Inside this article we will see the use of merge() and all() methods in laravel 9 collections. Article contains a very classified information about the basic concept of How to merge Eloquents in laravel 9 collection.
We will merge collections in laravel and also will see how to merge laravel eloquents.
The <strong>Illuminate\Support\Collection</strong>
class provides a fluent, convenient wrapper for working with arrays of data. For example, check out the following code. We’ll use the <strong>collect</strong>
helper to create a new collection instance from the array.
As mentioned above, the collect
helper returns a new <strong>Illuminate\Support\Collection</strong>
instance for the given array.
So, creating a collection is as simple as:
$collection = collect([1, 2, 3]);
Learn More –
- Laravel 9 Authentication with Laravel UI Tutorial
- Laravel 9 Bootstrap Growl jQuery Notification Plugin
- Laravel 9 Call MySQL Stored Procedure Tutorial
- Laravel 9 Clear Cache of Application Route, View & Config
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Method #1 Use merge() Method
We will see how to use merge() into collection.
Suppose we have SiteController.php a controller file inside /app/Http/Controllers folder.
Code Example #1 – Merge Collection with Values
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class SiteController extends Controller { public function index() { $collection1 = collect([1, 2, 3]); $collection2 = collect([4, 5]); $mergedCollection = $collection1->merge($collection2); $mergedCollection->all(); dd($mergedCollection); } }
Concept
$mergedCollection = $collection1->merge($collection2);
$mergedCollection->all();
Output
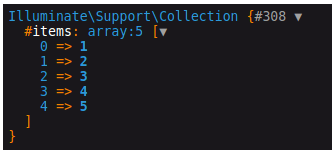
Code Example #2 – Merge Collection with Unique Values
Here,
Suppose we have two collections, but few elements of collections are duplicate. Now, let’s see how to merge unique values only.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class SiteController extends Controller { public function index() { $collection1 = collect([1, 2, 3, 4]); $collection2 = collect([4, 5, 5, 6, 7]); $mergedCollection = $collection1->merge($collection2); $mergedCollection = $mergedCollection->unique(function ($item) { return $item; }); $mergedCollection->all(); dd($mergedCollection); } }
Concept
$mergedCollection = $mergedCollection->unique(function ($item) {
return $item;
});
Output
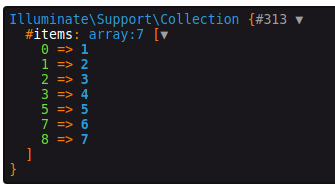
Method #2 Merge Eloquent Collection
In this example we will see how to merge laravel eloquents.
Let’s say we have two models – Product & Seller
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Product; use App\Models\Seller; class SiteController extends Controller { public function index() { $collection1 = Product::get(); $collection2 = Seller::get(); $mergedCollection = $collection1->merge($collection2); $mergedCollection->all(); dd($mergedCollection); } }
We hope this article helped you to How to Merge Eloquents in Laravel 9 Collection Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.