In CodeIgniter 4, we have amazing spark CLI features. By the help of php spark we can manage everything like controller, model, migration, seeder, custom command etc.
To work with controllers, we use in make:controller from spark commands. Inside this article we will see the concept of RESTful Resource Controller in CodeIgniter 4. Step by step we will discuss about it’s creation and managing resource routes of application.
Resource Controller and their routes is very useful to get all routes which can manage a complete application. Routes include create, store, list, update, destroy etc. Inside this article body we are going to see each interesting things in a very detailed concept.
Learn More –
- Complete Concept of Named Route in CodeIgniter 4 Tutorial
- Concept of Date And Time in CodeIgniter 4 Tutorial
- Concept of Inflector Helper in CodeIgniter 4 Tutorial
- Concept of Migration in CodeIgniter 4 Tutorial
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Create Controller
Controllers are those files which manage or controls the application flow by binding user layouts and database data together.
In keyword MVC, C stands for Controller.
Creating a controller by using spark –
$ php spark make:controller Device
This above command will create a controller file at /app/Controllers/Device.php. Here is the default skeleton of normal controller file.
<?php namespace App\Controllers; use App\Controllers\BaseController; class Device extends BaseController { public function index() { // } }
Create Route
Open /app/Config/Routes.php file to manage application routes. Here, application has a normal route.
$routes->get('/', 'Home::index');
The added routes means –
- / – URLRoute
- Home::index – index() method of Home controller
What is a Resource Controller in CodeIgnietr 4 ?
The ResourceController provides a convenient starting point for your RESTful API, with methods that correspond to the resource routes. CodeIgniter makes development of RESTful APIs very easy for resources with the use of resource routes and ResourceController.
Open help manual of spark make:controller
$ php spark help make:controller
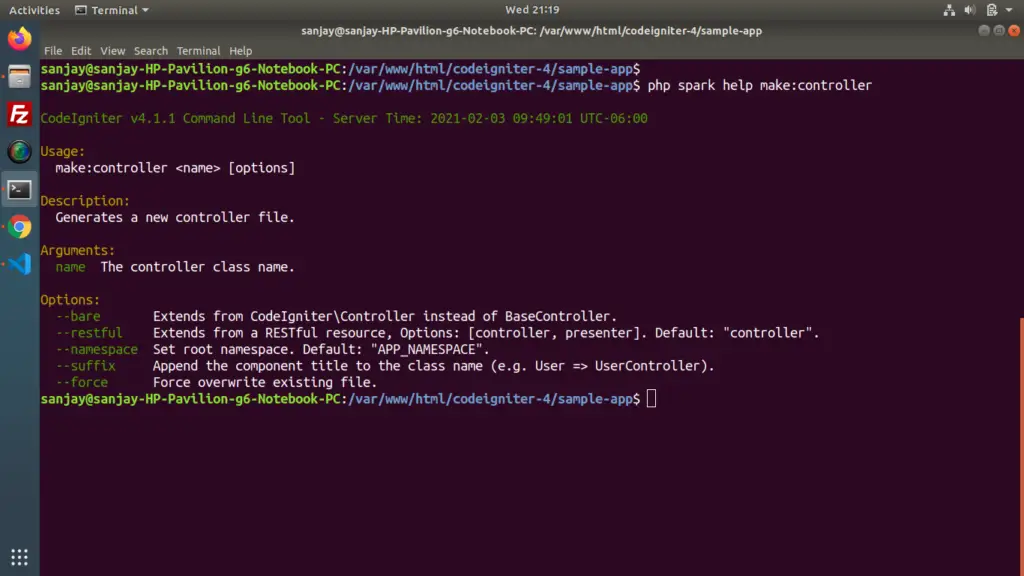
Inside this above help manual, we can see a option called –restful, description is Extends from a RESTful resource, Options: [controller, presenter]. Default: “controller”.
So to create a restful resource controller –
$ php spark make:controller Employees --restful
This command will create a controller with the name Employees inside /app/Controllers
Open Employees.php file
<?php namespace App\Controllers; use CodeIgniter\RESTful\ResourceController; class Employees extends ResourceController { /** * Return an array of resource objects, themselves in array format * * @return mixed */ public function index() { // } /** * Return the properties of a resource object * * @return mixed */ public function show($id = null) { // } /** * Return a new resource object, with default properties * * @return mixed */ public function new() { // } /** * Create a new resource object, from "posted" parameters * * @return mixed */ public function create() { // } /** * Return the editable properties of a resource object * * @return mixed */ public function edit($id = null) { // } /** * Add or update a model resource, from "posted" properties * * @return mixed */ public function update($id = null) { // } /** * Delete the designated resource object from the model * * @return mixed */ public function delete($id = null) { // } }
Here, this is the default skeleton of creating a resource controller in CodeIgniter 4. Each method inside this file is used for handling different different functions.
All methods together handle a complete CRUD application.
Resource Routes
To configure resource routes we need to use resource() method, open Routes.php file. Add this code.
$routes->resource("employees");
This added line will generate all routes for managing resources. It returns a group of routes of all types of request.
Behind the scene the above written line returns these routes
$routes->get('employees/new', 'Employees::new');
$routes->post('employees', 'Employees::create');
$routes->get('employees', 'Employees::index');
$routes->get('employees/(:segment)', 'Employees::show/$1');
$routes->get('employees/(:segment)/edit', 'Employees::edit/$1');
$routes->put('employees/(:segment)', 'Employees::update/$1');
$routes->patch('employees/(:segment)', 'Employees::update/$1');
$routes->delete('employees/(:segment)', 'Employees::delete/$1');
Open project in terminal after adding resource route line into Routes.php.
$ php spark routes
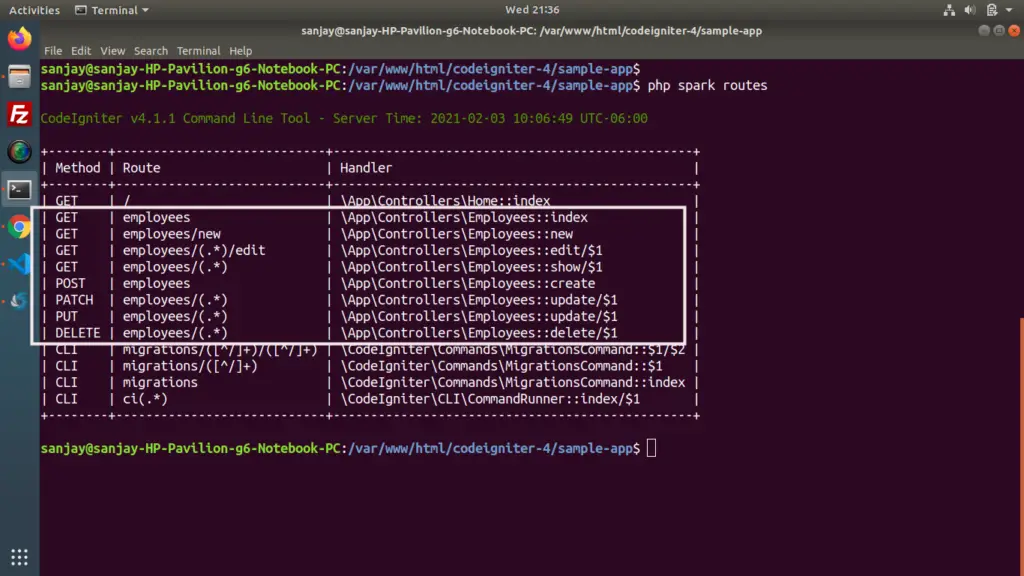
Here we have all routes. For example let’s pick a random one from the list
\App\Controllers\Employees::create
This line means create() method of Employees controller. \App\Controllers is the namespace of Employee controller.
Remove Specific Resource Routes
As above we can see, we have the list of routes generated once we add a resource line into Routes.php file.
Let’s say we need only few routes and their methods into the list. For example application uses index(), create(), show(), update() only.
Now how can we fix these methods only in resource routes.
$routes->resource('employees', ['only' => ['index', 'create', 'show', 'update']]);
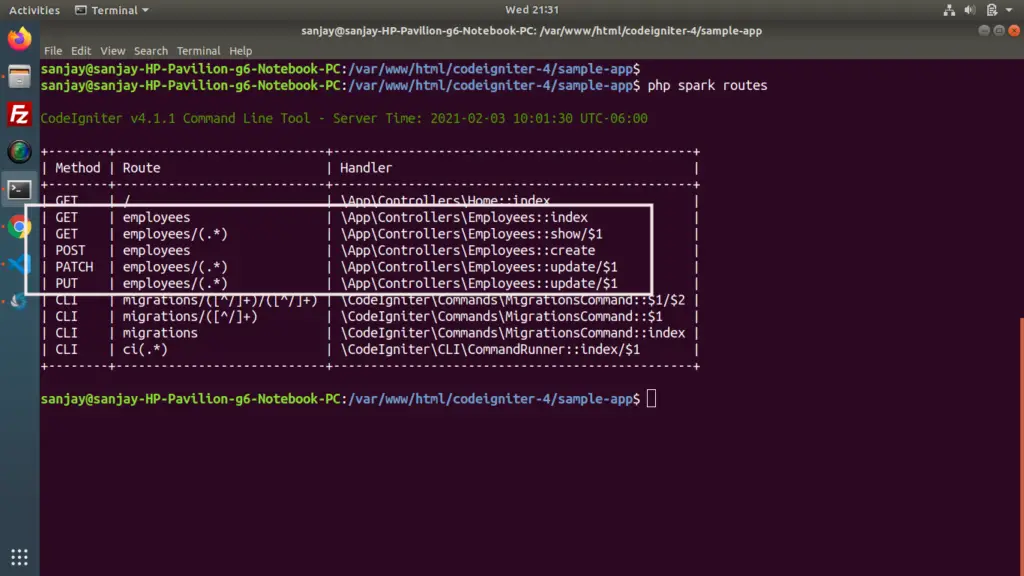
Successfully we have fixed that.
In some cases we need to remove only create() method and want rest all in the list. So here instead of writing all methods in the list, we use the concept of except
$routes->resource('employees', ['except' => ['create']]);
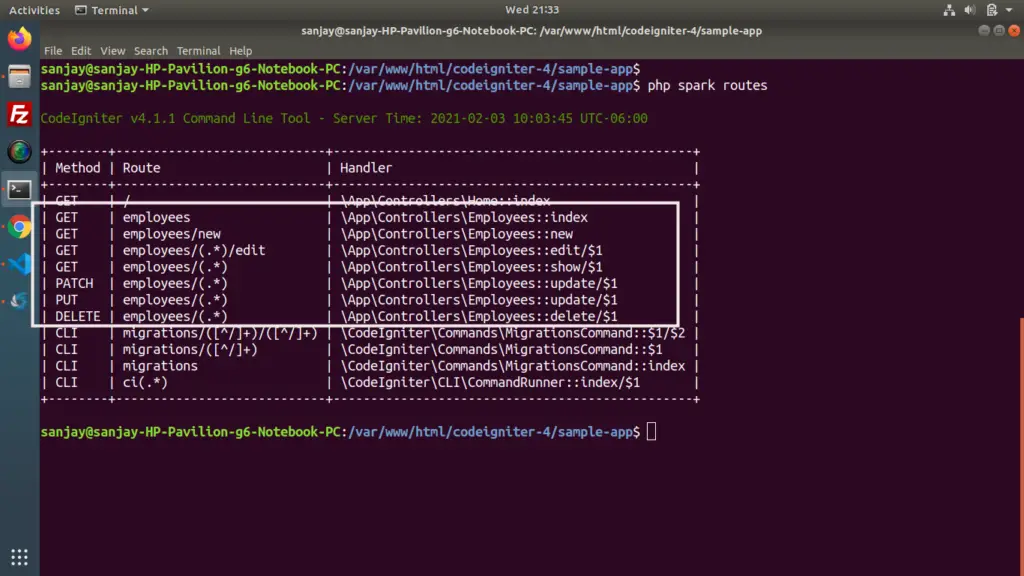
We hope this article helped you to learn about RESTful Resource Controller in CodeIgniter 4 Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.
Thanks Sanjay, you save my time!
Great! It helped