In every web application, somewhere we need data table which contains data into a list format. Converting a list into a data table by means of two ways i.e Client side using jQuery datatable plugin and Server side using Pagination Library in Laravel 8.
DataTable gives a perfect view for data listing to end users.
Inside this article, we will discuss step by step guide to laravel 8 pagination tutorial. Very simple few steps we need to follow to integrate pagination into a laravel project.
Let’s get started.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Migration
To create a table in database, we will use migration command of laravel artisan to create schema. Open project into terminal and run this artisan command.
$ php artisan make:migration CreateProductsTable
It will create a migration file with name 2021_03_15_030914_create_products_table.php according to my timestamp values at location /database/migrations.
Open migration file 2021_03_15_030914_create_products_table.php and write this code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateProductsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('products', function (Blueprint $table) { $table->id(); $table->string("name", 70); $table->string("slug", 100); $table->text("description"); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('products'); } }
Migration Migration
To run migration file and create table.
$ php artisan migrate
Create Model
To create model, run this artisan command.
$ php artisan make:model Product
It will create a file with name Product.php at location /app/Models.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Product extends Model { use HasFactory; public $timestamps = false; }
Create Factory File – Data Seed
To seed dummy data into table, we need a factory file. To create factory file we will use artisan command. Back to terminal and run this artisan command.
$ php artisan make:factory ProductFactory --model=Product
It will create a file with name ProductFactory.php at location /database/factories.
Open ProductFactory.php and write this code into it.
<?php namespace Database\Factories; use App\Models\Product; use Illuminate\Database\Eloquent\Factories\Factory; use Illuminate\Support\Str; class ProductFactory extends Factory { /** * The name of the factory's corresponding model. * * @var string */ protected $model = Product::class; /** * Define the model's default state. * * @return array */ public function definition() { return [ 'name' => $this->faker->name, 'slug' => Str::slug($this->faker->name), 'description' => $this->faker->text, ]; } }
Run Factory File
To run this factory which results, it creates dummy data into database table. We will run this factory file by Tinker Shell panel
$ php artisan tinker
It will open a shell interface, by the help of which we will interact with database.
>>> App\Models\Product::factory()->count(500)->create()

This command will create 500 dummy data to database table.
Create Route
Open web.php file from /routes folder. Add these lines into it.
# At header use App\Http\Controllers\ProductController; # Route Route::get('products', [ProductController::class, 'index']);
Create Controller File
Open project into terminal and run this artisan command to create controller
$ php artisan make:controller ProductController
It will create ProductController.php at /app/Http/Controllers folder. Open file and write this following code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; class ProductController extends Controller { public function index() { $data = Product::paginate(10); return view('products', compact('data')); } }
Create View Layout
Create a file products.blade.php at /resources/views folder. In this file, we will write the code to list data.
Open products.blade.php,
<!DOCTYPE html> <html> <head> <title>Laravel 8 Pagination Tutorial - Online Web Tutor</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> </head> <body> <div class="container"> <h3 style="text-align: center;">Laravel 8 Pagination Tutorial - Online Web Tutor</h3> <div class="panel panel-primary"> <div class="panel-heading">Laravel 8 Pagination Tutorial - Online Web Tutor</div> <div class="panel-body"> <table class="table"> <thead> <tr> <th>#ID</th> <th>#Name</th> <th>#Slug</th> <th>#Action</th> </tr> </thead> <tbody> @if (!empty($data) && $data->count()) @foreach ($data as $key => $value) <tr> <td>{{ $value->id }}</td> <td>{{ $value->name }}</td> <td>{{ $value->slug }}</td> <td> <button class="btn btn-danger">Delete</button> </td> </tr> @endforeach @else <tr> <td colspan="10">There are no data.</td> </tr> @endif </tbody> </table> {!! $data->links() !!} </div> </div> </div> </body> </html>
Link Paginator To Use Bootstrap
Open AppServiceProvider.php from /app/Providers folder. Inside this file, we will have two methods i.e register() and boot(). In boot() method, we will enable bootstrap to use by paginator links.
Open AppServiceProvider.php and update this code.
<?php namespace App\Providers; use Illuminate\Support\ServiceProvider; use Illuminate\Pagination\Paginator; class AppServiceProvider extends ServiceProvider { /** * Register any application services. * * @return void */ public function register() { // } /** * Bootstrap any application services. * * @return void */ public function boot() { Paginator::useBootstrap(); // here we have added code. } }
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/products
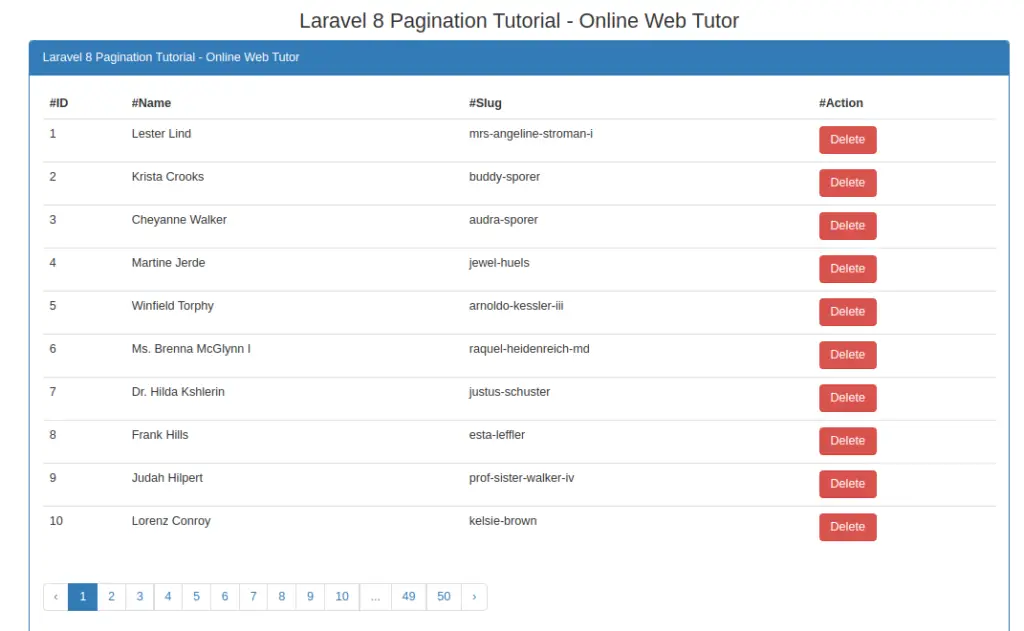
We hope this article helped you to learn about Step By Step Laravel 8 Pagination Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.