A dynamic sitemap is a crucial tool in the realm of web development, assisting search engines in efficiently crawling and indexing your website’s content. Creating a dynamic sitemap in Laravel 10 allows you to automatically build and update the sitemap anytime your content changes.
It ensures that search engines always have the most up-to-date information about the structure of your website.
Read More: Laravel 10 LogViewer: A Beautiful Log Viewer
This tutorial will walk you through the steps of creating a dynamic sitemap in Laravel 10. We’ll look at how to create a route, generate XML-based sitemap content, and integrate it with your app. You may ensure that new pages, modifications, and removals are reflected in the sitemap by dynamically producing it.
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Model & Migration
Open project into terminal and run this command.
$ php artisan make:model Post -m
This command will create two files.
- A model file Post.php inside /app/Models folder.
- A migration file 2023_08_21_031111_create_posts_table.php inside /database/migrations folder.
Read More: Laravel 10 Telescope: Debugging and Monitoring Tool
Open xxx_create_posts_table.php migration file and write this code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. */ public function up(): void { Schema::create('posts', function (Blueprint $table) { $table->id(); $table->string('title'); $table->string('slug'); $table->text('body'); $table->timestamps(); }); } /** * Reverse the migrations. */ public function down(): void { Schema::dropIfExists('posts'); } };
Run Migration
Back to project terminal and run this command,
$ php artisan migrate
It will run all migrations and create their respective tables.
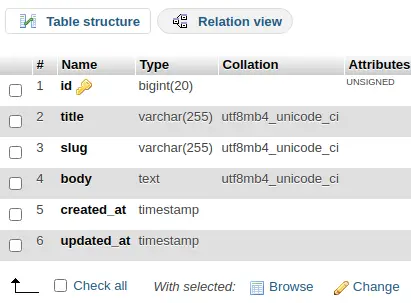
Open Post.php model file from /app/Models folder.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Post extends Model { use HasFactory; protected $fillable = [ 'title', 'slug', 'body' ]; }
Next,
Setup Post Factory (Test Data)
Open project terminal and run this command.
$ php artisan make:factory PostFactory
It will create a file PostFactory.php file inside /database/factories folder.
Read More: Laravel 10 Working with MySQL Joins Example
Open file and write this complete code into it.
<?php namespace Database\Factories; use Illuminate\Database\Eloquent\Factories\Factory; use Illuminate\Support\Str; /** * @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Post> */ class PostFactory extends Factory { /** * Define the model's default state. * * @return array<string, mixed> */ public function definition(): array { return [ 'title' => $this->faker->text(), 'slug' => Str::slug($this->faker->text()), 'body' => $this->faker->paragraph() ]; } }
Process of Data Seeding (Run Factory File)
Back to project terminal and execute these commands –
$ php artisan tinker
It will open tinker shell.
Next, run this command to create 50 fake rows for posts table.
>>> App\Models\Post::factory()->count(50)->create();
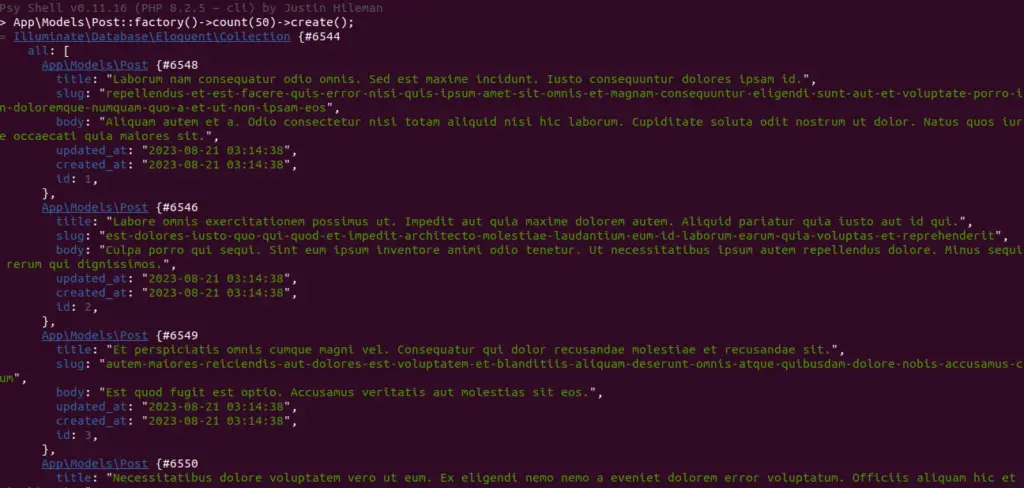
Now,
Create Controller
Back to terminal and run this artisan command,
$ php artisan make:controller SitemapController
It will create SitemapController.php file inside /app/Http/Controllers folder.
Read More: Laravel 10 Seed Database Using Faker Library Tutorial
Open file and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Post; class SitemapController extends Controller { public function index() { $posts = Post::latest()->get(); return response()->view('sitemap', [ 'posts' => $posts ])->header('Content-Type', 'text/xml'); } }
Create Template File
Create a file sitemap.blade.php inside /resources/views folder.
Open file and write this code into it.
<?php echo '<?xml version="1.0" encoding="UTF-8"?>'; ?> <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> @foreach ($posts as $post) <url> <loc>{{ url('/') }}/post/{{ $post->slug }}</loc> <lastmod>{{ $post->created_at->tz('UTC')->toAtomString() }}</lastmod> <changefreq>daily</changefreq> <priority>0.8</priority> </url> @endforeach </urlset>
Above is the structure which will be used and generates a dynamic sitemap.xml file.
Add Route
Open web.php file from /routes folder. Add this route into it.
//... use App\Http\Controllers\SitemapController; Route::get('sitemap.xml', [SitemapController::class, 'index']); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/sitemap.xml
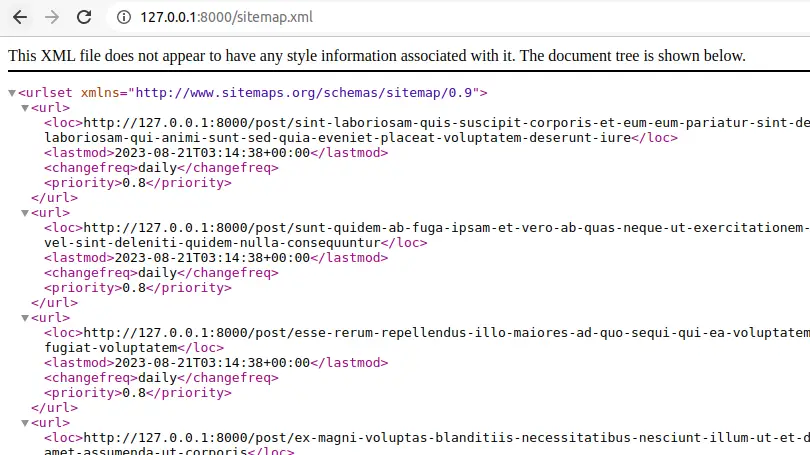
Read More: Laravel 10 How To Setup Ajax Request Tutorial
We hope this article helped you to learn about Laravel 10 Generate Dynamic Sitemap Example in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.