Inside this article we will see the concept i.e Laravel 9 How to Get Browser and Platform Details Tutorial. Article contains the classified information about how to get browser name, system details and device details in laravel.
If you are looking for a solution i.e laravel jenssegers/agent in Laravel 9 to get browser, system details, device details, etc then this article will help you a lot for this. Tutorial is super easy to understand and implement it in your code as well.
We will use jenssegers/agent composer package to get browser name and version in laravel application. jenssegers/agent will help to get browser name, browser version, device name, and platform name, check if a device is mobile, desktop, or tablet, and check whether the user is a robot or not.
Learn More –
- Laravel 9 Create Blade View File Using Artisan Command
- How to Convert JSON to Array in Laravel 9 Example Tutorial
- How To Use Carbon in Laravel 9 Blade or Controller Tutorial
- Laravel 9 Autocomplete Places Search Box Using Google Maps JavaScript API
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Install Composer Package (jenssegers/agent)
A PHP package desktop/mobile user agent parser with support for Laravel, based on Mobile Detect with desktop support and additional functionality.
Open project into terminal and run this command to install it.
$ composer require jenssegers/agent
It will install above package into your application.
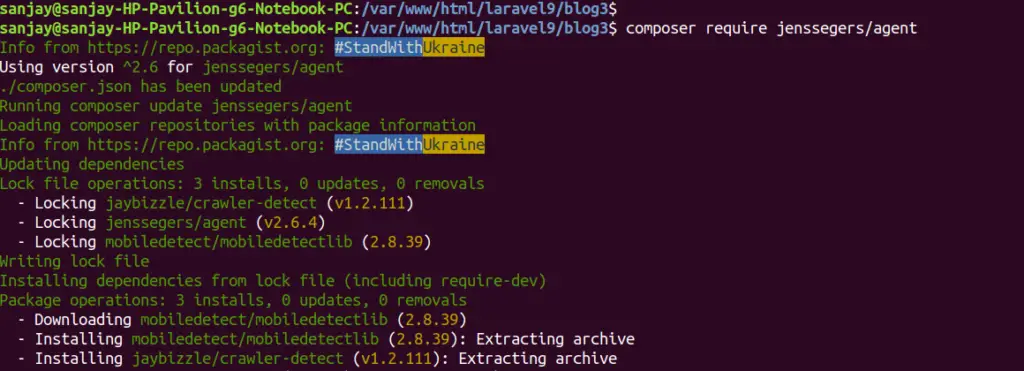
Next,
Let’s use this package to detect device details, browser related information, etc.
Example #1: Laravel Get Browser Name and Version
Open project into terminal and run this command to create controller file.
$ php artisan make:controller DemoController
It will create a file DemoController.php inside /app/Http/Controllers folder.
To get browser data.
Open this controller file and write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Jenssegers\Agent\Facades\Agent; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $browser = Agent::browser(); $version = Agent::version($browser); dd($browser, $version); } }
Output
Chrome
105.0.0.0
Example #2: Laravel Get Device Name
To get device name.
Open DemoController.php file from /app/Http/Controllers folder. Write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Jenssegers\Agent\Facades\Agent; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $device = Agent::device(); dd($device); } }
Output
WebKit
Example #3: Laravel Get Platform Name
To get platform detail.
Open DemoController.php file from /app/Http/Controllers folder. Write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Jenssegers\Agent\Facades\Agent; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $platform = Agent::platform(); dd($platform); } }
Output
Linux
Example #4: Laravel Check Device is Desktop, Tablet or Phone
To check device type.
Open DemoController.php file from /app/Http/Controllers folder. Write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Jenssegers\Agent\Facades\Agent; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index() { if (Agent::isMobile()) { $result = 'Yes, This is Mobile.'; } else if (Agent::isDesktop()) { $result = 'Yes, This is Desktop.'; } else if (Agent::isTablet()) { $result = 'Yes, This is Desktop.'; } else if (Agent::isPhone()) { $result = 'Yes, This is Phone.'; } dd($result); } }
Output
Yes, This is Desktop.
Example #5: Laravel User is Robot or Not
To check laravel user is robot or not.
Open DemoController.php file from /app/Http/Controllers folder. Write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Jenssegers\Agent\Facades\Agent; class DemoController extends Controller { /** * Write code on Method * * @return response() */ public function index() { if (Agent::isRobot()) { dd('Yes, User is Robot.'); } dd("User is real!"); } }
Output
User is real!
We hope this article helped you to learn Laravel 9 How to Get Browser and Platform Details Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.