Routing simply means mapping of application URLs to view files. These are responsible to handle HTTP requests. We can use request type GET, POST, PUT, DELETE according to need.
Routes configuration for web application we do via web.php file. Also, we have a different file to handle APIs request that is by api.php. Inside this article we will see Laravel 8 routing in complete details.
Learn More –
- API Authentication using Laravel 8 Sanctum Tutorial
- Bar Chart Integration with Laravel 8 Tutorial Example
- Barcode Generator in Laravel 8 Tutorial
- Bootstrap Growl jQuery Notification Plugin to Laravel 8
We will start this tutorial with very basic concept and ends up with great detail.
Let’s get started.
Laravel 8 Application Installation
Laravel is an open source widely used PHP Framework for web applications. It’s syntax, working principle is so much expressive and elegant. Development of web application in Laravel framework nowdays very popular among developers. Laravel is packed of strong features and/or module for the development environment.
While working and/or developing any application in laravel 8 version. We should have some PHP and system configuration need. Already we have a tutorial over Laravel 8 installation. Click here to go to article and see the complete guide.
Assuming laravel 8 already installed at system. Next we are moving forward to routes tutorial.
What is Routing in Laravel?
Routing simply means mapping of application URLs to application view files. They usually handle HTTP requests and serve response. Inside this article we will see about followings –
- Basic Route Configuration
- Route with View file
- Route with Controller Method
- Sending Parameters to Route
- Routes with Regular Expression
- Redirect Routes
- Route Methods
So, step by step we will proceed and understand about each Laravel 8 routing.
Basic Route Configuration
As we have already discussed that all routes configuration of web application we do in web.php file. The file web.php you will find inside /routes folder
Let’s create a very basic route inside this –
//... Route::get('about-us', function () { return 'Very basic route in application - Online Web Tutor Blog'; }); //...
How can we access?
As we know we have two options available to access application URL into browser. These are following ways.
- php artisan serve
- By application URL
Assuming application has served by php artisan serve command. So to access the above route what we have defined above will be –
http://localhost:8000/about-us
This will simply print the static message what we have written inside callback function of closure route.
Route with View file
We can call view file directly from web.php. No need to create any controller.
Method #1
//... Route::get('our-products', function () { return view("products"); // view file in /resources/views/products.blade.php }); //...
Method #2
This is also termed as view routes in laravel.
//... // Route::view('route-name', 'view-file') Route::view('our-products', 'products'); // view file in /resources/views/products.blade.php //...
So to access the above route what we have defined above will be –
http://localhost:8000/our-products
This will return products.blade.php view file content.
Route with Controller Method
In Laravel 8 routing, this is the most commonly used approach. Means we will have a route and routes map with the controller’s method and method points to a view file.
Open web.php file –
# Add these to headers use Illuminate\Support\Facades\Route; use App\Http\Controllers\SiteController; //Route::get("/route-name", [SiteController::class, "method_name"]); Route::get("services", [SiteController::class, "services"]);
Next, we need to create controller. Controllers will be stored in the directory of /app/Http/Controllers. We create controllers in two different ways.
- By PHP artisan command
- Manual method of creating controller
We will create controller by artisan command.
$ php artisan make:controller SiteController
<?php namespace App\Http\Controllers; class SiteController extends Controller { public function services() { return view("services"); } }
So to access the above route what we have defined above will be –
http://localhost:8000/services
This will return services.blade.php view file content.
Sending Parameters to Route
This route will send some parameter values to it’s associated method. Open up web.php file –
# Add these to headers use Illuminate\Support\Facades\Route; use App\Http\Controllers\SiteController; // Passing parameter to URL Route::get("services/{service_id}", [SiteController::class, "services"]); // Passing parameter to URL Route::get('product/{id}', function ($id) { return 'Product ID '.$id; }); // Passing values to view routes Route::view('/about-us', 'about-us', ['name' => 'Sanjay Kumar']);
So to access the above route what we have defined above will be –
http://localhost:8000/services/101
This will pass 101 value to controller method services. Back to controller and access this value.
<?php namespace App\Http\Controllers; class SiteController extends Controller { public function services($service_id) { //return "This is service we are using as an ID of ".$service_id; return view("services", ["id" => $service_id]); } }
One more thing, this parameter is required value for the route which we pass into the URL. If we don’t pass then it will give error about missing parameters. In some cases we need to pass parameters but as a optional value. Means we want to create optional parameters. So for that,
//... Route::get('product/{id?}', function ($id = null) { return "Product: ". $id; }); //...
In this case, we can either pass value or need not to pass value. It is optional now.
Routes with Regular Expression
In routes configuration, regular express set the value patter for parameters. From above discussion, we understood about passing parameter values to routes.
Now, in case if we set the placeholder to accept some pattern values like –
Route::get('product/{name}', function ($name) { return "Product Name: ". $name; })->where('name', '[A-Za-z]+');
Here, {name} is required parameter. and by using where to placeholder “name” as you can see we have set a pattern for it. Inside {name} we can pass the values either in between of A-Z or a-z. Means string value will be accepted only. If we send any integer, it will give error.
To pass integer value –
Route::get('student/{id}', function ($id) { return "Student ID: ". $id; })->where('id', '[0-9]+');
Two parameters passing in route.
Route::get('student/{id}/{name}', function ($id, $name) { return "Student name: ".$name." and ID : ".$id; })->where(['id' => '[0-9]+', 'name' => '[a-z]+']);
Here, we have configured some routes in web.php
<?php use Illuminate\Support\Facades\Route; Route::get('/', function () { return view('welcome'); }); Route::get('about-us', function () { return 'Very basic route in application - Online Web Tutor Blog'; }); Route::get('our-products', function () { return view("products"); // view file in /resources/views/products.blade.php }); Route::get('product/{id}', function ($id) { return 'Product ID '.$id; });
To check all available routes in laravel application, we have a artisan command to list all. Artisan command to check all routes –
$ php artisan route:list
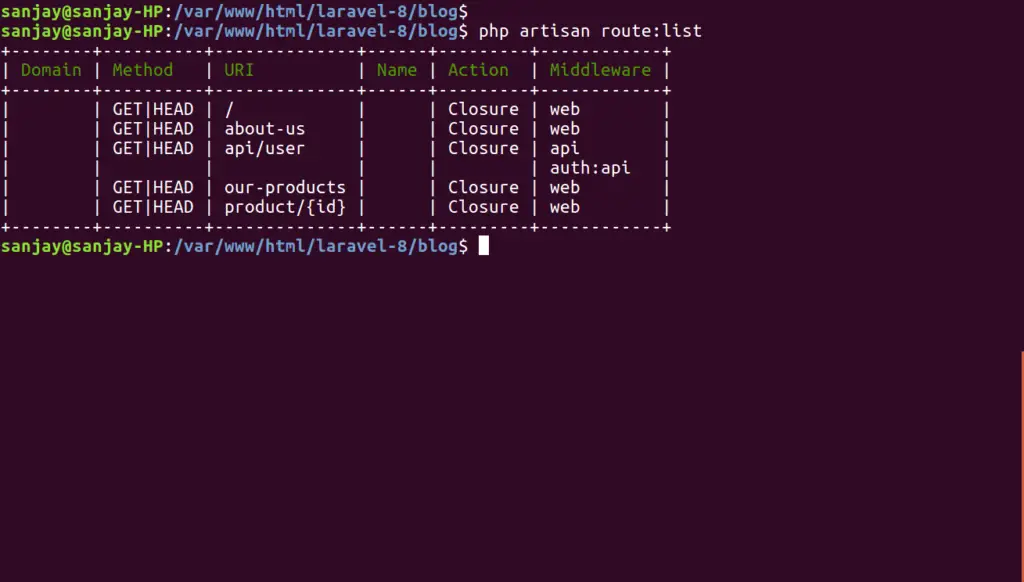
Redirect Routes
If we want redirect some URLs and/or routes to some different URL, we can use the concept of redirect routes. By default Route::redirect returns 302 status code. But if we want change we can pass into it’s syntax.
// When we open http://localhost:8000/source-url then it will be redirected to // http://localhost:8000/desitnation-url Route::redirect('/source-url', '/desitnation-url'); //Route::redirect('/source-url', '/desitnation-url', 301);
Route Methods
The router in Laravel 8 allows us to register routes that respond to any HTTP request as –
Route::get($uri, $callback); Route::post($uri, $callback); Route::put($uri, $callback); Route::patch($uri, $callback); Route::delete($uri, $callback); Route::options($uri, $callback);
According to the request type, we can move further with the Route method. To learn more about routing in Laravel 8, you can see the available document here at official website.
We hope this article helped you to learn Laravel 8 Routing in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.
I like this web blog very much so much excellent info.