Google Pie Charts are an effective approach to illustrate data composition and distribution in online applications visually. To generate dynamic and interactive pie charts, Laravel, a sophisticated PHP framework, can be easily linked with Google Charts.
We will walk you through the process of integrating Google Pie Charts in Laravel 10, allowing you to present data in an appealing and informative manner.
Throughout this article, we’ll look at how to build up a Laravel project, integrate Google Charts, and create dynamic pie charts that can display data in real time.
Read More: Laravel 10 Create a Dynamic Line Chart Using HighChart
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Setup Data Model & Migration
Consider users table to work with this Pie chart tutorial.
By default, you should see the users table migration (2014_10_12_000000_create_users_table.php inside /database/migrations folder) and User.php model in /app/Models.
We will work with these files.
Run Migration
Next, we need to create tables inside database.
php artisan migrate
This command will create tables inside database.
You will also see users table with all tables in database.
Next,
Prepare Dummy Data for Graph
You need to copy the given mysql query and run into sql tab of phpmyadmin. This will give us a set of data to test.
-- -- Dumping data for table `users` -- INSERT INTO `users` (`id`, `name`, `email`, `email_verified_at`, `password`, `remember_token`, `created_at`, `updated_at`) VALUES (1, 'Emilie Mante', 'kwiegand@example.com', '2023-03-02 17:43:37', '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', 'Y3FP2DroH6', '2023-03-02 17:43:37', '2023-03-02 17:43:37'), (2, 'Reyna Stroman II', 'schuster.carlos@example.com', '2023-03-02 17:43:37', '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', '8tDlUix4oY', '2023-03-02 17:43:37', '2023-03-02 17:43:37'), (3, 'Prof. Lauriane Yost I', 'greta69@example.com', '2023-02-02 17:43:37', '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', 'z6nEz8FkN6', '2023-02-02 17:43:37', '2023-02-02 17:43:37'), (4, 'Zelma Yundt', 'ricardo.cartwright@example.com', '2023-02-02 17:43:37', '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', 'RsOsNYIogy', '2023-02-02 17:43:37', '2023-02-02 17:43:37'), (5, 'Hayley Lebsack', 'elna.tillman@example.com', '2023-03-02 17:43:37', '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', '203SAe79eI', '2023-03-02 17:43:37', '2023-03-02 17:43:37'), (6, 'Topmse Lebsack', 'topse.tillman@net.com', '2023-01-02 17:43:37', '$2y$10$92IXUNpkjO0rOQ5byMi.Ye4oKoEa3Ro9llC/.og/at2.uheWG/igi', '203SAe79eI', '2023-01-02 17:43:37', '2023-01-02 17:43:37');
Right now, you have only these few rows of dummy data. But you can take more rows in it. These data will help to draw graph between Total Registered Users according to Month wise.
Read More: Laravel 10 Create a Dynamic Pie Chart with Highcharts
You will see something like this after this test data insertion.
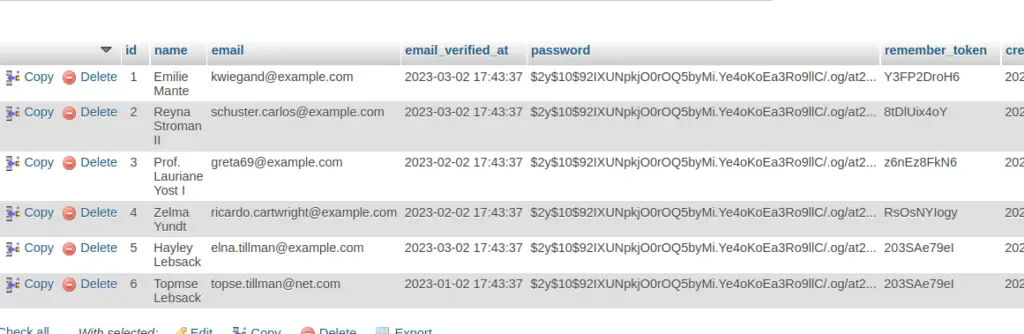
Create Graph Controller
Next, we need to create a controller file,
php artisan make:controller GraphController
It will create a file GraphController.php inside /app/Http/Controllers folder.
Open file and write this complete code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; use Illuminate\Support\Facades\DB; use Illuminate\View\View; class GraphController extends Controller { public function index(): View { $data['pieChart'] = User::select(DB::raw("COUNT(*) as count"), DB::raw("MONTHNAME(created_at) as month_name")) ->whereYear('created_at', date('Y')) ->groupBy('month_name') ->orderBy('count') ->get(); return view('pie-chart', $data); } }
Concept
Here, this is the concept by which you can setup data to be visible into graph.
User::select(DB::raw("COUNT(*) as count"), DB::raw("MONTHNAME(created_at) as month_name"))
->whereYear('created_at', date('Y'))
->groupBy('month_name')
->orderBy('count')
->get();
The date(‘Y’) returns the current year.
That’s why In test data, we have taken the year as 2023 so that we can get a pie chart on the behalf of sample data.
Note*: If your current year is different then you will not be able to see the pie chart view on output.
Create Blade Template File
Go to /resources/views folder and create a file with name pie-chart.blade.php
Open file and write this complete code into it,
<!doctype html> <html lang="en"> <head> <title>Laravel 10 and Google Pie Chart Integration Tutorial</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> </head> <body> <div class="container"> <h3 style="text-align: center;"> Laravel 10 and Google Pie Chart Integration Tutorial </h3> <div id="pie-chart" style="width: 900px; height: 500px"></div> </div> <script type="text/javascript" src="https://www.gstatic.com/charts/loader.js"></script> <script type="text/javascript"> google.charts.load('current', { 'packages': ['corechart'] }); google.charts.setOnLoadCallback(drawChart); function drawChart() { var data = google.visualization.arrayToDataTable([ ['Month Name', 'Registered User Count'], @php foreach ($pieChart as $d) { echo "['" . $d->month_name . "', " . $d->count . '],'; } @endphp ]); var options = { title: 'Users Detail', is3D: false, }; var chart = new google.visualization.PieChart(document.getElementById('pie-chart')); chart.draw(data, options); } </script> </body> </html>
Add Route
Open web.php file from /routes folder. Add this route into it.
//... use App\Http\Controllers\GraphController; //... Route::get('pie-chart', [GraphController::class, 'index']); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Read More: How To Use Laravel 10 to Create a Dynamic Bar Chart
URL: http://127.0.0.1:8000/pie-chart
Output
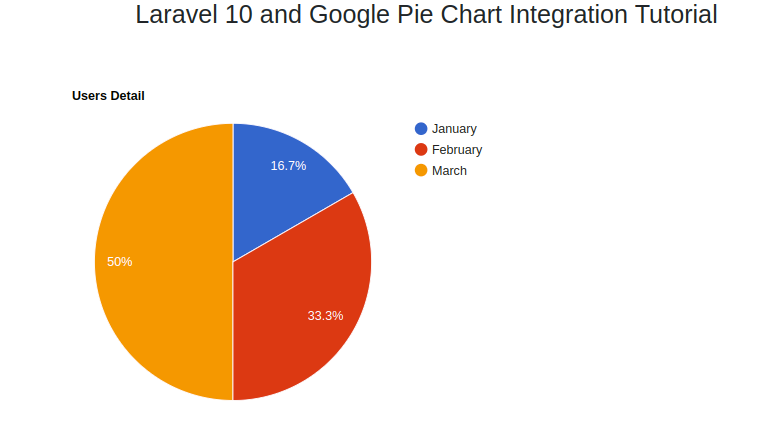
That’s it.
We hope this article helped you to learn about Laravel 10 How To Use Google Pie Chart Example Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.