In most applications we need sometime a schedule calendar so that we can add our tasks, events, functions. Those scheduled tasks act like a reminder to the calendar. This feature is very interesting to implement if you are providing adding events to your clients, users, etc.
Inside this article we will see the complete concept of laravel 8 fullcalendar ajax crud tutorial. This article is super easy to learn and implement in your code as well.
FullCalendar is a jquery plugin by the help of which we can display a calendar to webpage. Even we can create events, appointments etc on a specific date, update existing data, delete data etc. All CRUD operations we can easily manage from it’s interface.
This is not laravel 8 specific feature, you can use it in any version of laravel.
Let’s get started.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Model & Migration
We need very first a Model and a migration file.
Open project into terminal and run this artisan command.
$ php artisan make:model Event -m
It will create a model i.e Event.php at /app/Models folder as well as a migration file 2021_05_01_073927_create_events_table.php at /database/migrations.
Open Event.php and write this complete code into it.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Event extends Model { use HasFactory; protected $fillable = [ 'title', 'start', 'end' ]; }
Open Migration file and write this complete code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateEventsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('events', function (Blueprint $table) { $table->id(); $table->string('title'); $table->date('start'); $table->date('end'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('events'); } }
Migration to Migrate
Next, we need to migrate the migration what we have created.
$ php artisan migrate
This command will creates a table events in your database.
Create Controller
We need now a controller file. Back to terminal and run this artisan command to create.
$ php artisan make:controller FullCalendarController
This will creates a file FullCalendarController.php at /app/Http/Controllers folder.
Open FullCalendarController.php and write this complete code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Event; class FullCalenderController extends Controller { /** * Write code on Method * * @return response() */ public function index(Request $request) { // on page load this ajax code block will be run if ($request->ajax()) { $data = Event::whereDate('start', '>=', $request->start) ->whereDate('end', '<=', $request->end) ->get(['id', 'title', 'start', 'end']); return response()->json($data); } return view('fullcalendar'); } /** * This method is to handle event ajax operation * * @return response() */ public function ajax(Request $request) { switch ($request->type) { // For add event case 'add': $event = Event::create([ 'title' => $request->title, 'start' => $request->start, 'end' => $request->end, ]); return response()->json($event); break; // For update event case 'update': $event = Event::find($request->id)->update([ 'title' => $request->title, 'start' => $request->start, 'end' => $request->end, ]); return response()->json($event); break; // For delete event case 'delete': $event = Event::find($request->id)->delete(); return response()->json($event); break; default: break; } } }
Create Blade Layout File
Next, create a blade layout file with the name fullcalendar.blade.php inside /resources/views folder.
Open fullcalendar.blade.php and write this complete code into it.
<!DOCTYPE html> <html> <head> <title>Laravel 8 Fullcalender Tutorial - Online Web Tutor</title> <meta name="csrf-token" content="{{ csrf_token() }}"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script> <!--fullcalendar plugin files --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/fullcalendar/3.9.0/fullcalendar.css" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.24.0/moment.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/fullcalendar/3.9.0/fullcalendar.js"></script> <!-- for plugin notification --> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.css" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.js"></script> </head> <body> <div class="container"> <h3 style="text-align: center">Laravel 8 Fullcalendar Tutorial - Online Web Tutor</h3> <div id="calendar"></div> </div> <script> var site_url = "{{ url('/') }}"; </script> <script src="{{ URL::asset('script.js') }}"></script> </body> </html>
- {{ URL::asset(‘script.js’) }} Add script.js file from /public folder.
- var site_url = “{{ url(‘/’) }}”; Declare site url to use it in script.js file.
Create Javascript File – to Handle Ajax request
Create a file script.js inside /public folder. This file will contain all ajax handling code.
Open script.js file and write this complete code into it.
$(document).ready(function() { $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); var calendar = $('#calendar').fullCalendar({ editable: true, events: site_url + "/event", displayEventTime: false, editable: true, eventRender: function(event, element, view) { if (event.allDay === 'true') { event.allDay = true; } else { event.allDay = false; } }, selectable: true, selectHelper: true, select: function(start, end, allDay) { var title = prompt('Event Title:'); if (title) { var start = $.fullCalendar.formatDate(start, "Y-MM-DD"); var end = $.fullCalendar.formatDate(end, "Y-MM-DD"); $.ajax({ url: site_url + "/eventAjax", data: { title: title, start: start, end: end, type: 'add' }, type: "POST", success: function(data) { displayMessage("Event Created Successfully"); calendar.fullCalendar('renderEvent', { id: data.id, title: title, start: start, end: end, allDay: allDay }, true); calendar.fullCalendar('unselect'); } }); } }, eventDrop: function(event, delta) { var start = $.fullCalendar.formatDate(event.start, "Y-MM-DD"); var end = $.fullCalendar.formatDate(event.end, "Y-MM-DD"); $.ajax({ url: site_url + '/eventAjax', data: { title: event.title, start: start, end: end, id: event.id, type: 'update' }, type: "POST", success: function(response) { displayMessage("Event Updated Successfully"); } }); }, eventClick: function(event) { var deleteMsg = confirm("Do you really want to delete?"); if (deleteMsg) { $.ajax({ type: "POST", url: site_url + '/eventAjax', data: { id: event.id, type: 'delete' }, success: function(response) { calendar.fullCalendar('removeEvents', event.id); displayMessage("Event Deleted Successfully"); } }); } } }); }); function displayMessage(message) { toastr.success(message, 'Event'); }
Create Routes
Add these routes into web.php at /routes folder.
Open web.php file,
# Add this to header use App\Http\Controllers\FullCalenderController; //... Route::get('event', [FullCalenderController::class, 'index']); Route::post('eventAjax', [FullCalenderController::class, 'ajax']);
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL – http://127.0.0.1:8000/event
Before adding any event by the fullcalendar.
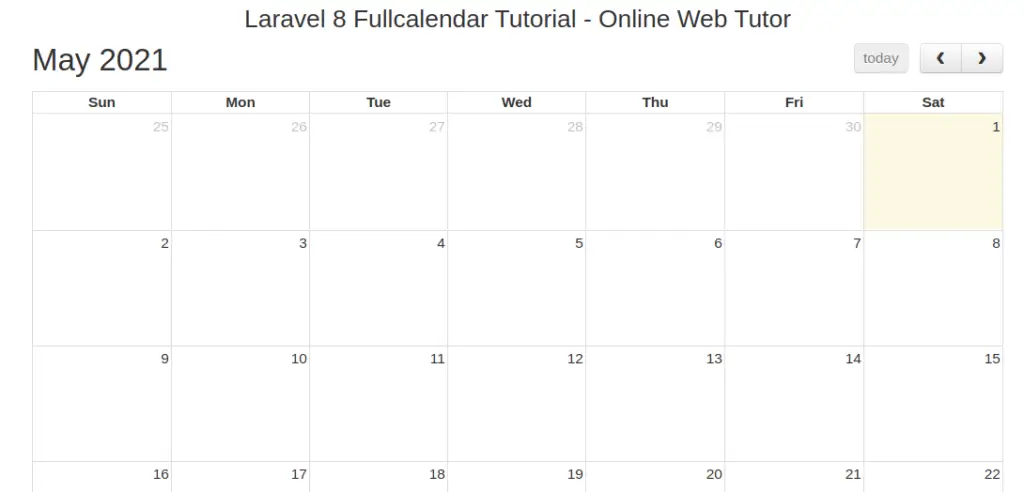
Add Event
To add event, simply click on any specific date and add your event via javascript popup box. when you fill Event title and hit Ok button. By ajax it will save data into database table.
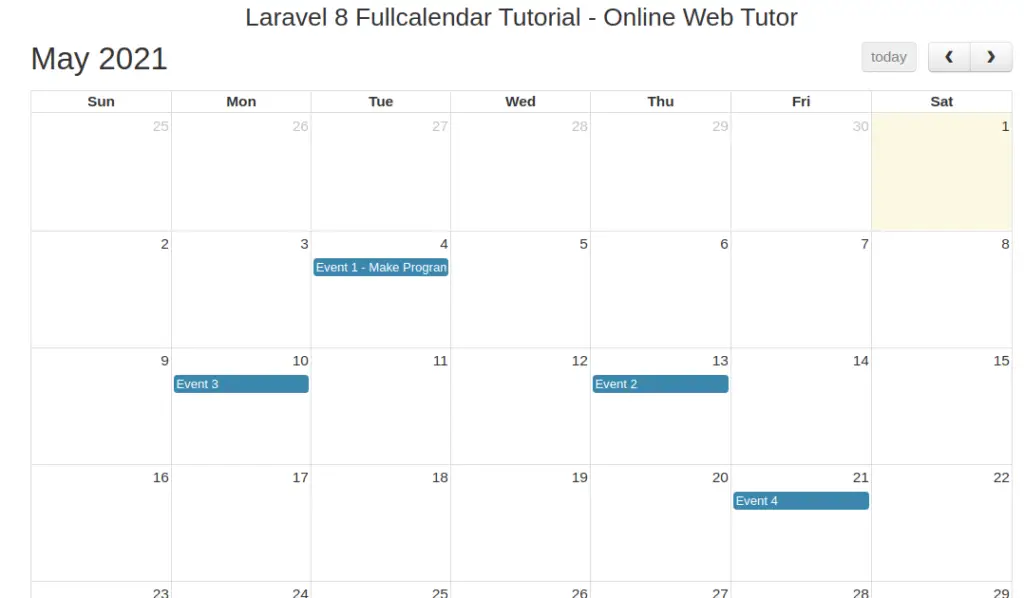
Update event
Simply drag and drop your events here and there inside calendar. It will update the event where you drop by ajax handler.
Delete Event
When you click on any event of calendar, it will open a javascript confirmation box, if Yes then it will delete that event by ajax handler.
Events inside database
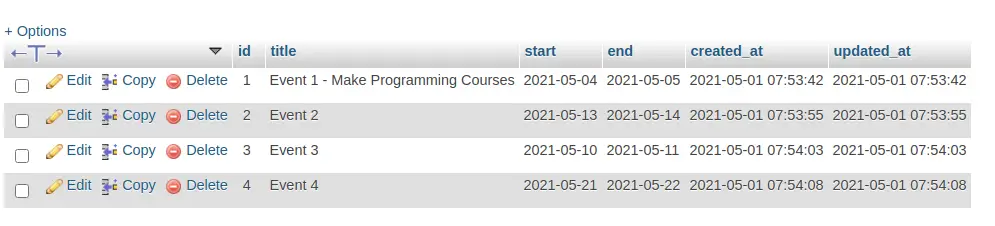
We hope this article helped you to learn about Laravel 8 FullCalendar Ajax CRUD Tutorial Example Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.