Digital signatures are essential in document verification and security, particularly in the digital era. By including digital signatures in PDF documents, you may assure their authenticity and integrity. This tutorial will walk you through the steps of adding digital signatures to PDF documents in Laravel 10, the most recent version of the popular PHP framework.
Digital signatures validate the signer’s identity and confirm that the document’s content has not been tampered with. They are used for signing contracts, agreements, and crucial papers in a variety of areas, including legal, finance, and government.
By the end of this lesson, you’ll have the knowledge and skills to add digital signatures to PDF documents in your Laravel 10 project, hence improving document security and integrity.
Read More: How To Validate Image Upload in Laravel 10 Example
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Installation of Laravel DomPDF Package
Here, we will install a pdf package to laravel application.
Open project terminal and run this command,
DomPDF Package
composer require barryvdh/laravel-dompdf
Setup Signature PDF Controller
Back to project terminal and run this command into it,
php artisan make:controller SignaturePDFController
It will create a SignaturePDFController.php file inside /app/Http/Controllers folder.
Open file and write this code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Barryvdh\DomPDF\Facade\Pdf; use Illuminate\View\View; class SignaturePDFController extends Controller { public function index(): View { return view('signature-pdf'); } public function upload(Request $request) { $data['image'] = $this->uploadSignature($request->signed); $pdf = Pdf::loadView('signature-pdf-view', $data); return $pdf->download('onlinewebtutor.pdf'); } public function uploadSignature($signed) { $folderPath = public_path('uploads/'); $image_parts = explode(";base64,", $signed); $image_type_aux = explode("image/", $image_parts[0]); $image_type = $image_type_aux[1]; $image_base64 = base64_decode($image_parts[1]); $file = $folderPath . uniqid() . '.'.$image_type; file_put_contents($file, $image_base64); return $file; } }
Read More: Laravel 10 Convert Word Docx File to PDF File Example
Create Blade Template
Create a file named as signature-pdf.blade.php and one more view file with name signature-pdf-view.blade.php inside /resources/views folder.
Open signature-pdf.blade.php file (This is the layout where you can sign your document with PDF content) and write this code into it,
<html> <head> <title>How To Add Digital Signature To PDF in Laravel 10 Tutorial</title> <link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.3.1/css/bootstrap.css"> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <link type="text/css" href="http://ajax.googleapis.com/ajax/libs/jqueryui/1.12.1/themes/south-street/jquery-ui.css" rel="stylesheet"> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jqueryui/1.12.1/jquery-ui.min.js"></script> <script type="text/javascript" src="http://keith-wood.name/js/jquery.signature.js"></script> <link rel="stylesheet" type="text/css" href="http://keith-wood.name/css/jquery.signature.css"> <style> .kbw-signature { width: 300px; height: 200px; } #sig canvas { width: 300px; height: 200px; border: 1px solid #a1a1a1; } </style> </head> <body> <div class="container"> <div class="row"> <div class="col-md-10 offset-md-1 mt-5"> <div class="card"> <div class="card-header"> <h5>How To Add Digital Signature To PDF in Laravel 10 Tutorial</h5> </div> <div class="card-body"> @if ($message = Session::get('success')) <div class="alert alert-success alert-dismissible"> <button type="button" class="close" data-dismiss="alert">×</button> <strong>{{ $message }}</strong> </div> @endif <form method="POST" action="{{ route('signature.upload') }}" enctype="multipart/form-data"> @csrf <p>Dear Boss, <br /><br /> I am writing to formally resign from my position as [Your Position] at [IT Company Name], with my last working day being [Last Working Day], in accordance with the notice period stipulated in my employment contract. <br /><br /> I want to express my sincere gratitude for the opportunities and experiences I've had during my time at [IT Company Name]. It has been an incredible journey, and I have had the privilege of working alongside an outstanding team of professionals. <br /><br /> After careful consideration, I have decided to take a new direction in my career. This decision wasn't easy, as I have cherished my time here and the projects we've accomplished together. I am immensely proud of our collective achievements. <br /><br /> During my notice period, I am committed to ensuring a seamless transition. I am more than willing to assist in the transfer of my responsibilities, provide training to my successor, and complete any pending projects. Please let me know how I can best contribute to this process. <br /><br /> Sincerely, [Emloyee Name] </p> <div class="col-md-12"> <strong>Signature:</strong> <br /> <div id="sig"></div> <br /> <button id="clear" class="btn btn-danger btn-sm mt-1">Clear Signature</button> <textarea id="signature64" name="signed" style="display: none"></textarea> </div> <br /> <div class="col-md-12 text-center"> <button class="btn btn-success">Submit & Download PDF</button> </div> </form> </div> </div> </div> </div> </div> <script type="text/javascript"> $(function() { var sig = $('#sig').signature({ syncField: '#signature64', syncFormat: 'PNG' }); $('#clear').click(function(e) { e.preventDefault(); sig.signature('clear'); $("#signature64").val(''); }); }); </script> </body> </html>
Open signature-pdf-view.blade.php file (This is the preview of your PDF document with digital signature) and write this code into it,
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title></title> </head> <body> <p>Dear Boss, <br /><br /> I am writing to formally resign from my position as [Your Position] at [IT Company Name], with my last working day being [Last Working Day], in accordance with the notice period stipulated in my employment contract. <br /><br /> I want to express my sincere gratitude for the opportunities and experiences I've had during my time at [IT Company Name]. It has been an incredible journey, and I have had the privilege of working alongside an outstanding team of professionals. <br /><br /> After careful consideration, I have decided to take a new direction in my career. This decision wasn't easy, as I have cherished my time here and the projects we've accomplished together. I am immensely proud of our collective achievements. <br /><br /> During my notice period, I am committed to ensuring a seamless transition. I am more than willing to assist in the transfer of my responsibilities, provide training to my successor, and complete any pending projects. Please let me know how I can best contribute to this process. <br /><br /> Sincerely, <br /> [Employee Name] <br /> <br /> <img src="{{ $image }}" style="border: 1px solid #a1a1a1;"> </p> </body> </html>
Add Route
Open web.php from /routes folder. Add this route into it,
//... use App\Http\Controllers\SignaturePDFController; Route::get('signature-pdf', [SignaturePDFController::class, 'index']); Route::post('signature-pdf', [SignaturePDFController::class, 'upload'])->name('signature.upload'); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Read More: Laravel 10 Model Create Events Example Tutorial
URL: http://127.0.0.1:8000/signature-pdf
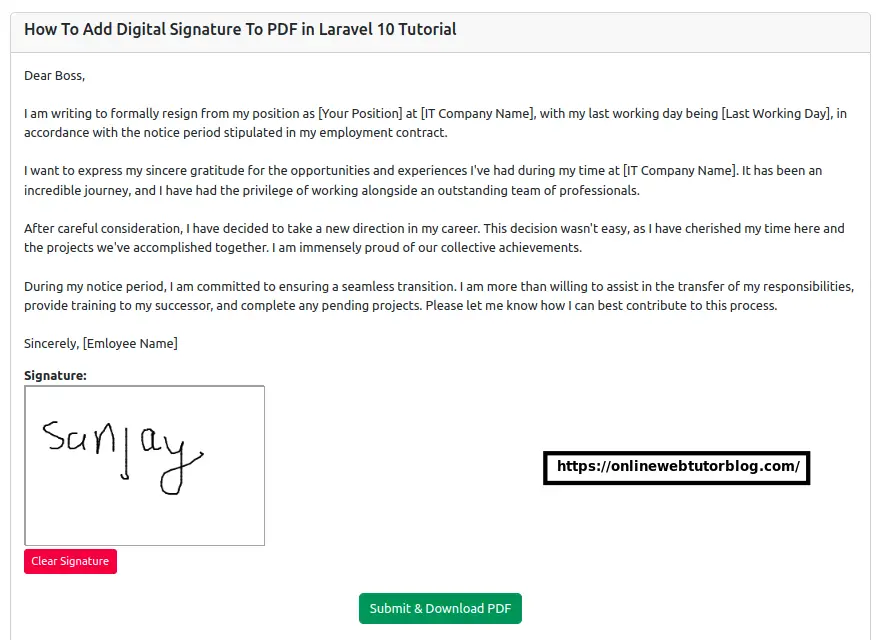
When you click on “Submit & Download PDF”
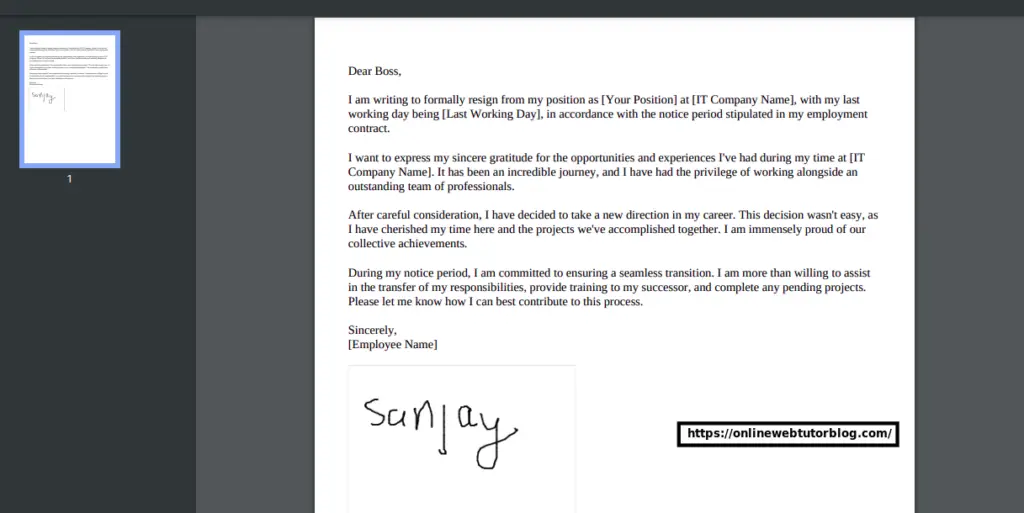
That’s it.
We hope this article helped you to learn about How To Add Digital Signature To PDF in Laravel 10 Tutorial Example in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.