Pagination plays a crucial role in API development by efficiently managing and delivering data in smaller, digestible chunks, enhancing performance and user experience. In Laravel 10, paginating API resources allows developers to break down large data sets into paginated results, optimizing API response handling.
In this tutorial, we’ll see the comprehensive process of paginating API resources in Laravel 10. This functionality empowers developers to organize and present API data in manageable segments, improving performance and navigability for API consumers.
Read More: How To Use Laravel 10 Queue To Send Emails Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Seed Test Users in Database
Here, we will create test users using data seeding concept. Please make sure your application should be connected with application and also default tables must be migrated.
Open project into terminal, run this command
php artisan tinker
It will open a shell where you can run this command to generate test users,
> App\Models\User::factory(100)->create()
Above command will generate 100 fake users and insert into users table.
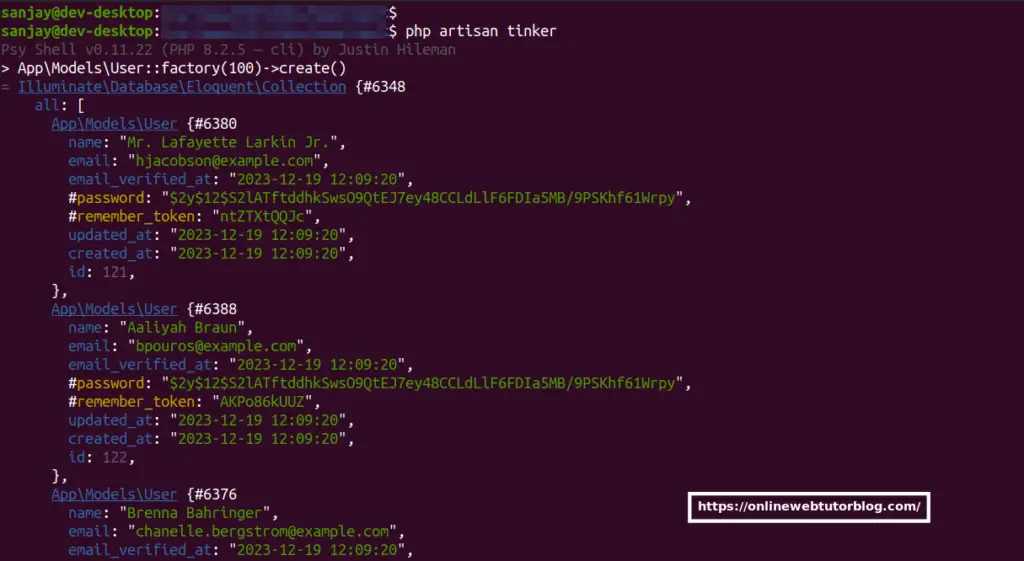
Read More: Build WordPress User Login Using Laravel Corcel Tutorial
Create API Resource Class
Open project terminal and run this command,
php artisan make:resource UserResource
It will create a resource class with name UserResource.php inside app/Http/Resources folder.
Source code of this class file will be like this,
<?php namespace App\Http\Resources; use Illuminate\Http\Request; use Illuminate\Http\Resources\Json\JsonResource; class UserResource extends JsonResource { /** * Transform the resource into an array. * * @return array<string, mixed> */ public function toArray(Request $request): array { return parent::toArray($request); } }
Setup API Controller Class
Open project terminal and run this command,
php artisan make:controller UserController
It will create a resource class with name UserResource.php inside app/Http/Resources folder.
Open file and write this code into it,
<?php namespace App\Http\Controllers; use App\Http\Resources\UserResource; use App\Models\User; use Illuminate\Http\Request; class UserController extends Controller { public function index() { $users = User::paginate(10); return UserResource::collection($users); } }
Read More: Laravel with Gupshup API Send WhatsApp Message
Add API Route
Open web.php file from /routes folder. Add this route and code into it.
//... use App\Http\Controllers\UserController; Route::get("user-data", [UserController::class, "index"]); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/user-data
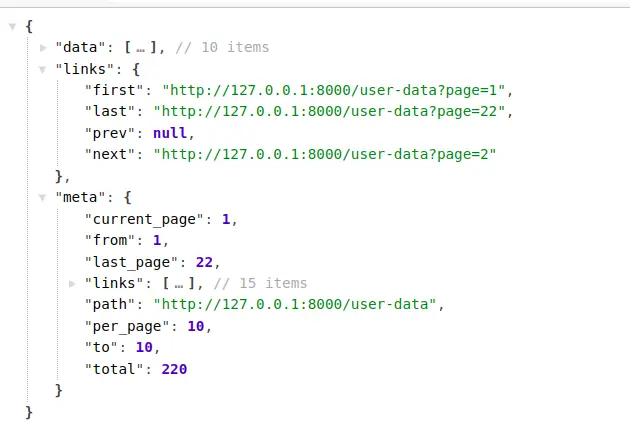
That’s it.
We hope this article helped you to learn about How to Paginate Laravel 10 API Resources Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.