We create wordpress admin tables using WP_List_Table class to show data. In the last tutorial we seen about creating table header columns.
Now, How to bind data with table. We will discuss in this tutorial.
For data to show, we have two options. Either we can show a set of static data into table. Static data means we can’t change it once fixed. Or we can show dynamic data from wordpress database table. We will discuss both ways tutorial by tutorial.
Inside this we will use the concept of static data i.e a fixed amount of data to be shown inside table.
Prepare Example Data
We will create our example data for table inside a class variable.
private $table_data = array(
array(...), array(...), ...
);
We will use this data variable inside prepare_items() method as well as bind data with column name.
Define Column Names
We will use get_columns() method to define header column name.
function get_columns()
{
$columns = array(
'cb' => '<input type="checkbox" />',
'id' => 'ID',
'name' => 'Name',
'email' => 'Email',
'designation' => 'Designation',
'salary' => 'Salary'
);
return $columns;
}
Here, we have the columns as a checkbox, ID, Name, Email, Designation, Salary.
Create Data For Table
We need to use the above created data to list into table.
function prepare_items()
{
...
$this->items = $this->table_data;
}
Now, we can use the table data for admin table. $items is variable coming from WP_List_Table class. We have initialized data into it.
To bind data with table columns, we will use default method for table columns as –
Bind Data To Table Column
function column_default($item, $column_name)
{
switch ($column_name) {
case 'id':
case 'name':
case 'email':
case 'designation':
case 'salary':
return $item[$column_name];
default:
return print_r($item, true); //Show the whole array for troubleshooting purposes
}
}
column_default() method is default method to perform operations with all table columns as we can see we have mentioned in switch case. $item[$column_name] returns the data from $item array according to $column_name. This is the method taken from WP_List_Table clas.
Additionally we have specific method as well to do individual operation with each column.
Alternatively also we can create column wise method to do a different function for each column column_id(), column_name(), column_name()… Syntax used column_{column_name}() method. We will discuss more about this in coming tutorials.
Checkbox for Each Row
To show checkbox for each row of table, we need to write like this. This method will bind checkbox for each row. Also we can see the syntax used to create this method as column_cb(). cb refers to identifier for checkbox.
function column_cb($item)
{
return sprintf(
'<input type="checkbox" name="emp[]" value="%s" />',
$item['id']
);
}
Also $item[‘id’] returns id of each row.
In code it will generate like this –
<input type="checkbox" name="emp[]" value="1">
So let’s see how to setup data with table via plugin.
Plugin Setup
Here, is the complete code of plugin.
<?php
/*
Plugin Name: Simple Employees Table
Description: It displays a table with employee data
Author: Online Web Tutor
Author URI: https://onlinewebtutorblog.com/
License: GPLv2 or later
License URI: https://www.gnu.org/licenses/gpl-2.0.html
Text Domain: basic-wp-list-table
Version: 1.0
*/
// Loading table class
if (!class_exists('WP_List_Table')) {
require_once(ABSPATH . 'wp-admin/includes/class-wp-list-table.php');
}
// Extending class
class Employees_List_Table extends WP_List_Table
{
private $table_data = array(
array(
'id' => 1,
'name' => "Sanjay Kumar",
'email' => 'example1@mail.net',
'designation' => 'Software Developer',
'salary' => '80,000'
),
array(
'id' => 2,
'name' => "Vijay Kumar",
'email' => 'example2@mail.net',
'designation' => 'Python Developer',
'salary' => '60,000'
),
array(
'id' => 3,
'name' => "Ashish Kumar",
'email' => 'example3@mail.net',
'designation' => 'Android Developer',
'salary' => '90,000'
),
array(
'id' => 4,
'name' => "Dhananjay Negi",
'email' => 'example4@mail.net',
'designation' => 'Mean Stack Developer',
'salary' => '80,000'
)
);
// Define table columns
function get_columns()
{
$columns = array(
'cb' => '<input type="checkbox" />',
'id' => 'ID',
'name' => 'Name',
'email' => 'Email',
'designation' => 'Designation',
'salary' => 'Salary'
);
return $columns;
}
// Bind table with columns, data and all
function prepare_items()
{
$columns = $this->get_columns();
$hidden = array();
$sortable = array();
$this->_column_headers = array($columns, $hidden, $sortable);
$this->items = $this->table_data;
}
// bind data with column
function column_default($item, $column_name)
{
switch ($column_name) {
case 'id':
case 'name':
case 'email':
case 'designation':
case 'salary':
return $item[$column_name];
default:
return print_r($item, true); //Show the whole array for troubleshooting purposes
}
}
function column_cb($item)
{
return sprintf(
'<input type="checkbox" name="emp[]" value="%s" />',
$item['id']
);
}
//...
}
// Adding menu
function my_add_menu_items()
{
add_menu_page('Employees List Table', 'Employees List Table', 'activate_plugins', 'employees_list_table', 'employees_list_init');
}
add_action('admin_menu', 'my_add_menu_items');
// Plugin menu callback function
function employees_list_init()
{
// Creating an instance
$empTable = new Employees_List_Table();
echo '<div class="wrap"><h2>Employees List Table</h2>';
// Prepare table
$empTable->prepare_items();
// Display table
$empTable->display();
echo '</div>';
}
Plugin
Go to Plugins >> Installed Plugins

Click on Activate
It will create a admin menu. When we click on it.
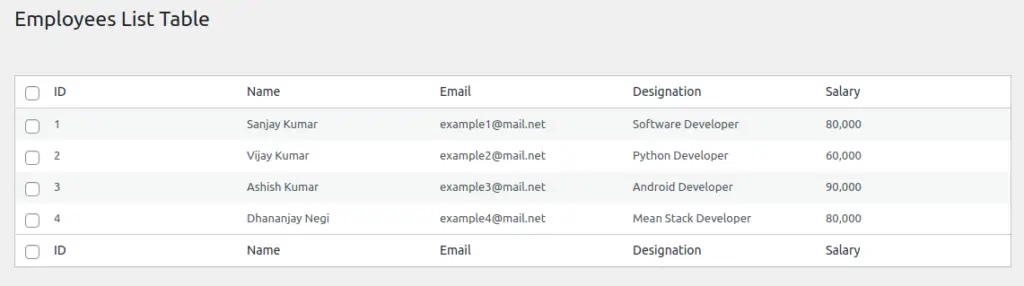
We will add more functions for data, sorting in coming tutorials.