We create wordpress admin tables using WP_List_Table class to show data. Suppose we have large amount of data. To show data into a perfect format we need some pagination kind of feature. This class also provides method to add pagination. In this tutorial we will see how to add data pagination to WordPress admin table.
Data pagination means data will come according to pagination settings. This is also a bit logical implementation you need to focus on.
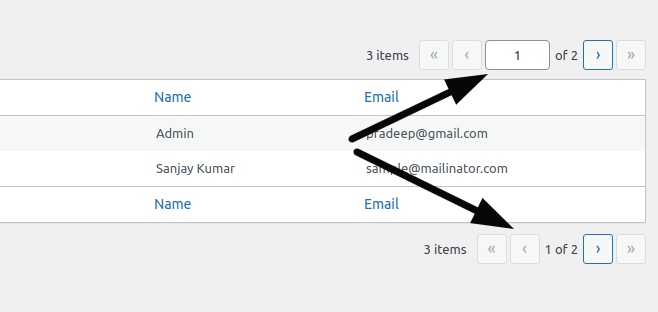
Probably while working with wordpress admin tables of Post, Pages, Users, etc you may or may not seen this pagination. This pagination works on the basis of rows available to list table.
We will see here how to add pagination to wordpress admin table.
Add Pagination to List Table
WP_List_Table class provides a method to add data pagination feature to list tables. Assuming we are adding data pagination to users data.
In this last tutorials we have seen how to show users data into list table.
/* pagination */
$per_page = 2;
$current_page = $this->get_pagenum();
$total_items = count($this->users_data);
$this->users_data = array_slice($this->users_data, (($current_page - 1) * $per_page), $per_page);
$this->set_pagination_args(array(
'total_items' => $total_items, // total number of items
'per_page' => $per_page // items to show on a page
));
$per_page = 2 means total 2 rows will show on each page.
$this->get_pagenum(), method is provided by WP_List_Table class. It returns the current page number. Like for first page it will be 1, for second page it will be 2 and so on.
array_slice(), it’s a PHP function which picks data on the basis of start index.
$this->set_pagination_args(), method is provided by WP_List_Table class. It takes values in an array. Array values are like count of total items and how many rows on each page.
This is the only whole concept we need to add inside prepare_items() method to add pagination. That’s it.
Plugin Setup
Here, is the complete code of plugin.
<?php
/*
Plugin Name: Simple Employees Table
Description: It displays a table with employee data
Author: Online Web Tutor
Author URI: https://onlinewebtutorblog.com/
License: GPLv2 or later
License URI: https://www.gnu.org/licenses/gpl-2.0.html
Text Domain: basic-wp-list-table
Version: 1.0
*/
// Loading table class
if (!class_exists('WP_List_Table')) {
require_once(ABSPATH . 'wp-admin/includes/class-wp-list-table.php');
}
// Extending class
class Employees_List_Table extends WP_List_Table
{
private $users_data;
private function get_users_data()
{
global $wpdb;
return $wpdb->get_results(
"SELECT ID,user_login,user_email,display_name from {$wpdb->prefix}users",
ARRAY_A
);
}
// Define table columns
function get_columns()
{
$columns = array(
'cb' => '<input type="checkbox" />',
'ID' => 'ID',
'user_login' => 'Username',
'display_name' => 'Name',
'user_email' => 'Email'
);
return $columns;
}
// Bind table with columns, data and all
function prepare_items()
{
$this->users_data = $this->get_users_data();
$columns = $this->get_columns();
$hidden = array();
$sortable = $this->get_sortable_columns();
$this->_column_headers = array($columns, $hidden, $sortable);
/* pagination */
$per_page = 2;
$current_page = $this->get_pagenum();
$total_items = count($this->users_data);
$this->users_data = array_slice($this->users_data, (($current_page - 1) * $per_page), $per_page);
$this->set_pagination_args(array(
'total_items' => $total_items, // total number of items
'per_page' => $per_page // items to show on a page
));
usort($this->users_data, array(&$this, 'usort_reorder'));
$this->items = $this->users_data;
}
// bind data with column
function column_default($item, $column_name)
{
switch ($column_name) {
case 'ID':
case 'user_login':
case 'user_email':
return $item[$column_name];
case 'display_name':
return ucwords($item[$column_name]);
default:
return print_r($item, true); //Show the whole array for troubleshooting purposes
}
}
// To show checkbox with each row
function column_cb($item)
{
return sprintf(
'<input type="checkbox" name="user[]" value="%s" />',
$item['ID']
);
}
// Add sorting to columns
protected function get_sortable_columns()
{
$sortable_columns = array(
'user_login' => array('user_login', false),
'display_name' => array('display_name', false),
'user_email' => array('user_email', true)
);
return $sortable_columns;
}
// Sorting function
function usort_reorder($a, $b)
{
// If no sort, default to user_login
$orderby = (!empty($_GET['orderby'])) ? $_GET['orderby'] : 'user_login';
// If no order, default to asc
$order = (!empty($_GET['order'])) ? $_GET['order'] : 'asc';
// Determine sort order
$result = strcmp($a[$orderby], $b[$orderby]);
// Send final sort direction to usort
return ($order === 'asc') ? $result : -$result;
}
}
// Adding menu
function my_add_menu_items()
{
add_menu_page('Employees List Table', 'Employees List Table', 'activate_plugins', 'employees_list_table', 'employees_list_init');
}
add_action('admin_menu', 'my_add_menu_items');
// Plugin menu callback function
function employees_list_init()
{
// Creating an instance
$empTable = new Employees_List_Table();
echo '<div class="wrap"><h2>Employees List Table</h2>';
// Prepare table
$empTable->prepare_items();
// Display table
$empTable->display();
echo '</div>';
}
Plugin
Go to Plugins >> Installed Plugins

Click on Activate
It will create a admin menu. When we click on it.
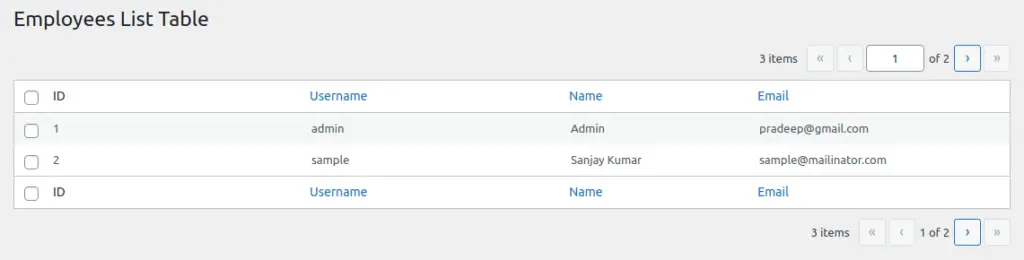
Now, successfully we have implemented the pagination feature to wordpress admin table.