Dynamic carousel sliders are a popular and engaging way to showcase dynamic content such as images, products, or announcements in web applications. In Laravel 10, implementing a dynamic carousel slider enables developers to display and manage interactive sliders with changing content seamlessly.
In this tutorial, we’ll see the comprehensive process of creating a dynamic carousel slider in Laravel 10. This functionality empowers developers to build a flexible and customizable carousel slider that fetches content dynamically from the backend, providing a user-friendly and visually appealing way to present dynamic content.
Read More: How To Use SweetAlert Message Box in Laravel 10 Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Setup Model and Table Migration
Open project into terminal and run this command,
php artisan make:model Slider -m
It will create a model file app/Models/Slider.php and a migration file xxx_create_sliders_table.php inside /database/migrations folder.
Open migration file and write this complete code into it,
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('sliders', function (Blueprint $table) { $table->id(); $table->string('title'); $table->string('url'); $table->timestamps(); }); } /** * Reverse the migrations. */ public function down(): void { Schema::dropIfExists('sliders'); } };
Run Migration
Next, you need to migrate migration files,
php artisan migrate
This command will create tables inside database.
Read More: How To Read PDF File Contents in Laravel 10 Example
Open app/Models/Slider.php and write this complete code into it,
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Slider extends Model { use HasFactory; protected $fillable = [ 'title', 'url' ]; }
Create Database Seeder Class
Back to project terminal and run this command,
php artisan make:seeder SliderSeeder
It will create a file database/seeders/SliderSeeder.php.
Open file and write this complete code into it,
<?php namespace Database\Seeders; use Illuminate\Database\Console\Seeds\WithoutModelEvents; use Illuminate\Database\Seeder; use App\Models\Slider; class SliderSeeder extends Seeder { /** * Run the database seeds. */ public function run(): void { $sliders = [ [ 'title' => 'Image 1', 'url' => 'https://picsum.photos/seed/picsum/700/400' ], [ 'title' => 'Image 2', 'url' => 'https://picsum.photos/id/237/700/400' ], [ 'title' => 'Image 3', 'url' => 'https://picsum.photos/id/200/700/400' ] ]; foreach ($sliders as $key => $value) { Slider::create($value); } } }
Next,
You have to run the seeder command to see this,
php artisan db:seed --class=SliderSeeder
Create Slider Controller
Next, you need to create a controller class,
php artisan make:controller SliderController
It will create a file SliderController.php inside /app/Http/Controllers folder.
Read More: Work with VUE 3 Axios Get Request in Laravel 10 Example
Open file and write this complete code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Slider; class SliderController extends Controller { public function index() { $sliders = Slider::get(); return view('sliders', compact('sliders')); } }
Create Blade Template File
Go to resources/views folder and create a file with name sliders.blade.php
Open file and write this complete code into it,
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Carousel Slider in Laravel</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="container"> <h3>Carousel Slider in Laravel by Online Web Tutor</h3> <div id="carouselExampleIndicators" class="carousel slide" data-bs-ride="carousel"> <div class="carousel-inner"> @foreach($sliders as $key => $slider) <div class="carousel-item {{$key == 0 ? 'active' : ''}}"> <img src="{{ $slider->url }}" class="d-block w-100" alt="{{ $slider->title }}"> </div> @endforeach </div> <button class="carousel-control-prev" type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide="prev"> <span class="carousel-control-prev-icon" aria-hidden="true"></span> <span class="visually-hidden">Previous</span> </button> <button class="carousel-control-next" type="button" data-bs-target="#carouselExampleIndicators" data-bs-slide="next"> <span class="carousel-control-next-icon" aria-hidden="true"></span> <span class="visually-hidden">Next</span> </button> </div> </div> </body> </html>
Add Route
Open web.php file from routes folder. Add this route into it.
//... use App\Http\Controllers\SliderController; //... Route::get('sliders', [SliderController::class, 'index']);
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/sliders
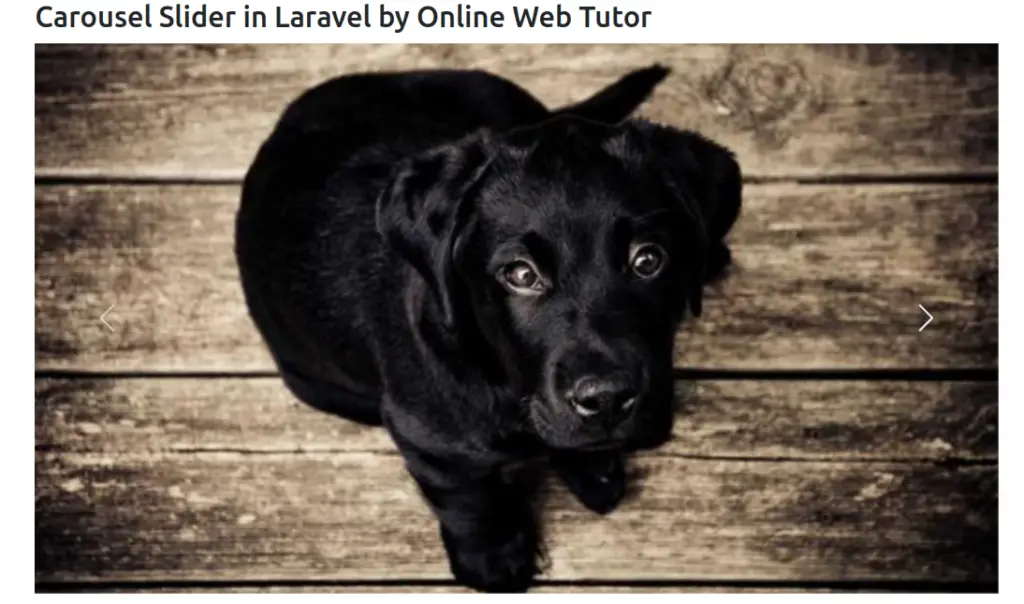
That’s it.
We hope this article helped you to learn about How To Create Dynamic Carousel Slider in Laravel 10 Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.