Inside this article we will see the concept of password validation on keyup jquery event without using any plugin.
There are many plugins which provides full form validation with error messages as well. But inside this tutorial we will see How to validate password and confirm password using jQuery without any jquery plugin. We will add password check validation on keyup event.
Learn More –
- Javascript Auto Logout in CodeIgniter 4 Tutorial
- jQuery Datatables Header Misaligned With Vertical Scrolling
- SweetAlert2 jQuery Notification Plugin to Laravel 8
- Toastr jQuery Notification Plugin to Laravel 8
- Upload And Convert Excel File into JSON in Javascript
Let’s get started.
Create an Application
Create a folder with name password-validate. Create a file index.html into it.
Open index.html and write this complete code into it.
<html> <head> <meta charset="utf-8"> <title>How To Validate Password And Confirm Password Using jQuery</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> </head> <body> <div class="container"> <h3>How To Validate Password And Confirm Password Using jQuery</h3> <div class="panel panel-primary"> <div class="panel-heading">How To Validate Password And Confirm Password Using jQuery</div> <div class="panel-body"> <form action="#"> <div class="form-group"> <label for="pwd">Password:</label> <input type="password" required class="form-control" id="password" placeholder="Enter a password"> </div> <div class="form-group"> <label for="pwd">Confirm Password:</label> <input type="password" required class="form-control" id="confirm_password" placeholder="Enter a Confirm Password"> </div> <div style="margin-top: 7px;" id="CheckPasswordMatch"></div> <br> <button type="submit" class="btn btn-primary">Submit</button> </form> </div> </div> </div> <script> $(document).ready(function() { $("#confirm_password").on('keyup', function() { var password = $("#password").val(); var confirmPassword = $("#confirm_password").val(); if (password != confirmPassword) $("#CheckPasswordMatch").html("Password does not match !").css("color", "red"); else $("#CheckPasswordMatch").html("Password match !").css("color", "green"); }); }); </script> <body> </html>
Concept is for jQuery is –
$("#confirm_password").on('keyup', function() { var password = $("#password").val(); var confirmPassword = $("#confirm_password").val(); if (password != confirmPassword) $("#CheckPasswordMatch").html("Password does not match !").css("color", "red"); else $("#CheckPasswordMatch").html("Password match !").css("color", "green"); });
Application Testing
Open browser and type this –
URL – http://localhost/password-validate/index.html
Enter wrong password
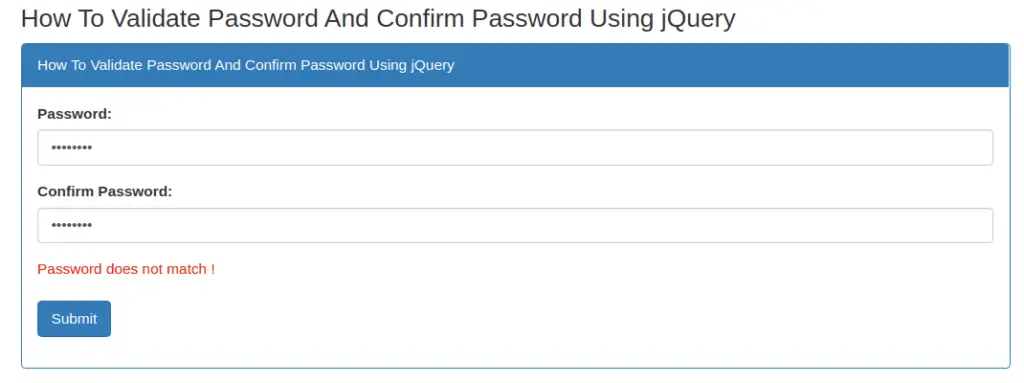
When enter right password
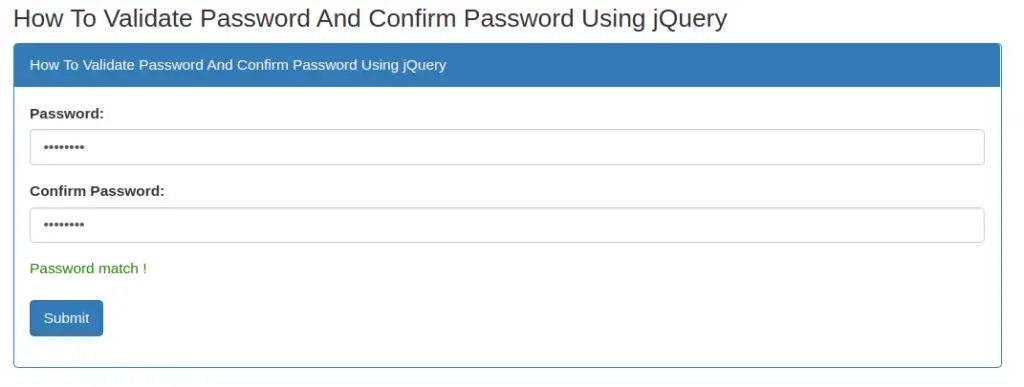
We hope this article helped you to learn Validate Password And Confirm Password Using jQuery Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.