Inside this article we will see the concept i.e Laravel 9 Autocomplete Places Search Box using Google Maps JavaScript API Tutorial. Article contains the classified information about google places autocomplete search box using google map api and JavaScript.
If you are looking for a solution i.e how to use google place api to create autocomplete search box for places in Laravel then this article will help you a lot for this. Tutorial is super easy to understand and implement it in your code as well.
Note that, Google autocomplete address API will return address and as well as latitude, longitude, place code, state, city, country, etc. Using latitude and longitude of address, you can show markers location in google map dynamically.
Learn More –
- Laravel 9 Drag and Drop Reorder Items with jQuery
- CodeIgniter 4 How To Use Froala WYSIWYG HTML Editor Tutorial
- CodeIgniter 4 Drag and Drop Reorder Items with jQuery
- PHP & MySQLi Drag and Drop Reorder Items with jQuery
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Getting Started with Google Map API Key
Before using the Places library in the Maps JavaScript API, first you need to make sure that the Places API is enabled in the Google Cloud Console, in the same project you set up for the Maps JavaScript API.
To view your list of enabled APIs:
- Go to the Google Cloud Console.
- Click the Select a project button, then select the same project you set up for the Maps JavaScript API and click Open.
- From the list of APIs on the Dashboard, look for Places API.
- If you see the API in the list, you’re all set. If the API is not listed, enable it:
- At the top of the page, select ENABLE API to display the Library tab. Alternatively, from the left side menu, select Library.
- Search for Places API, then select it from the results list.
- Select ENABLE. When the process finishes, Places API appears in the list of APIs on the Dashboard.
Map API Key will look like this:
AIzaxxxXSQRl-XLDybXXX9cBcNtoZ1HXXxXxxXX
Assuming you have your correct Map API Key.
Create Controller
Open project into terminal and run this command to create controller file.
$ php artisan make:controller PlaceController
It will create a file PlaceController.php inside /app/Http/Controllers folder.
Open this controller file and write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class PlaceController extends Controller { public function index() { return view("places"); } }
Create Blade Template File
Next,
Create a fie places.blade.php inside /resources/views folder.
Open places.blade.php and write this content into it.
<!doctype html> <html lang="en"> <head> <title>Laravel 9 Autocomplete Places Search Box using Google Maps JavaScript API</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <script src="https://code.jquery.com/jquery-3.4.1.js"></script> </head> <body> <div class="container mt-5"> <div class="row"> <div class="col-xl-10 col-lg-10 col-md-10 col-sm-12 col-12 m-auto"> <div class="card shadow"> <div class="card-header bg-primary"> <h5 class="card-title text-white">Laravel 9 Autocomplete Places Search Box using Google Maps JavaScript API</h5> </div> <div class="card-body"> <div class="form-group"> <label for="autocomplete"> Location </label> <input type="text" name="autocomplete" id="autocomplete" class="form-control" placeholder="Select Location"> </div> <div class="form-group" id="lat_area"> <label for="latitude"> Latitude </label> <input type="text" name="latitude" id="latitude" class="form-control"> </div> <div class="form-group" id="long_area"> <label for="latitude"> Longitude </label> <input type="text" name="longitude" id="longitude" class="form-control"> </div> </div> </div> </div> </div> </div> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script> <script src="https://maps.google.com/maps/api/js?key=AIzaxxxXSQRl-XLDybXXX9cBcNtoZ1HXXxXxxXX&libraries=places&callback=initAutocomplete" type="text/javascript"></script> <script src="{{ asset('map.js') }}"></script> </body> </html>
When page loads, initAutocomplete() will be called.
<script src="https://maps.google.com/maps/api/js?key=AIzaxxxXSQRl-XLDybXXX9cBcNtoZ1HXXxXxxXX&libraries=places&callback=initAutocomplete" type="text/javascript"></script>
Create ‘map.js’ File
Create a file map.js inside /public folder. Inside this file we will write code for map settings.
Open map.js file and write this code into it.
//... $(document).ready(function() { $("#lat_area").addClass("d-none"); $("#long_area").addClass("d-none"); }); google.maps.event.addDomListener(window, 'load', initialize); function initialize() { var input = document.getElementById('autocomplete'); var autocomplete = new google.maps.places.Autocomplete(input); autocomplete.addListener('place_changed', function() { var place = autocomplete.getPlace(); $('#latitude').val(place.geometry['location'].lat()); $('#longitude').val(place.geometry['location'].lng()); // --------- show lat and long --------------- $("#lat_area").removeClass("d-none"); $("#long_area").removeClass("d-none"); }); } //...
Add Route
Open web.php from /routes folder. Add this route into it.
//... use App\Http\Controllers\PlaceController; Route::get('places', [PlaceController::class, "index"]); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/places
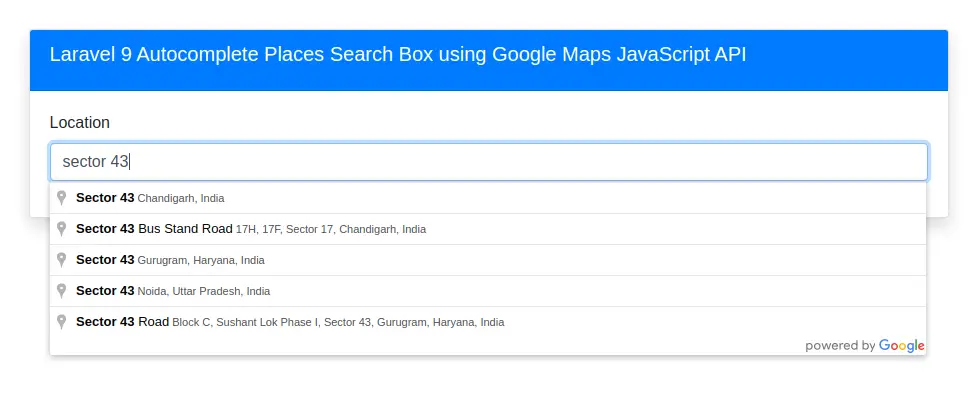
Whenever you search and click on any location, you will get latitude and longitude of selected location.
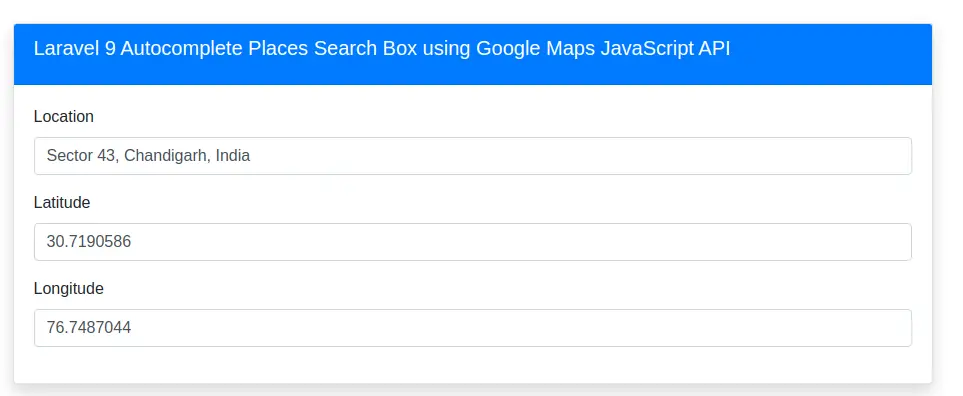
We hope this article helped you to learn Laravel 9 Autocomplete Places Search Box using Google Maps JavaScript API in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.