Inside this article we will see how to create Excel from database with fix header row . We will take database values into an array and then easily we will convert array value to a Excel file with fixed header.
This tutorial will be super easy to understand and implement in your code. We will see the concept of Laravel 8 Create Excel From Database With Fix Header Row.
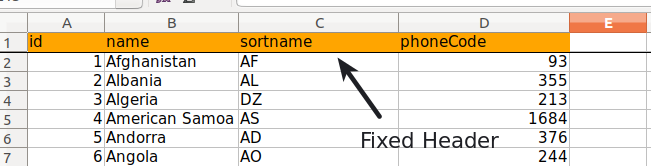
Learn More –
- Laravel 8 Run Case Sensitive Query Tutorial
- Laravel 8 Send Mail using Gmail SMTP Server
- Laravel 8 Send Push Notification to Android Using Firebase
- Laravel 8 Send Push Notification to IOS Using Firebase
Let’s get started.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Database Table Rows
Suppose you have a database table named as countries. In that table you have some kind of data.
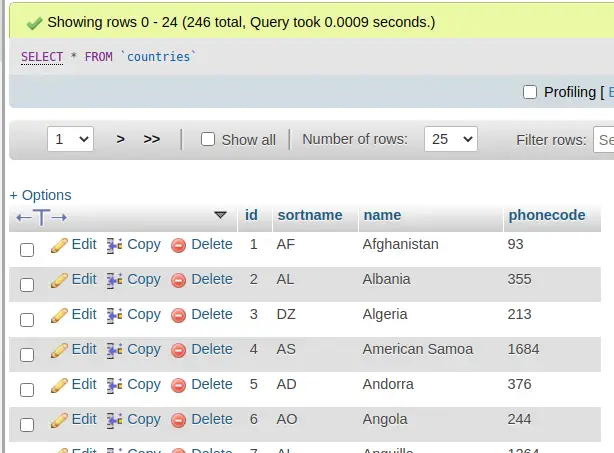
For this tutorial you can take any table from your application. The table we have taken, it’s only for demonstration.
Install maatwebsite/excel Package & Configuration
Open project into terminal and run this composer command to install package.
$ composer require maatwebsite/excel
After package installation, open app.php file from /config folder. Search for providers and aliases add these –
'providers' => [ .... Maatwebsite\Excel\ExcelServiceProvider::class, ], 'aliases' => [ .... 'Excel' => Maatwebsite\Excel\Facades\Excel::class, ],
Create Export Class
Once you will install the above package. You will see we should have a command available in artisan list i.e make:export. We will use this command to create export class.
Open terminal and run this command –
$ php artisan make:export CountryExport
It will create a file CountryExport.php file inside /app/Exports folder.
Open CountryExport.php and write this complete code into it.
<?php namespace App\Exports; use Maatwebsite\Excel\Concerns\FromCollection; use Maatwebsite\Excel\Concerns\WithHeadings; use Maatwebsite\Excel\Concerns\WithEvents; use Maatwebsite\Excel\Events\AfterSheet; class CountryExport implements FromCollection, WithHeadings, WithEvents { protected $data; /** * Write code on Method * * @return response() */ public function __construct($data) { $this->data = $data; } /** * Write code on Method * * @return response() */ public function collection() { return collect($this->data); } /** * Write code on Method * * @return response() */ public function headings(): array { return [ 'id', 'name', 'sortname', 'phoneCode' ]; } /** * Write code on Method * * @return response() */ public function registerEvents(): array { return [ AfterSheet::class => function (AfterSheet $event) { $event->sheet->getDelegate()->getStyle('A1:D1') ->getFill() ->setFillType(\PhpOffice\PhpSpreadsheet\Style\Fill::FILL_SOLID) ->getStartColor() ->setARGB('FFA500'); $event->sheet->getDelegate()->freezePane('A2'); }, ]; } }
Inside registerEvents, we will have event to add background color to cell data. This is A1:D1 cell selected range. Sheet column A1 will be fixed. From A2 all rows will be scrollable.
Create Controller
Next, we need to create a controller file.
$ php artisan make:controller DataExportController
It will create a file DataExportController.php at /app/Http/Controllers folder.
Open DataExportController.php and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Exports\CountryExport; use Maatwebsite\Excel\Facades\Excel; use App\Models\Country; class DataExportController extends Controller { public function export() { $countries = Country::select(["id", "name", "sortname", "phonecode"])->get(); return Excel::download(new CountryExport($countries), 'countries.xlsx'); } }
Create Route
Open web.php from /routes folder and add these route into it.
//... use App\Http\Controllers\DataExportController; Route::get('export', [DataExportController::class, 'export']); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/export
Once you hit this URL, it will download the excel file with all rows from countries table with cell background color with fixed header.
We hope this article helped you to learn Laravel 8 Create Excel From Database With Fix Header Row Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.