Inside this article we will see laravel 9 how to override auth login method using laravel ui package. laravel ui package provides the simple steps for authentication scaffolding. We will see the step by step process to change login form into a custom login form of laravel 9 authentication.
If you are looking for an article which gives you the understanding to override the default auth login method then you are at right place to learn.
Laravel authentication via laravel ui package provides the simple steps to override auth register method, login method etc.
Learn More –
- Laravel 9 How to Send Automatic Email Tutorial
- Laravel 9 How To Send Email To Multiple Users Tutorial
- Laravel 9 How To Install Tailwind CSS in Application
- Laravel 9 How To Generate Dynamic Sitemap Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Authentication with Laravel UI
To learn complete process of laravel authentication with laravel ui package, click here. This will provide you the step by step solution to get into laravel authentication.
Next,
We will see how to change or override auth login method of laravel authentication.
Authentication Routes
Open project into terminal, if you type this command.
$ php artisan route:list
You will see all hidden routes of laravel authentication.
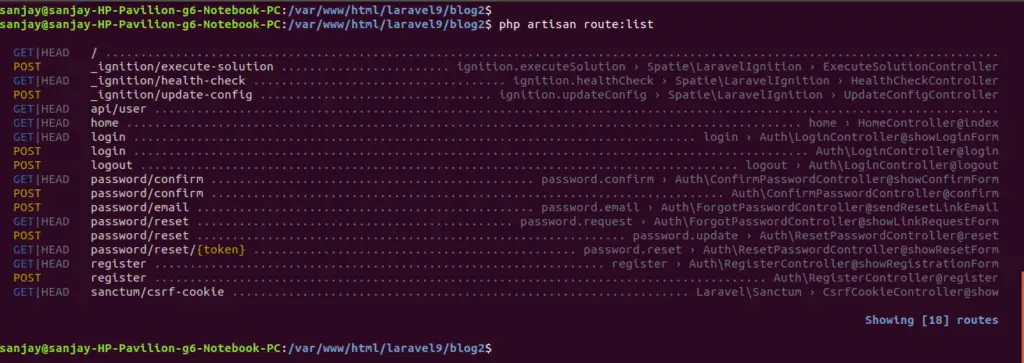
For login auth methods, laravel ui provides these methods
Method for Registration form, showLoginForm() from LoginController.
GET|HEAD | login | login | App\Http\Controllers\Auth\LoginController@showLoginForm
Method to submit login form, login() from LoginController will be called.
POST | login | | App\Http\Controllers\Auth\LoginController@login
To override login form we need to work on showLoginForm() method.
Override Login in Authentication
Open LoginController.php file from /app/Http/Controllers/Auth folder.
<?php namespace App\Http\Controllers\Auth; use App\Http\Controllers\Controller; use App\Providers\RouteServiceProvider; use Illuminate\Foundation\Auth\AuthenticatesUsers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Auth; class LoginController extends Controller { /* |-------------------------------------------------------------------------- | Login Controller |-------------------------------------------------------------------------- | | This controller handles authenticating users for the application and | redirecting them to your home screen. The controller uses a trait | to conveniently provide its functionality to your applications. | */ use AuthenticatesUsers; /** * Where to redirect users after login. * * @var string */ protected $redirectTo = RouteServiceProvider::HOME; /** * Create a new controller instance. * * @return void */ public function __construct() { $this->middleware('guest')->except('logout'); } // Custom Login form public function showLoginForm() { return view("login"); } // Add mobile number to login public function login(Request $request) { $request->validate([ 'mobile' => 'required', 'password' => 'required', ]); $credentials = $request->only('mobile', 'password'); if (Auth::attempt($credentials)) { return redirect()->route('home'); } return redirect("login")->withSuccess('Oppes! You have entered invalid credentials'); } }
We have added a method showLoginForm() to override the default auth login page.
Also, we updated login() method for login via mobile number and password.
Next,
We need to create login.blade.php layout file inside /resources/views folder.
Custom Login Blade Template
Create a layout file named as login.blade.php inside /resources/views folder.
Open file and write this complete code into it.
@extends('layouts.app') @section('content') <div class="container"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header">{{ __('Login') }}</div> <div class="card-body"> <form method="POST" action="{{ route('login') }}"> @csrf <div class="row mb-3"> <label for="mobile" class="col-md-4 col-form-label text-md-right">{{ __('Mobile Number') }}</label> <div class="col-md-6"> <input id="mobile" type="text" class="form-control @error('mobile') is-invalid @enderror" name="mobile" value="{{ old('mobile') }}" required autocomplete="mobile" autofocus> @error('mobile') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <label for="password" class="col-md-4 col-form-label text-md-right">{{ __('Password') }}</label> <div class="col-md-6"> <input id="password" type="password" class="form-control @error('password') is-invalid @enderror" name="password" required autocomplete="current-password"> @error('password') <span class="invalid-feedback" role="alert"> <strong>{{ $message }}</strong> </span> @enderror </div> </div> <div class="row mb-3"> <div class="col-md-6 offset-md-4"> <div class="form-check"> <input class="form-check-input" type="checkbox" name="remember" id="remember" {{ old('remember') ? 'checked' : '' }}> <label class="form-check-label" for="remember"> {{ __('Remember Me') }} </label> </div> </div> </div> <div class="row mb-0"> <div class="col-md-8 offset-md-4"> <button type="submit" class="btn btn-primary"> {{ __('Login') }} </button> @if (Route::has('password.request')) <a class="btn btn-link" href="{{ route('password.request') }}"> {{ __('Forgot Your Password?') }} </a> @endif </div> </div> </form> </div> </div> </div> </div> </div> @endsection
We have added a mobile number input field in place of Email address as login input.
Note*: When you override login() method, first you need to add custom field for mobile number into database table i.e mobile. For custom registration tutorial, learn here.
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/
Click on Login link of dashboard.
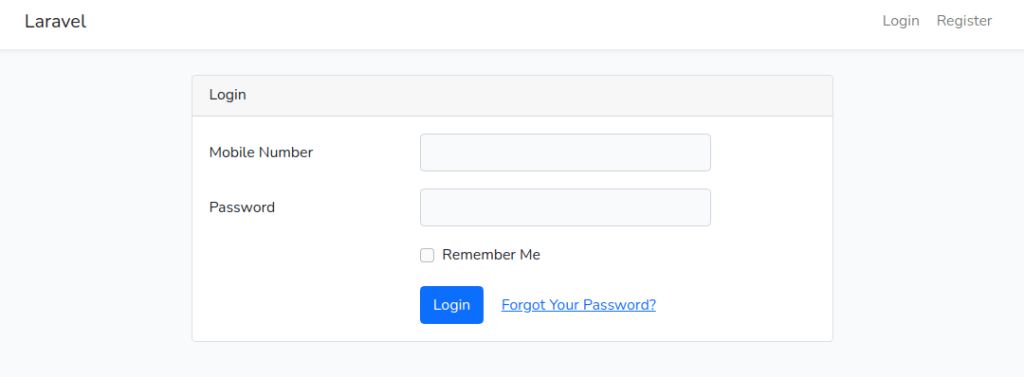
When you provide data Mobile and password then hit on Login button, it will login user to the dashboard.
We hope this article helped you to learn Laravel 9 How to Override Auth Login Method in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.