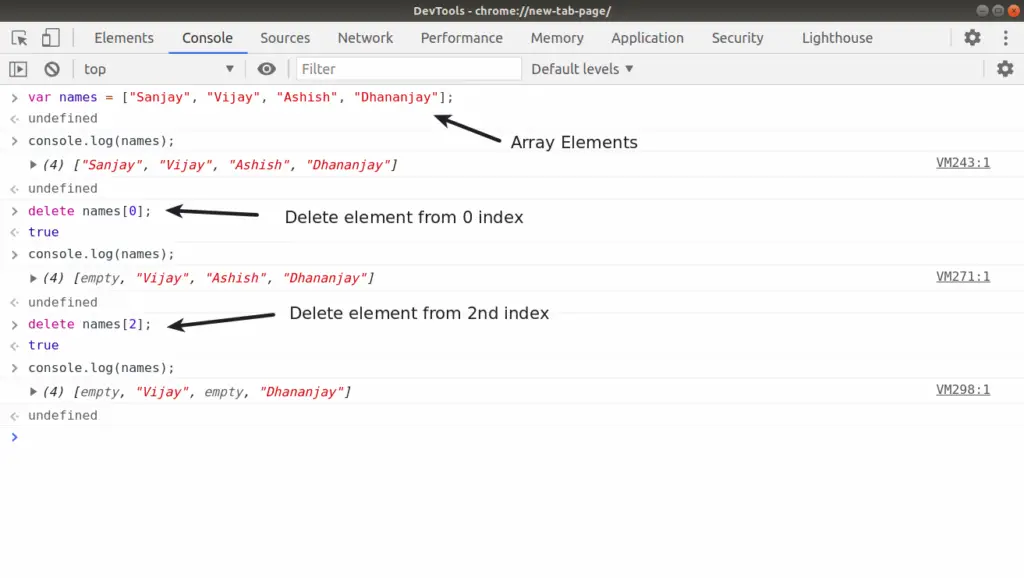
Example
var names = ["Sanjay", "Vijay", "Ashish", "Dhananjay"]; console.log(names); //Output (4) ["Sanjay", "Vijay", "Ashish", "Dhananjay"] #Remove an element delete names[0]; //Output true console.log(names); //Output (4) [empty, "Vijay", "Ashish", "Dhananjay"]
Method #3 Remove an Element by User Function
Here, we will create a user defined function. This function will search an element from given array and remove it.

Example
function remove(arr, what) { var found = arr.indexOf(what);while (found !== -1) {
arr.splice(found, 1);
found = arr.indexOf(what);
}
} var names = ["Sanjay", "Vijay", "Ashish", "Dhananjay"]; remove(names, "Ashish"); console.log(names); //Output (3) ["Sanjay", "Vijay", "Dhananjay"]
Method #4 Remove Element Using Filter Method

Example
var names = ["Sanjay", "Vijay", "Ashish", "Dhananjay"]; console.log(names); //Output (4) ["Sanjay", "Vijay", "Ashish", "Dhananjay"] names = names.filter(e => e !== 'Ashish'); //Output (3) ["Sanjay", "Vijay", "Dhananjay"] names = names.filter(e => e !== 'Vijay'); //Output (2) ["Sanjay", "Dhananjay"]
We hope this article helped you to learn How To Remove Array Element From Array in Javascript in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.