In general, a Push notifications are the messages / Notifications that we receive in devices. This really makes the customers/subscribers engage with the client’s content and their updates on digital platforms.
Inside this article, we will see the concept of Laravel 8 Send Push Notification to Android Using Firebase. Step by step guide to implement and use it. Already we have an article of sending firebase push notification on web, Click here to go.
This article assuming you have the concept of little bit of firebase and it’s console. Let’s get started about Laravel 8 Push notification to Android using FCM.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Firebase Project and App
First we need to create a project in Firebase console for web application notification. To create project Click here to go to console.
Creating a Firebase Project at Firebase console.
Step #1
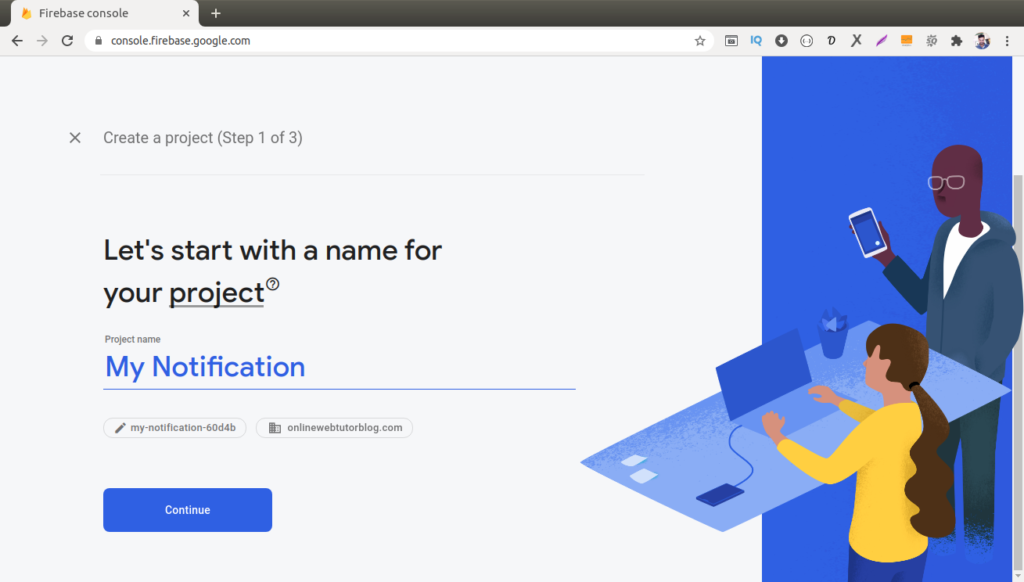
Step #2
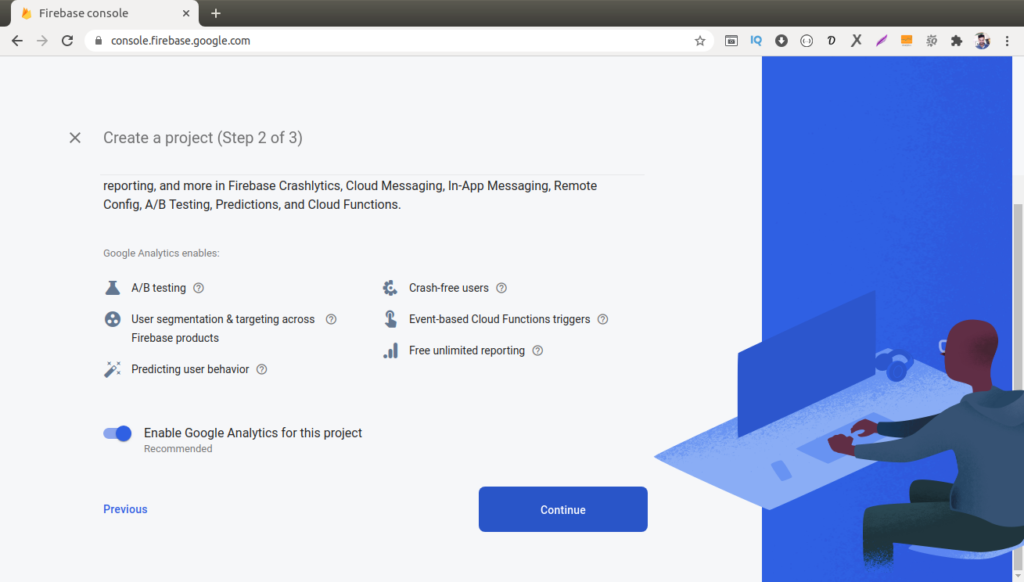
Step #3
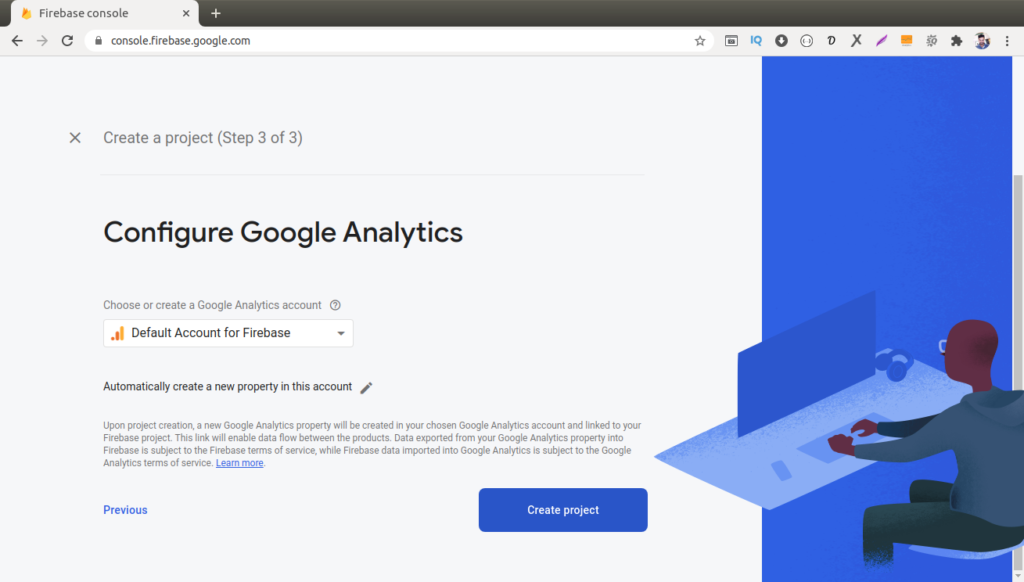
Step #4
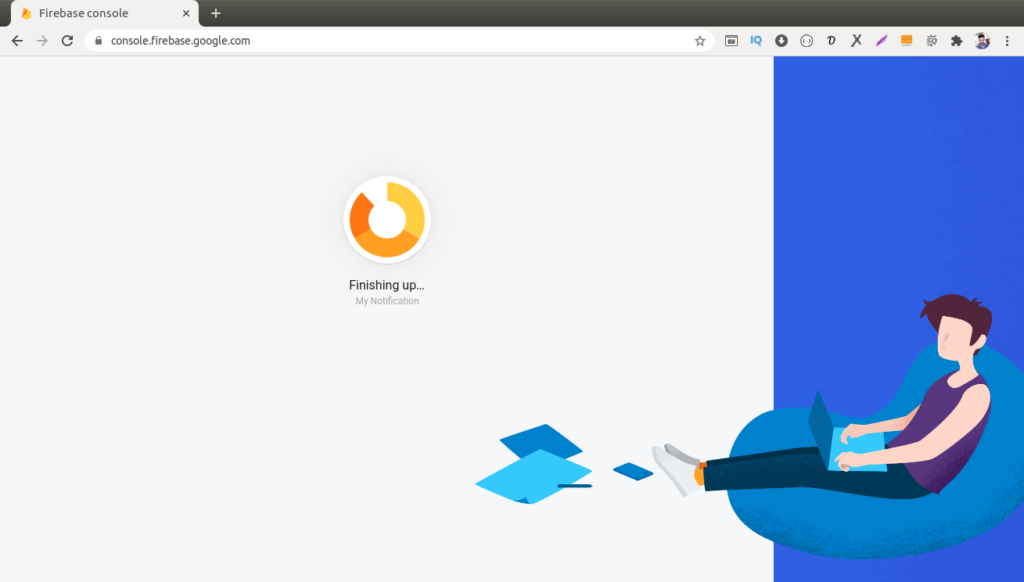
Back to Firebase Console dashboard panel. We can see we will have a setup icon for android as you can see in this given image. Simply click on that Icon and then the process of Setting up the registration of App will start.
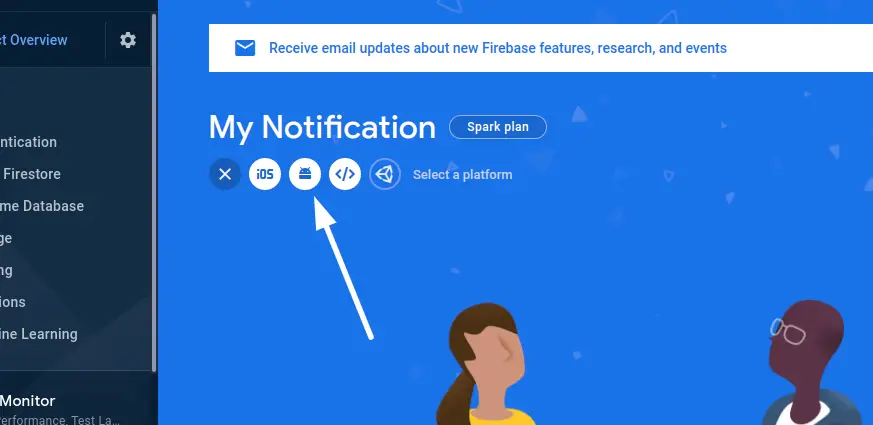
Register a Android App into Firebase Project.
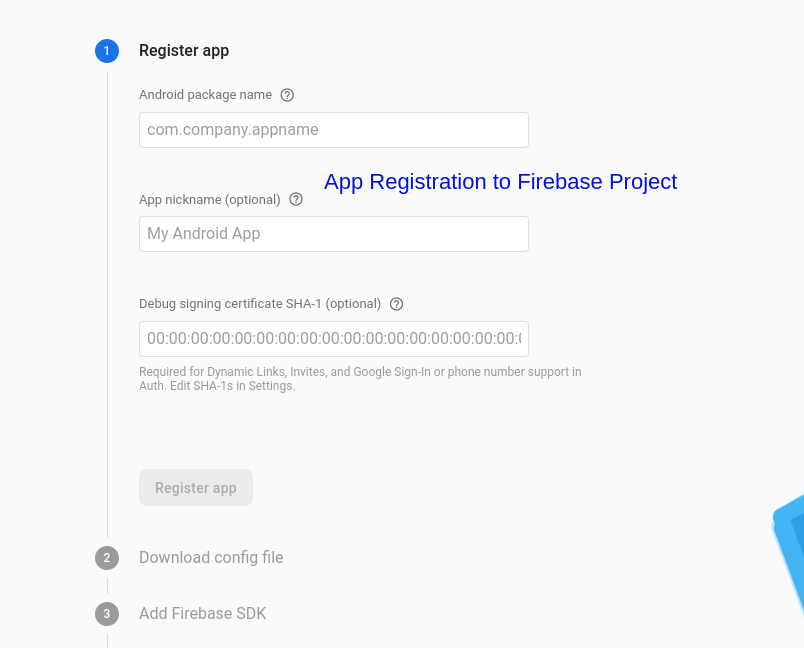
After creating App from here, back to firebase console. Go to App settings by click on gear icon and Click on Cloud messaging tab. You will find the Server key, we need that.
So copy server key.
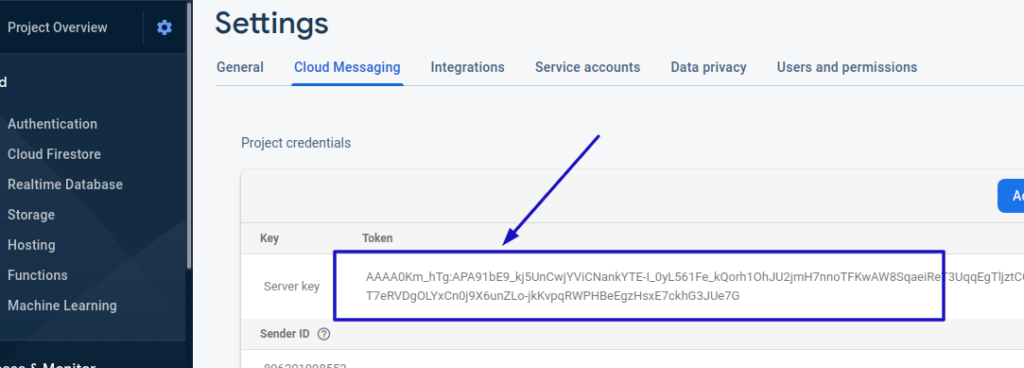
Successfully, we have now completed the whole process of Creating Firebase Project > Register Android App > Copy & Paste Server API Key.
Create API Route
We need to create a POST request api route which will be hit by android device. Open up the file /routes/api.php and copy the given code into and paste it.
//..Other routes Route::post('send-notification', [App\Http\Controllers\NotificationController::class, 'send']);
Create Controller & Add Method
Let’s create a notification controller into application. So to create need to run this artisan command.
$ php artisan make:controller NotificationController
After running this command, you will find a controller created at /app/Http/Controllers/NotificationController.php
Open up the file and paste the give code.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class NotificationController extends Controller { /** * Create a new controller instance. * * @return void */ public function send(Request $request) { return $this->sendNotification($request->device_token, array( "title" => "Sample Message", "body" => "This is Test message body" )); } /** * Write code on Method * * @return response() */ public function sendNotification($device_token, $message) { $SERVER_API_KEY = '<YOUR-SERVER-API-KEY>'; // payload data, it will vary according to requirement $data = [ "to" => $device_token, // for single device id "data" => $message ]; $dataString = json_encode($data); $headers = [ 'Authorization: key=' . $SERVER_API_KEY, 'Content-Type: application/json', ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://fcm.googleapis.com/fcm/send'); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_POSTFIELDS, $dataString); $response = curl_exec($ch); curl_close($ch); return $response; } }
The above code will send notification to a single device ID which we will pass into request.
Let’s say that we want to send notifications to multiple devices
So how will we send ?
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class NotificationController extends Controller { /** * Create a new controller instance. * * @return void */ public function send(Request $request) { return $this->sendNotification(array( $request->device_token1, $request->device_token2, $request->device_token3 //.. ), array( "title" => "Sample Message", "body" => "This is Test message body" )); } /** * Write code on Method * * @return response() */ public function sendNotification($device_tokens, $message) { $SERVER_API_KEY = '<YOUR-SERVER-API-KEY>'; // payload data, it will vary according to requirement $data = [ "registration_ids" => $device_tokens, // for multiple device ids "data" => $message ]; $dataString = json_encode($data); $headers = [ 'Authorization: key=' . $SERVER_API_KEY, 'Content-Type: application/json', ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://fcm.googleapis.com/fcm/send'); curl_setopt($ch, CURLOPT_POST, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_POSTFIELDS, $dataString); $response = curl_exec($ch); curl_close($ch); return $response; } }
When we send for a single device, we need to use
$data = [ "to" => $device_token, // for single device id "data" => $message ];
And for multiple devices, we have the key as registration_ids. If we want use this registration_ids to send only to a single device, it also works. Only we need to pass a single device token.
$data = [ "registration_ids" => $device_tokens, // for multiple device ids "data" => $message ];
We hope this article helped you to learn about Laravel 8 Send Push Notification to Android Using Firebase in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.
Hi,
Can you kindly tell me that the device id used here is the device id or the FCM token of firebase?
Thanks
Hi, it’s a device token value. To send notification you only needs these two –
1. Device ID or you can device ID
2. Server API key of firebase