Inside this article we will see the concept of validating maximum 10 numbers entry in textbox using jquery. Article contains classified information about the number validation.
If you are looking for an article which helps you to understand how to validate maximum of 10 numbers in textbox using jquery then you are at right place to learn.
There are several options available by using jquery/javascript which do maximum input validation.
Learn More –
- How To Create Signature Pad Using jQuery Plugin
- How To Generate QR Code Using Javascript
- How To Generate QR Code Using Javascript Library
- How to Generate Random String in jQuery / Javascript
Let’s get started.
Create an Application
Example #1
Create a folder with name max-numbers. Create a file index.html into it.
Open index.html and write this complete code into it.
<!DOCTYPE html> <html lang="en"> <head> <title>Allow only 10 Numbers in Textbox Using jQuery</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> </head> <body> <div class="container"> <h4>Allow only 10 Numbers in Textbox Using jQuery</h4> <div class="panel panel-primary"> <div class="panel-heading">Allow only 10 Numbers in Textbox Using jQuery</div> <div class="panel-body"> <form action="#" method="post"> <div class="form-group"> <label for="email">Phone Number:</label> <input type="text" name="phone" class="form-control" placeholder="Phone Number..." pattern="[1-9]{1}[0-9]{9}"> </div> <button type="submit" class="btn btn-success">Submit</button> </form> </div> </div> </div> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> </body> </html>
Concept
Pattern attribute of input type text element.
pattern="[1-9]{1}[0-9]{9}"
Application Testing
Open browser and type this –
URL – http://localhost/max-numbers/index.html
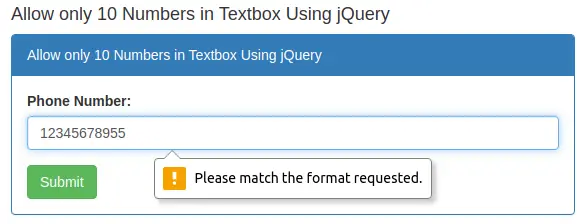
Example #2
<!DOCTYPE html> <html lang="en"> <head> <title>Allow only 10 Numbers in Textbox Using jQuery</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> </head> <body> <div class="container"> <h4>Allow only 10 Numbers in Textbox Using jQuery</h4> <div class="panel panel-primary"> <div class="panel-heading">Allow only 10 Numbers in Textbox Using jQuery</div> <div class="panel-body"> <form action="#" method="post"> <div class="form-group"> <label for="email">Phone Number:</label> <input type="text" name="phone" class="form-control phone" maxlength="10" placeholder="Phone Number..."> </div> <button type="submit" class="btn btn-success">Submit</button> </form> </div> </div> </div> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script type="text/javascript"> $('.phone').keypress(function(e) { var arr = []; var kk = e.which; for (i = 48; i < 58; i++) arr.push(i); if (!(arr.indexOf(kk) >= 0)) e.preventDefault(); }); </script> </body> </html>
Concept
Maxlength attribute of input element and script code to restrict maximum allowed length.
$('.phone').keypress(function(e) { var arr = []; var kk = e.which; for (i = 48; i < 58; i++) arr.push(i); if (!(arr.indexOf(kk) >= 0)) e.preventDefault(); });
Application Testing
Open browser and type this –
URL – http://localhost/max-numbers/index.html
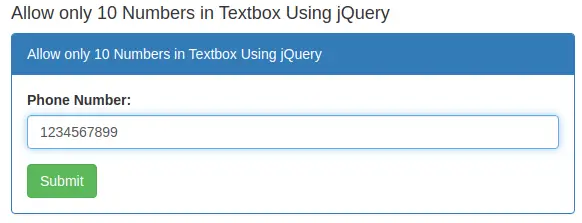
We hope this article helped you to learn Validate Maximum of 10 Numbers in Textbox Using jQuery Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.